How to Loop Over Files in Directory in Bash
- Method 1: Using a Basic For Loop
-
Method 2: Using a
while
Loop with Find Command - Method 3: Using the Globstar Option for Recursive Looping
- Conclusion
- FAQ
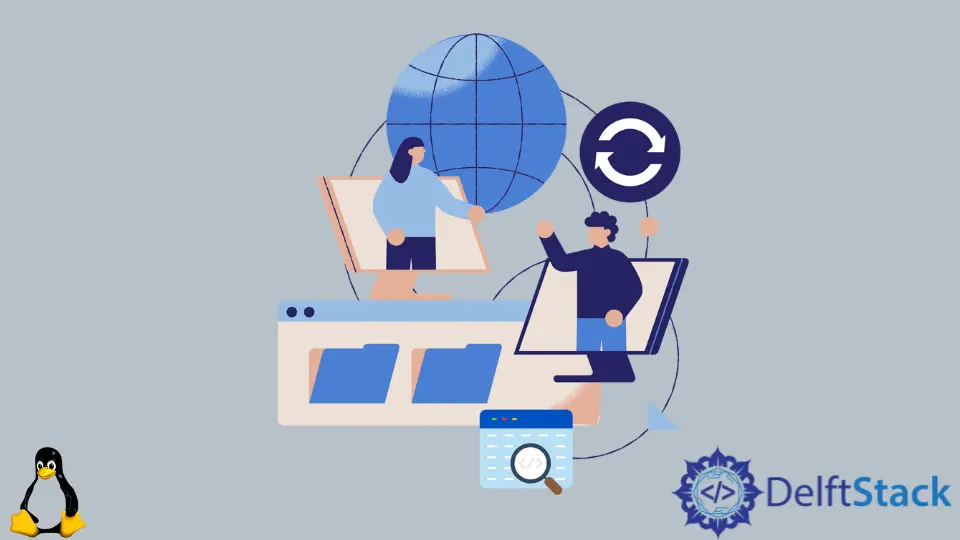
Looping over files in a directory using Bash is a common task that can simplify various automation processes. Whether you are managing files, performing batch operations, or just organizing your directory, understanding how to loop through files can save you time and effort.
In this tutorial, we will explore several methods to loop over files in a directory using Bash commands. This guide is designed for beginners and experienced users alike, providing step-by-step instructions and practical examples. By the end of this article, you will confidently loop through files in your directories, enhancing your scripting skills and efficiency.
Method 1: Using a Basic For Loop
One of the simplest ways to loop over files in a directory is by using a basic for
loop. This method iterates through each file in the specified directory and allows you to perform operations on them. Here’s how you can do it:
for file in /path/to/directory/*; do
echo "Processing $file"
done
In this example, replace /path/to/directory/
with the actual path of your directory. The *
wildcard matches all files in the specified directory. The loop goes through each file, and the echo
command outputs the name of the file being processed. You can replace the echo
command with any other command you want to execute on each file, such as copying, moving, or modifying files.
Output:
Processing /path/to/directory/file1.txt
Processing /path/to/directory/file2.txt
Processing /path/to/directory/file3.txt
This method is straightforward and efficient for most use cases. However, it does not handle subdirectories or hidden files (those starting with a dot). If you need to include those, you might want to consider other methods.
Method 2: Using a while
Loop with Find Command
If you want more control over the files you are processing, using the find
command in combination with a while
loop is a powerful approach. This method allows you to find files based on specific criteria, such as file type or modification date. Here’s how to use it:
find /path/to/directory/ -type f | while read file; do
echo "Processing $file"
done
In this example, find
searches for files (-type f
) in the specified directory and outputs their paths. The while read file
construct reads each line of output from find
, allowing you to process each file. The echo
command outputs the name of each file being processed.
Output:
Processing /path/to/directory/file1.txt
Processing /path/to/directory/file2.txt
Processing /path/to/directory/file3.txt
This method is particularly useful when dealing with large directories or when you want to apply filters to the files you are processing. The find
command is highly versatile and can be customized to fit various needs, making it a preferred choice for more complex file operations.
Method 3: Using the Globstar Option for Recursive Looping
If you need to loop over files in a directory and its subdirectories, you can enable the globstar
option in Bash. This allows you to use the **
wildcard to match files recursively. Here’s how to do it:
shopt -s globstar
for file in /path/to/directory/**/*; do
echo "Processing $file"
done
In this example, shopt -s globstar
enables the recursive globbing feature. The **
wildcard matches all files and directories at any level within the specified path. The loop processes each file found, and you can replace the echo
command with any operation you wish to perform.
Output:
Processing /path/to/directory/file1.txt
Processing /path/to/directory/subdirectory/file2.txt
Processing /path/to/directory/subdirectory/file3.txt
This method is extremely useful for projects that involve nested directories, as it allows you to handle files at all levels without needing to write complex scripts. However, be cautious when using this method in directories with a large number of files, as it may result in performance issues.
Conclusion
Looping over files in a directory using Bash is a fundamental skill that can greatly enhance your productivity and scripting capabilities. Whether you choose a basic for loop, a while
loop with the find command, or the globstar option for recursive processing, each method has its unique advantages. By mastering these techniques, you can automate tasks, manage files more effectively, and streamline your workflow. As you practice these commands, you’ll find that they become invaluable tools in your Bash scripting toolkit.
FAQ
-
How do I loop through only text files in a directory?
You can modify thefor
loop to target specific file types, like this:for file in /path/to/directory/*.txt; do ...
-
Can I loop over files in multiple directories at once?
Yes, you can usefind
with multiple paths:find /path/to/dir1 /path/to/dir2 -type f | while read file; do ...
-
What if I want to ignore hidden files
while
looping?
You can use a pattern that excludes hidden files:for file in /path/to/directory/[!.]*; do ...
-
Is there a way to execute a command on each file instead of just printing its name?
Absolutely! Replace theecho
command with any command you want to execute, likemv
,cp
, orrm
. -
Can I use these methods in a script?
Yes, you can include any of these looping methods in your Bash scripts to automate file processing tasks.