How to Read From a File or User Input in Bash
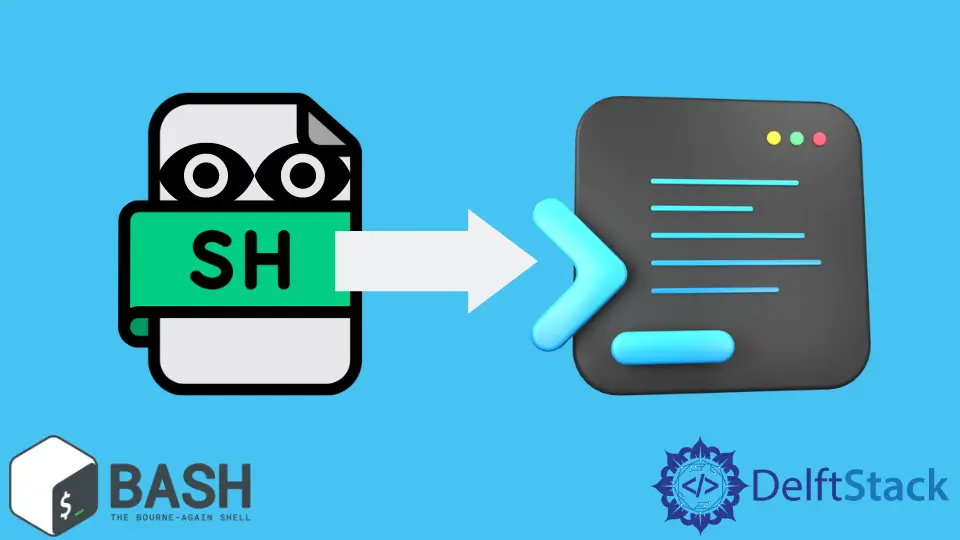
BASH is the shorthand for Bourne Again Shell, a smart name that refers to Bourne Shell (i.e., created by Steven Bourne). BASH is a shell program developed by Brian Fox as an updated version of the Bourne Shell application sh
.
This article will discuss the input feature in bash scripts and the methods to get input from the standard input console and some specific files. Furthermore, we will also go through multiple example scripts to illustrate the idea.
The BASH Script
A plain text file containing a list of instructions we would normally put in the command line is called Bash Shell Script. A Bash is particularly useful when a sequence of tasks is to be performed repetitively.
Instead of typing the same sequence of instructions manual each time, just a pre-written Bash script file can be executed. Therefore, this saves lots of manual work and also saves time.
One more advantage of the Bash script is its imperative programming features. This means it allows writing procedures, conditional statements, and looping structures like the programming languages (e.g., C/C++, Javascript, etc.).
Bash Script is a text file with a .sh
extension. To create it, we use the touch
command with the extension .sh
.
touch myscript.sh
We can open it in any text editor, for example, gedit
. To open it, use the following command.
Command:
gedit myscipt.sh
We use the line #!/bin/bash
to start a shell script. In this line #!
part is known as the shebang
, and the remaining part is the path to the bash interpreter in the operating system.
After that, we will write our required set of commands in the script and save it. To run a Bash script, we use the command bash
.
Command:
bash myscript.sh
This will run our script and give us the output of the commands used in the script.
Input From stdin
in Bash Script
We can also take user input from the terminal or stdin
in our Bash script. We can use the built-in Bash command read
to read the Bash user input.
It accepts the user’s input and assigns it to a variable. It can read only a single line from the Bash shell.
Syntax:
read <variable>
Each word separated by space is saved in a different variable.
Bash Script Example:
#!/bin/bash
echo "Enter your name: "
read userName
echo "Enter your age: "
read userAge;
echo "Enter your 3 Subjects: "
read sub1 sub2 sub3
echo "The user $userName is $userAge years old and is specialized in $sub1, $sub2, and $sub3. "
In this example, we have taken input from the user of his name, age and 3 subjects. Subjects are saved in separate variables.
Output:
Giving the variable name with the read
command is not compulsory. If we don’t specify the variable name, it stores the value in the REPLY
variable by default, so we can use it later in our script.
Bash Script Example:
#!/bin/bash
echo "Enter a number from 1-10: "
read
echo "You entered: $REPLY"
Output:
In the previous examples, we have used 2 lines to take input, one for the prompt statement and the other for the read command. This process can also be performed in a single line using a flag -p
with the read
command.
Syntax:
read -p <Prompt statement> <variable_name>
Bach Script Example:
#!/bin/bash
read -p "Please enter the name of the file: " fileName
echo "The filename you entered is: $fileName"
Output:
There are some cases when we need to hide the user input from the screen for security and privacy concerns. For example, when we need to take a password as input, it should not be visible on the screen while the user is entering it.
To achieve it, we use the flag -s
to make it silent.
Bash Script Example:
#!/bin/bash
read -p "Enter username: " userName
read -sp "Enter Password: " pass
echo
echo "Hello $userName"
Output:
We can also take input in an array using the -a
flag. With this flag, all the words in a line will be stored in the different indexes of the array that can be accessed later.
Bash Script Example:
#!/bin/bash
echo "Enter names of 2 students: "
read -a stuNames
echo "The names of students are:${stuNames[0]}, ${stuNames[1]} "
Output:
Bash File Input
Bash script can also take input from files. read
command is used for this purpose and reads the file line by line. We will discuss some examples for reading data from the file.
We can read from files using the console as well as the script. First, we look at the console command and then move toward the script.
Suppose we have a file data.txt
with some text in it like this:
LG
Samsung
Bosch
Pel
The command to read this file:
while read ln; do echo $ln; done < data.txt
Output:
We can also use a script to read from the file.
Bash Script Example:
#!/bin/bash
fname='data.txt'
a=1
while read ln; do
echo "Reading line $a : $ln"
a=$((a+1))
done < $fname
Output:
We can also read the file name from the command line. Create a bash file and put the script below into it.
The filename will be taken from the command line input by this script. The variable $1
, which will hold the filename for reading, reads the first argument value.
If the file exists at the current location, the while loop will read the file line by line and print the contents, just like in the previous example.
Bash Script Example:
#!/bin/bash
fname=$1
while read ln; do
echo $ln
done < $fname
To run this script, we will use the following command.
Command:
$ bash myscript.sh data.txt
Output:
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn