How to Check if Input Argument Exists in Bash
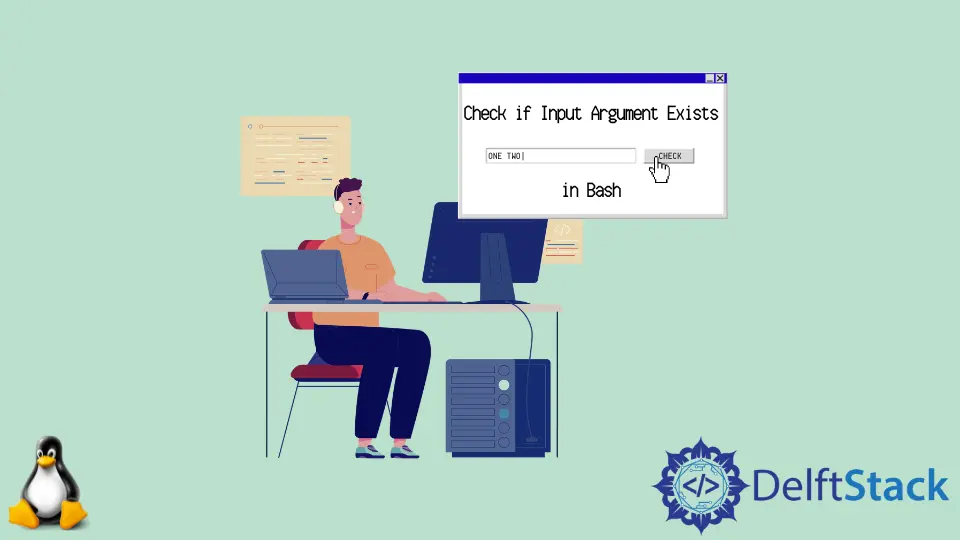
When we create Bash scripts, we might want to take arguments to use within our scripts to run successfully. Therefore, we need to create a script to check the number of input arguments that a user uses with the script.
All these prevent unexpected behaviors when a user doesn’t pass the required arguments when using the script or command, and then we can pass an error message telling the user that they have not used the required number of arguments.
This article will show you how to check whether an input argument exists or the number of existing arguments.
Use $#
to Check if Input Argument Exists in Bash
Within Bash, a special variable, $#
, holds the input argument. With $#
, you can check how many input arguments have been passed to your Bash script.
A simple Bash script will show you what this $#
variable holds when passed with no arguments or when passed with two arguments.
#!/bin/bash
echo "The number of input arguments passed to this script: "
echo $#
Let’s run the script with no input arguments:
$ ./script.sh
The terminal output is shown below:
The number of input arguments passed to this script:
0
Now, let’s pass two arguments to the same script:
$ ./script.sh one two
The output of the script becomes the below:
The number of input arguments passed to this script:
2
Now, we can use $#
and a conditional statement that checks whether $#
equals zero (meaning no input arguments) within our script to exit when true
. If $#
is greater than 0, the condition becomes false
, and the else
part of the conditional statement gets executed.
#!/bin/bash
if [ $# -eq 0 ]
then
echo "No input arguments exist"
exit 1
else
echo "The number of input arguments passed:"
echo $#
fi
Let’s run the script using the command below with no argument:
$ ./script.sh
The output of the code:
No input arguments exist
Now, let’s run a different script command with arguments:
$ ./script.sh one two
The output of the code is different because the condition check equates to false
:
The number of input arguments passed:
2
Aside from that, we can use another special variable that uses the $[number]
to access the input argument. These are positional parameters that we can use within Bash.
If we know that we will get three variables or have ascertained that, we can access the three variables using the code below.
#!/bin/bash
echo "The input arguments are:"
echo $1 $2 $3
The output of the code, when passed with three arguments, will be the below:
The input arguments are:
one two three
Use $1
to Check if Input Argument Exists in Bash
Remember the positional parameters we discussed in the previous section. We can use the first one, $1
, to check if any input argument has been passed since if there is no input argument, there can’t be any value within the positional parameter, $1
.
Therefore, we can use the if-else
statement where the condition expression checks if there is a value within the positional parameter, $1
. However, if there is a value, it echoes the number of input arguments and the first argument using the positional parameters.
#!/bin/bash
if [ -z "$1" ]
then
echo "Please, pass an argument"
exit 1
else
echo "The number of input arguments are"
echo $#
echo "The first one is"
echo $1
fi
Let’s run the code with no argument:
$ ./script.sh
The output of the script:
Please, pass an argument
Now, let’s run it with some arguments:
$ ./script.sh delft stack blog
The output of the code:
The number of input arguments are
3
The first one is
delft
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn