How to Break Out of a Loop in Bash
-
Break Out of the
while
Loop in Bash -
Break Out of the
for
Loop in Bash -
Break Out of the
until
Loop in Bash
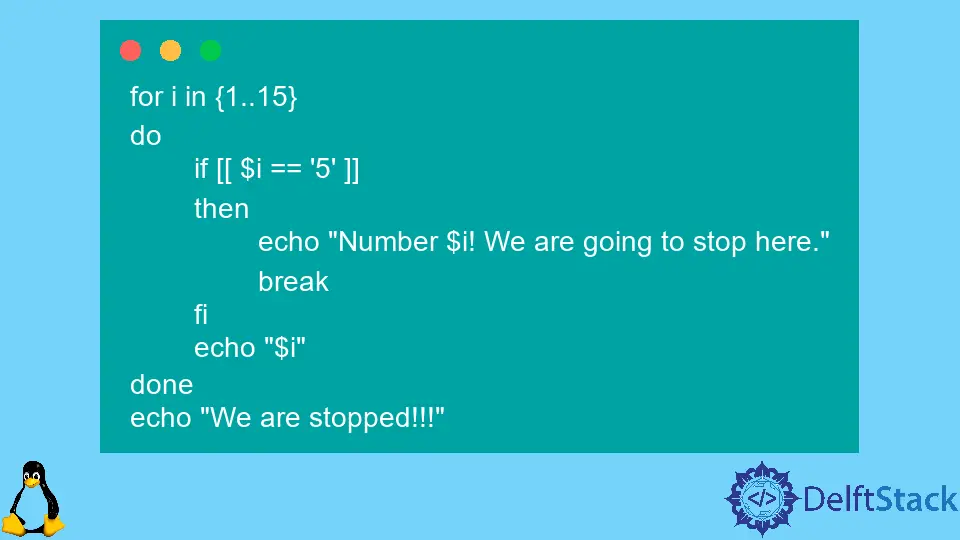
Working with the loop is a common task for any programming or scripting language. When working with the loop, sometimes we need to stop it under a pre-defined condition.
Like other programming and scripting languages, Bash uses the keyword break
to stop any loop.
This article will show how we can stop a loop from executing. Also, we will discuss the topic with necessary examples and explanations to make the topic easier to understand.
We will stop the three most used loops: while
, for
, and until
. Let us start one by one.
Break Out of the while
Loop in Bash
You can use the keyword break
with the while
loop. In this way, you can stop the execution of the while
loop in a specified condition.
Take a look at the below example:
i=0
while [[ $i -lt 15 ]]
do
if [[ "$i" == '4' ]]
then
echo "Number $i! We are going to stop here."
break
fi
echo $i
((i++))
done
echo "We are stopped!!!"
In the example shared above, we stopped the while
loop when the value of i
was equal to 4.
After executing the above Bash script, you will get an output like the one below:
0
1
2
3
Number 4! We are going to stop here.
We are stopped!!!
Break Out of the for
Loop in Bash
The keyword break
also can be used to stop the for
loop at a specific condition. To do this, see the below example:
for i in {1..15}
do
if [[ $i == '5' ]]
then
echo "Number $i! We are going to stop here."
break
fi
echo "$i"
done
echo "We are stopped!!!"
In the example shared above, we stopped the for
loop when the value of i
was equal to 5.
After executing the above Bash script, you will get the below output:
1
2
3
4
Number 5! We are going to stop here.
We are stopped!!!
Break Out of the until
Loop in Bash
There is another popular loop in Bash named until
, which can also be stopped by the keyword break
. To stop until
, you can follow the examples shared below:
i=0
until [[ $i -gt 15 ]]
do
if [[ $i -eq 5 ]]
then
echo "Number $i! We are going to stop here."
break
fi
echo $i
((i++))
done
echo "We are stopped!!!"
In the example shared above, we will stop the until
loop when the value of i
is equal to 5
.
After executing the above Bash script, you will get an output like the following:
0
1
2
3
4
Number 5! We are going to stop here.
We are stopped!!!
You can choose any of the methods above based on your loop.
All the codes used in this article are written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn