How to Replace Characters in a String Using Bash
-
Method 1 - Use the
tr
Command - Method 2 - Use Bash Parameter Expansion
-
Method 3 - Use the
sed
Command
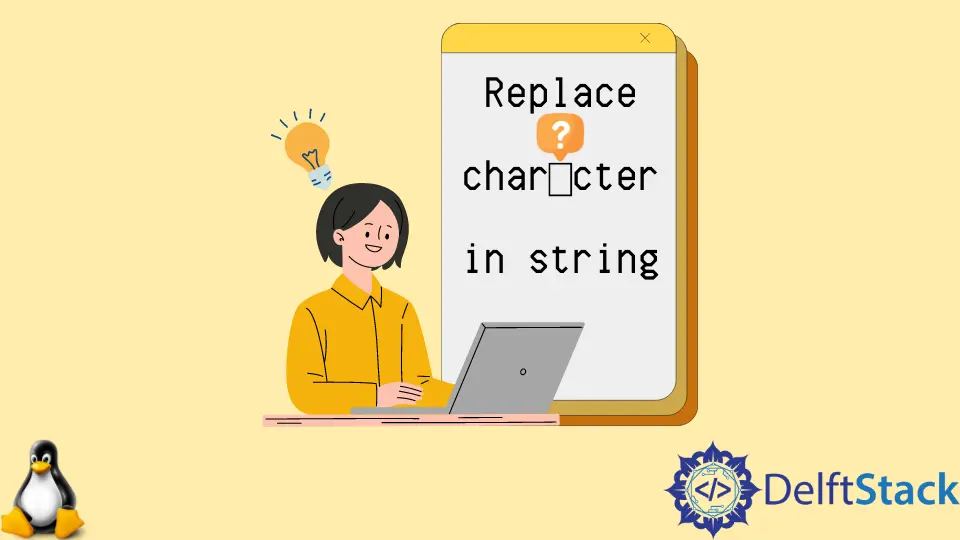
One common operation involving string
literals is replacing individual characters or substrings in that string with other characters or substrings.
In this article, we will look at several methods of string replacements using the BASH shell.
Method 1 - Use the tr
Command
The tr
is a very handy Bash command that can be used to perform different transformations on a string. It is particularly helpful in string transformations (e.g., changing the case of the string to upper or lower), removing substring repetitions, and searching and replacing substrings.
It is used to replace characters and substrings in a string.
Syntax:
echo "$variableName" | tr [character(s)ToBeRemoved] [character(s)ToBeAdded]
Script Example:
STR="ABCD"
echo "$STR" | tr A Z
Output:
ZBCD
The character "A"
in the string variable $STR
is replaced with the character "Z"
. It is important to note that the above command will also work for replacing all instances of the character "A"
.
Script Example:
STR="ABACAD"
echo "$STR" | tr A Z
Output:
ZBZCZD
For more complex use cases such as replacing a single character with multiple characters or replacing substrings with other substrings, the tr
command can still be used, but it would be necessary to incorporate and use regular expressions(RegEx
) to accomplish such tasks.
Another useful command option with tr
is the squeeze or -s
option, which can replace a sequence of characters or a substring with a single character.
Script Example:
STR="ABBBCDDDDDE"
echo "$STR" | tr -s BD Z
Output:
AZCZE
As can be seen, multiple adjacent occurrences of the characters “B” and “D” were replaced by a single “Z”.
Method 2 - Use Bash Parameter Expansion
The $
symbol is commonly preceded with a variable name to assign the variable a value or to print its contents. However, we can also use it for Parameter Expansion.
We can use a parameter expansion to replace the contents of a variable with a given substring in the following way.
Syntax:
${varName/substring/replacement}
Please note that the use of braces is optional with the variable expansion.
Script Example:
STR="I LIKE FOOTBALL"
echo ${STR/FOOTBALL/CRICKET}
Output:
I LIKE CRICKET
Note that using a single forward slash after the variable name in the parameter expansion command replaces the first occurrence of the substring only. Use the following script to replace all occurrences of a substring in a string or double forward slashes.
Script Example:
${varName//substring/replacement}
Method 3 - Use the sed
Command
The sed
command is similar to the tr
command. Still, it is more powerful as it can be used to edit text files directly without opening them. sed
command in BASH stands for stream editor. It performs many functions on files like searching, finding and replacing, and insertion or deletion.
The sed
command can be used for string replacement in the following way.
Script Example:
STR=AZC
echo "$STR" | sed -r 's/[Z]+/B/g'
Output:
ABC
This is similar to using the tr
command. The -r
option after the sed
command indicates replacement, and pattern matching(RegEx
) describes what to find and replace it with.
Note that the syntax for the sed
command varies slightly between bash versions on different systems, which may cause unforeseen behavior.