How to User Input in Bash
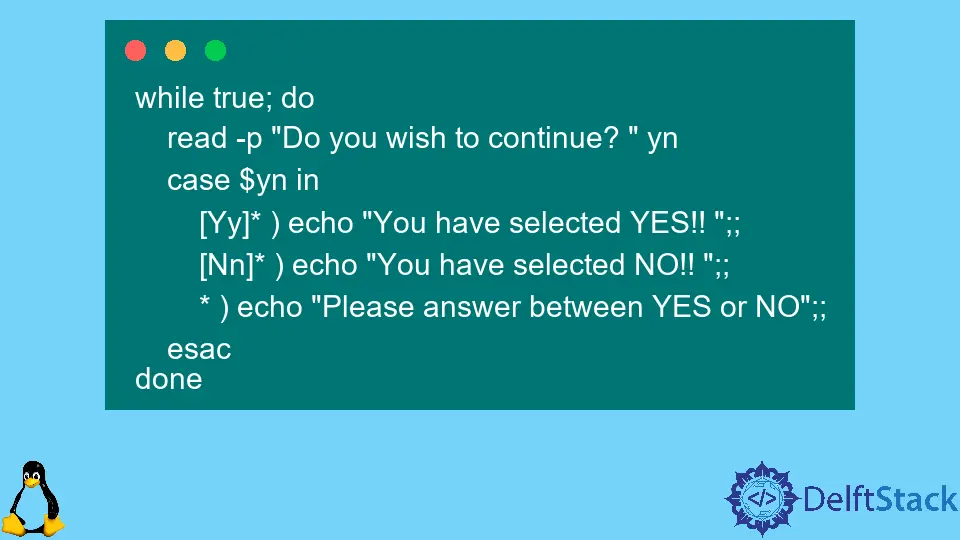
Taking user input is very important for any program or script. In this way, a user interacts with the system and provides input to the system.
Like other programming or scripting language, Bash supports taking user input. The general format to take user input is read YOUR_VARIABLE
.
If you want to create a tag for users to let them know what kind of input they need, the format will look like read -p "YOUR INSTRUCTION HERE : " YOUR_VARIABLE
. In this article, we’ll see how we can take user input to the system in Bash.
Also, we will see some examples and explanations relevant to the topic to make the topic easier.
User Input in Bash
As we already discussed, we need to use the keyword read
to take user input to the system. It’s a built-in keyword in Bash that reads the user input.
In the example below, we will take a user input and provide the user with the output, including the user input. The code for our example will look like this:
read YOUR_VAR
echo "You entered the number $YOUR_VAR"
Above shared a very simple example through which we take an input and provide an output like below.
50
You entered the number 50
But how will the user know he must provide a numeric value here? We need to instruct the user that he needs to provide a numeric value here.
To do this, you need to follow the below example, which is similar but advanced from the previous one. The code for our example will look like the below.
read -p "Please enter a number: " YOUR_VAR
echo "You entered the number $YOUR_VAR"
When you run this example, the script first shows a message Please enter a number:
and when the user provides a numeric value, he will get the below output.
Please enter a number: 50
You entered the number 50
Taking YES
or NO
Input From the User
Below we will see an example that will take only the user input between YES
or NO
. The code for our example will be something like this:
while true; do
read -p "Do you wish to continue? " yn
case $yn in
[Yy]* ) echo "You have selected YES!! ";;
[Nn]* ) echo "You have selected NO!! ";;
* ) echo "Please answer between YES or NO";;
esac
done
We used a while
loop and a conditional statement case
in our example. We take user input through the line read -p "Do you wish to continue the program? " yn
.
Through the line [Yy]* ) echo "You have selected YES";;
and [Nn]* ) echo "You have selected NO";;
we check the user input.
If the input is Y
or y
, it will show the output You have selected YES
, and if the input is N
or n
, it will show the output You have selected NO
.
Lastly, we set a default output if a user puts any unexpected input by the line * ) echo "Please answer YES or NO";;
. After running the code, we will get an output like this:
Do you wish to continue the program? Y
You have selected YES!!
Do you wish to continue the program? Y
You have selected YES!!
Do you wish to continue the program? N
You have selected NO!!
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn