How to Take Optional Input Arguments in Bash
- Understanding Optional Input Arguments
- Creating a Basic Bash Script
- Adding More Complexity: Handling Multiple Optional Arguments
- Validating Input Arguments
- Conclusion
- FAQ
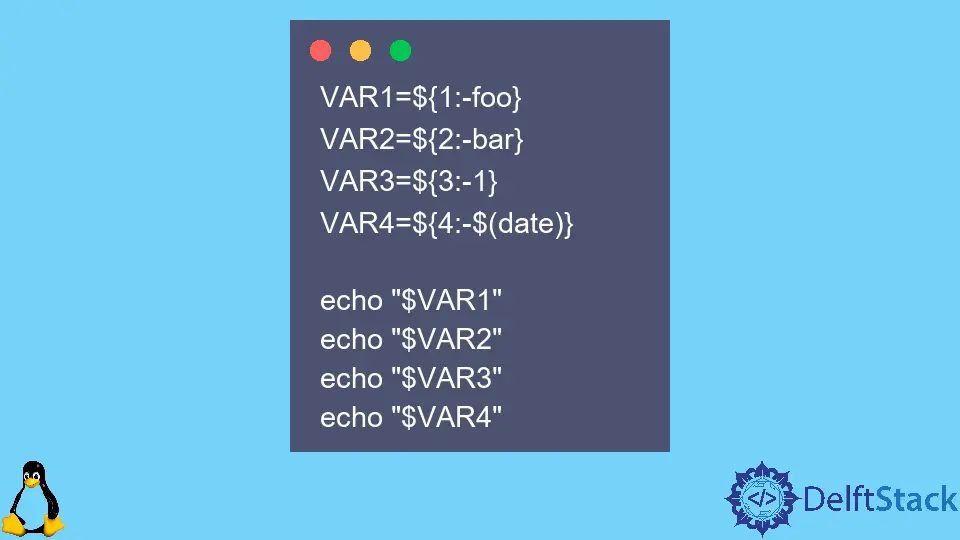
When writing Bash scripts, one of the most powerful features you can leverage is the ability to accept optional input arguments. This capability allows your scripts to be more flexible and user-friendly, enabling users to customize their behavior without modifying the script itself.
In this tutorial, we will explore how to create a Bash script that can handle optional input arguments effectively. By the end of this article, you will have a solid understanding of how to implement this feature, making your scripts more dynamic and adaptable to various situations.
Understanding Optional Input Arguments
Before diving into the code, let’s clarify what optional input arguments are. In Bash, input arguments are values passed to a script when it is executed. By making some of these arguments optional, you can allow users to run the script with fewer inputs while still providing default values or alternative behaviors.
For instance, imagine a script designed to clone a Git repository. You might want to allow users to specify the repository URL and an optional branch name. If they don’t provide a branch name, the script can default to the main branch. This flexibility is what makes optional input arguments so valuable.
Creating a Basic Bash Script
Let’s start by creating a simple Bash script that accepts optional input arguments. The following script will clone a Git repository and optionally allow the user to specify a branch name.
#!/bin/bash
REPO_URL=$1
BRANCH_NAME=${2:-main}
if [ -z "$REPO_URL" ]; then
echo "Usage: $0 <repository-url> [branch-name]"
exit 1
fi
git clone -b "$BRANCH_NAME" "$REPO_URL"
Output:
Cloning into 'your-repo'...
In this script, the first line specifies the interpreter to use (Bash). The second line captures the first input argument as REPO_URL
, while the third line uses parameter expansion to set BRANCH_NAME
to the second argument if provided; otherwise, it defaults to main
.
The script checks if the REPO_URL
is empty, prompting the user for the correct usage if so. Finally, it executes the git clone
command with the specified branch. This simple structure allows for optional input while ensuring that the script remains functional.
Adding More Complexity: Handling Multiple Optional Arguments
Now, let’s enhance the script to handle multiple optional arguments. We can add the ability to specify a directory where the repository should be cloned. This way, users can choose where to place their cloned repository.
#!/bin/bash
REPO_URL=$1
BRANCH_NAME=${2:-main}
TARGET_DIR=${3:-.}
if [ -z "$REPO_URL" ]; then
echo "Usage: $0 <repository-url> [branch-name] [target-directory]"
exit 1
fi
git clone -b "$BRANCH_NAME" "$REPO_URL" "$TARGET_DIR"
Output:
Cloning into 'your-repo'...
In this version of the script, we introduced TARGET_DIR
, which allows users to specify where the repository should be cloned. If the user doesn’t provide a target directory, it defaults to the current directory (denoted by .
). This makes the script even more versatile, as it caters to different user needs.
The script still checks for the presence of the REPO_URL
, ensuring that the user provides the necessary input. With this structure, users can now run the script in various ways, enhancing its usability.
Validating Input Arguments
Input validation is crucial for ensuring that your script behaves as expected. Let’s add some validation to check if the provided repository URL is valid and if the branch exists within that repository.
#!/bin/bash
REPO_URL=$1
BRANCH_NAME=${2:-main}
TARGET_DIR=${3:-.}
if [ -z "$REPO_URL" ]; then
echo "Usage: $0 <repository-url> [branch-name] [target-directory]"
exit 1
fi
if ! git ls-remote --exit-code "$REPO_URL" "$BRANCH_NAME" > /dev/null; then
echo "Branch '$BRANCH_NAME' does not exist in the repository '$REPO_URL'."
exit 1
fi
git clone -b "$BRANCH_NAME" "$REPO_URL" "$TARGET_DIR"
Output:
Cloning into 'your-repo'...
In this enhanced script, we use the git ls-remote
command to check if the specified branch exists in the provided repository. If the branch does not exist, the script exits with an error message. This level of validation makes your script more robust and user-friendly, preventing potential errors during execution.
Conclusion
In this tutorial, we explored how to take optional input arguments in Bash scripts, specifically in the context of Git operations. By allowing users to specify optional parameters, such as branch names and target directories, we enhanced the flexibility and usability of our scripts. We also covered essential input validation techniques to ensure that the provided arguments are valid, leading to more reliable script execution. With these skills, you can create more dynamic and user-friendly Bash scripts that cater to a variety of needs.
FAQ
-
What are optional input arguments in Bash?
Optional input arguments are parameters that a user can provide when executing a script, but they are not required for the script to run. -
How do I set default values for optional arguments in Bash?
You can use parameter expansion to set default values, like this:VAR=${1:-default_value}
. -
Can I validate input arguments in a Bash script?
Yes, you can validate input arguments using conditional statements and commands likegit ls-remote
. -
What happens if I don’t provide required arguments in a Bash script?
If a required argument is not provided, you can display a usage message and exit the script to prevent errors. -
How can I make my Bash scripts more user-friendly?
You can improve user-friendliness by providing clear usage instructions, validating input, and allowing optional arguments with default values.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn