For Loop in Bash
-
the
for
Loop in Bash -
Examples Using the
for
Loop in Bash -
Conditional Exit With a
break
in thefor
Loop in Bash -
Command Substitution With
for
Loop
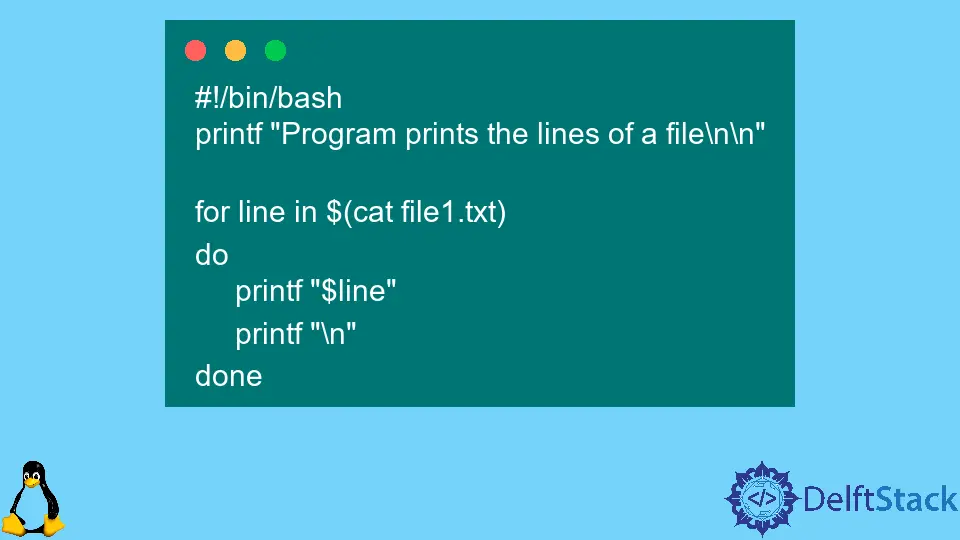
This tutorial explains using the for
loop in Bash scripts using the range notation and the three-expression notation like in the C programming language.
the for
Loop in Bash
The for
loop is a Bash statement used to execute commands repeatedly. The for
loop in Bash uses two main notations. The two notations are shown below.
The first notation uses the for
loop with a defined range. In the syntax below, the endpoint of the range is n
. It means the for
loop will execute the commands inside it for n
times before it stops.
for variable in 1 2 3 ... n
do
command1
command2
done
The second notation uses the for
loop with a three-expression like in the C programming language. exp1
is the initialization, exp2
is the condition, and exp3
is the increment.
for ((exp1; exp2; exp3))
do
command1
command2
done
Examples Using the for
Loop in Bash
The scripts below demonstrate using the for loop in Bash scripts.
This script sets the value of the variable i
to 1
the first time, and it prints line number 1
. Then it goes back to the for
loop, sets variable i
to 2
, and prints line number 2
.
The script repeatedly does this, changing the value of i
until it hits the last number, 5. Once it prints line number 5
, the for
loop automatically exits.
#!/bin/bash
for i in 1 2 3 4 5
do
printf "line number $i"
printf "\n"
done
Running the script above prints the following output to the standard output.
line number 1
line number 2
line number 3
line number 4
line number 5
The script below uses the curly braces to define a range for the for
loop to iterate through. The numbers inside the curly braces are incremented sequentially.
The first time, the value of i
is set to 1
. The script then executes the two printf
commands inside the for
loop. The value of i
is incremented by 1, it becomes 2
, and the printf
commands inside the for loop are executed.
The value of i
is repeatedly incremented until it finally becomes 5
, and once the two printf
commands have been executed, the for
loop automatically terminates itself.
!/bin/bash
for i in {1..5}
do
printf "Hello World $i"
printf "\n"
done
The following is the output that is printed out after running the script.
Hello World 1
Hello World 2
Hello World 3
Hello World 4
Hello World 5
Below, the script uses the three-expression notation like in the C programming language. In the first expression, x
is initialized to 0
. The second expression has the conditional test that checks if x
is less than or equal to the value of the max
variable.
The value of the max
variable has been set to 5
. The last expression increments the value of x
by 1
.
The first time, the value of x
is 0
, and the condition returns true because 0
is less than or equal to 5
. The for
loop then prints number: 0
to the terminal, and the value of x
is incremented by 1
. x
now becomes 1
.
1
is also tested if it is less than or equal to 5
, it returns true, and the for loop prints number: 1
. This process repeats itself until the condition returns false and the for
loop exits.
#!/bin/bash
max=5
printf "Print Numbers from 0 to $max\n"
for ((x=0;x<=max;x++)); do
printf "number: $x\n"
done
Below is the output printed to the terminal after running the script.
Print Numbers from 0 to 5
number: 0
number: 1
number: 2
number: 3
number: 4
number: 5
Conditional Exit With a break
in the for
Loop in Bash
The script below demonstrates exiting from a for
loop with the break
keyword. The for
loop is set to iterate if the x
variable’s value is less than or equal to the max
variable’s value. However, there is a test
command inside the for
loop.
The test
command checks if the value of the x
variable is equal to 3
; if this test returns true, the script encounters the break
keyword, and the for
loop exits.
#!/bin/bash
max=5
printf "Print Numbers from 0 to $max\n"
printf "Program will exit when we hit 3\n"
for ((x=0;x<=max;x++)); do
printf "number: $x\n"
if [ $x -eq 3 ]
then
break
fi
done
The following is the output displayed to the standard terminal when the script is executed. The for
loop exists when the value of x
is equal to 3
because the test
command inside the for
loop evaluates the arithmetic expression when the value of the x
variable is set to 3
, and it returns true. Then the script encounters the break
keyword inside the if
statement and exits.
Print Numbers from 0 to 5
Program will exit when we hit 3
number: 0
number: 1
number: 2
number: 3
Command Substitution With for
Loop
The script below shows using the for
loop with command substitution. Command substitution is a Bash feature that allows us to run Linux commands and store the command’s output in a Bash variable. Once a command is executed using this feature, the command’s standard output replaces the command.
In the script, the cat
command is executed using command substitution. The output of cat file1.txt
replaces the command, and the for
loop iterates through the command’s output and prints it to the standard output using the printf
command.
#!/bin/bash
printf "Program prints the lines of a file\n\n"
for line in $(cat file1.txt)
do
printf "$line"
printf "\n"
done
The script produces the following output when executed. The file file1.txt
contains a list of three languages that are printed out to the standard terminal.
Program prints the lines of a file
French
English
Spanish