How to Implement the for Loop in Bash
-
Bash
for
Loop -
Bash C-Style
for
Loop Syntax -
Bash
Foreach
/For-in
Style Syntax -
Defining Ranges and Jump Size in Bash
for
Loop -
Making the
for
Loop in Bash Prompt and Addressing the Issue With Delimiting Semi-Colon;
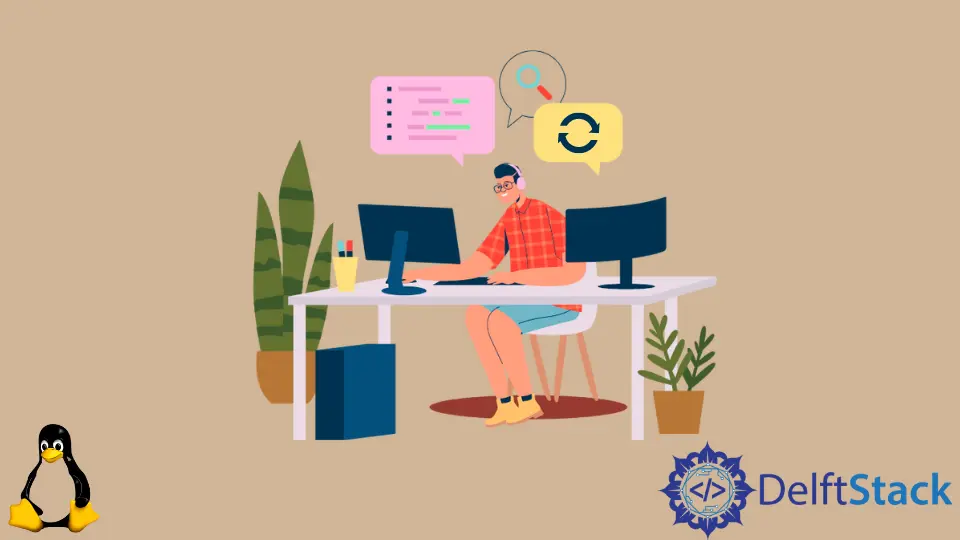
This tutorial will discuss the available Bash script formulations to write a Bash for
loop.
First, we will learn its basic syntax and concepts. Later, we will learn its different types in the Bash script, like the C-style for
loop notation and the foreach
or for-in
style.
In the end, we will conclude by discussing the issue of the semi-colon ;
while making the for
loop in the bash prompt.
Bash for
Loop
A loop in a programming or scripting language is an iterative control structure used to repetitively execute a statement or a set of statements until a certain criterion is not met.
The for
loop is the looping structure that is particularly used when a certain statement(s) or set of commands needs to be executed a specified number of times. We can also use the for
loop in the bash prompt and the Bash script.
The Bash script provides two syntaxes for writing the for
loops. The first one is known as C-style or three expression loop, and it is nearly the same as the C-language formulation for the for
loop.
The second formulation is a famous foreach
or for-in
style construct, also have been adopted by many popular programming languages like PHP, Python, C#, C++11, Ruby, and many others.
Bash C-Style for
Loop Syntax
This style uses three expressions like C-language to specify the number of loop iterations.
for (( initialization; condition; increment/decrement ))
do
Shell command[s]
done
Example: Assume we want to write a script that may help us print a table of any user-provided number. We can do that using the following code.
#!/bin/bash
echo "Enter a Number: "
read number
for ((j=1;j<=10;j++))
do
echo "$number X $j = $((number*j))"
done
The above code takes a number from the user and prints its table up to 10. Assume the user enters 5
as a number, the output of the program shall be as:
Enter a Number:
5
5 X 1 = 5
5 X 2 = 10
5 X 3 = 15
5 X 4 = 20
5 X 5 = 25
5 X 6 = 30
5 X 7 = 35
5 X 8 = 40
5 X 9 = 45
5 X 10 = 50
Bash Foreach
/For-in
Style Syntax
This type of for
loop expects a list of values/elements and performs a single iteration for each element in the list. The list can be provided by separating each item in a single space, or you may specify a range.
for Counter in 1 2 3 4 5 .. N
do
1st statement
2nd statement
nth statement
done
Example: Print Hello World
five times.
#!/bin/bash
for c in 1 2 4 5 6
do
echo "$c Hello World"
done
Output:
1 Hello World
2 Hello World
4 Hello World
5 Hello World
6 Hello World
Please note the missing 3
. This number is missed as the loop iterates only for the number of items given in the list. Therefore, the variable c
is assigned first a value 1
, and a single iteration is performed.
After that, 2
is assigned to c
, and the second iteration is performed. Later on, the immediate next value in the list (i.e., 4
) is assigned to the c
, and the process goes on until the whole list finishes.
for Item in File_0 File_1 File_N
do
Some Operations on Item
done
OR
for Item in $(Command)
do
Some Operations on Item
done
When a command is used with the for
loop, the Item
variable will be assigned with each of the output tokens one by one. For example, the following script will print all the files or folders starting with f
in the current directory using the for
loop.
#!/bin/bash
for Item in $(ls f*)
do
echo "$Item"
done
Defining Ranges and Jump Size in Bash for
Loop
If you know that there is no number missing between some start and end values. Even if the difference between the two consecutive values, also known as jump size, is greater than 1, you can use the for
loop with the following syntax.
for i in {Start_Value..End_Value..Jump_Size}
do
Statement(s)
done
Example: Printing all the even numbers between 10 and 20 will require the Start_Value=10
, the End_Value=20
, and the Jump_Size=2
.
#!/bin/bash
for evenNumber in {10..20..2}
do
echo "$evenNumber"
done
Output:
10
12
14
16
18
20
Making the for
Loop in Bash Prompt and Addressing the Issue With Delimiting Semi-Colon ;
The ;
is used to delimit or end commands on a single line. There are other delimiters as well, such as &
.
However, when the;
is used to terminate the command, the next command is only executed after the complete execution of the previous command, also known as synchronous execution.
Assume you want to write a for
loop on a single line in the bash prompt without using the newline
as a statement terminator, then you can use the following syntax taken from the Bash reference manual.
for Item [ [in [List of Items] ] ; ] do commands; done
Example: The following code is equivalent to our earlier script for printing even numbers.
#!/bin/bash
for even in {10..20..2} ; do echo "$even";done
do
keyword. Therefore, adding a ;
after the do
will generate a syntax error.The syntax for the three-expression or the C-type for
loop is the following.
for (( Initilization ; Condition ; Increment/Decrement )) ; do commands ; done
Example:
#!/bin/bash
for ((i=10;i<20;i=i+2));do echo "$i"; done
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn