How to Remove File Extension Using Bash
-
Use the
cut
Method to Remove the File Extension in Bash - Issues When Removing File Extension in Bash
-
Remove File Extension Using the
basename
Command in Bash
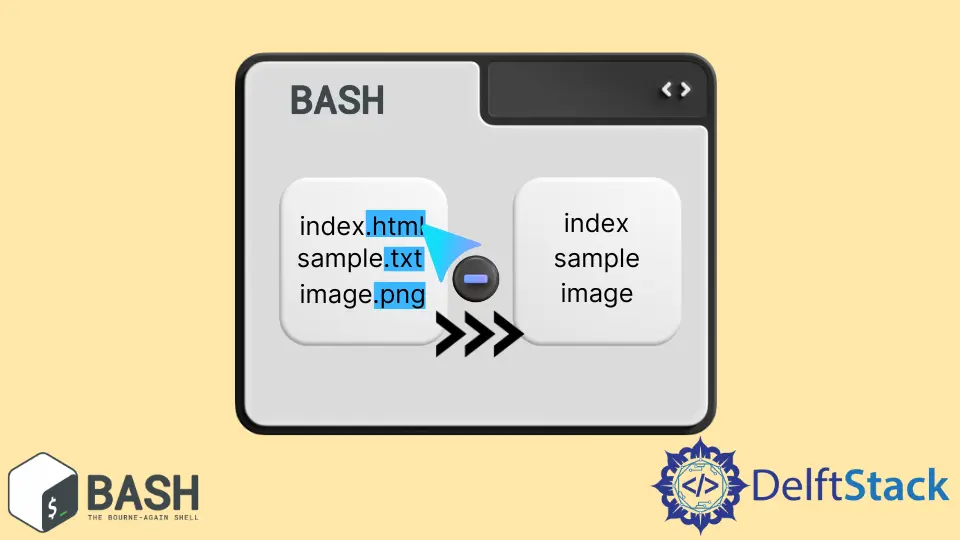
This tutorial discusses the method to cut and display specific fields of a file using the bash script through the cut
command and basename
bash commands. We will also see its use in conjunction with pipes. Then, we will see some issues that may potentially arise when cutting extensions from the filenames.
We will also look at multiple illustrative examples of trimming the file extensions from a filename and displaying just the stemmed version of that filename.
Use the cut
Method to Remove the File Extension in Bash
The cut
method s used to trim certain sections of a file from each line. This command takes a filename and an option (mandatory) as arguments and outputs the results on the standard console. We can use it to remove filename extensions as well. For this sake, the -f
option is used along with the filename. A brief description of some of the available options is as follows.
-b
(Bytes): This option trims portions of text from all the lines after some specified number of bytes.-c
(Column): Used to cut specific columns or range of columns from the file and display the remainder on consol.-f
(Field): A collection of symbols between two delimiting symbols (except for the first) is said to be a field. For each line in the file,-f
only displays fields or portions of the line mentioned using integral field numbers. Thetab
is considered a delimiter by default. However, we can use the-d
flag to define any user-defined delimiting symbol. Synopsis for thecut
command with-f
andd
options is as below.
$ cut -f (comma separated field numbers) -d "delimiting symbol" filename.txt
There are other options available as well like -complement
, -output-delimiter
, and -version
. You may take help using cut --help
to get more information.
Example 1: Removing Extensions From Content
Consider the following example to learn more about -f
and -d
. Assume webpages.txt contains names of different HTML webpages for a particular website, each on a new line. Let’s look at the contents of webpages.txt by executing the cat
command on the terminal: -
$ cat webpages.txt
index.html
contact_us.html
login.html
dashboard.html
Following bash script will cut extensions from all the filenames within the webpages.txt and will show output to the standard console. Assume we have created a shell script file named as script and wrote the following code in it.
#!/bin/sh
#script file
file=webpages.txt
cut -f 1 -d '.' $file
Here 1
in front of -f
is used to keep only the first field, and .
in -d
options indicates that fields shall be separated using the .
symbol. Therefore, the script keeps all the text in each line of webpages.txt before the first .
encounter. Let’s have a look at the output.
Output:
index
contact_us
login
dashboard
Here, If we set the field number to 2
, only a second field (i.e., HTML) shall be printed on the screen for each line.
Example 2: Removing Extension From a Filename Stored in a Variable Using Pipe and Displaying It on the Console
Assume that you want to remove the extension from a file name stored in a variable. The following piece of code will do that.
file=webpages.txt
echo "$file" | cut -f 1 -d '.'
Output:
webpages
The above code pipes the filename stored in the file
variable to the cut command, which retains only the first field of the text.
Example 3: Removing Extension From a Filename Stored in a Variable Using Pipe and Assigning It to Another Variable
file=webpages.txt
trimmed=$(echo "$filename" | cut -f 1 -d '.')
echo "$trimmed"
Output
webpages
The above code uses the command substitution method for substituting the output of the command at the place of command. Therefore, trimmed
is assigned whatever the right side of the assignment outputs.
Issues When Removing File Extension in Bash
When a filename contains the delimiting character itself in the filename, then the above formulation will only return text before the first encounter of the delimiting character. We can solve this issue by reversely cutting the name.
Let’s have a look at the following code.
file=abcd.efg.hmtl
trimmed=$(echo "$filename" | cut -f 2- -d '.')
echo "$trimmed"
Output:
abcd
In the above piece of code, the file
variable has one more dot in the assigned value. The output will contain all the text in the first field, which ends at the encounter of the first dot.
Solution: We can reverse the contents of file
before piping it to the cut
command. After that, the cut
command will retain all the fields except for the first from the reversed text. Now, it needs to be reversed again to get the final answer.
file=abcd.efg.html
trimmed=$(echo "$file" | rev | cut -f 2- -d '.' | rev)
echo "$trimmed"
Output:
abcd.efg.html
Please note the change in the f
options’ value. The option -f 2-
is used to retain all the fields with a position value two or greater.
Remove File Extension Using the basename
Command in Bash
If you know the name of the extension, then you can use the basename
command to remove the extension from the filename.
file=abcd.abc.html
trimmed=$(basename $file .html)
echo "$trimmed"
Output
abcd.abc
The first command-Line argument of the basename
command is the variable’s name, and the extension name is the second argument. The basename
command scans through the text and prints everything before the first encounter of the extension name (.html
in our case).
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn