How to Rename a File in Bash
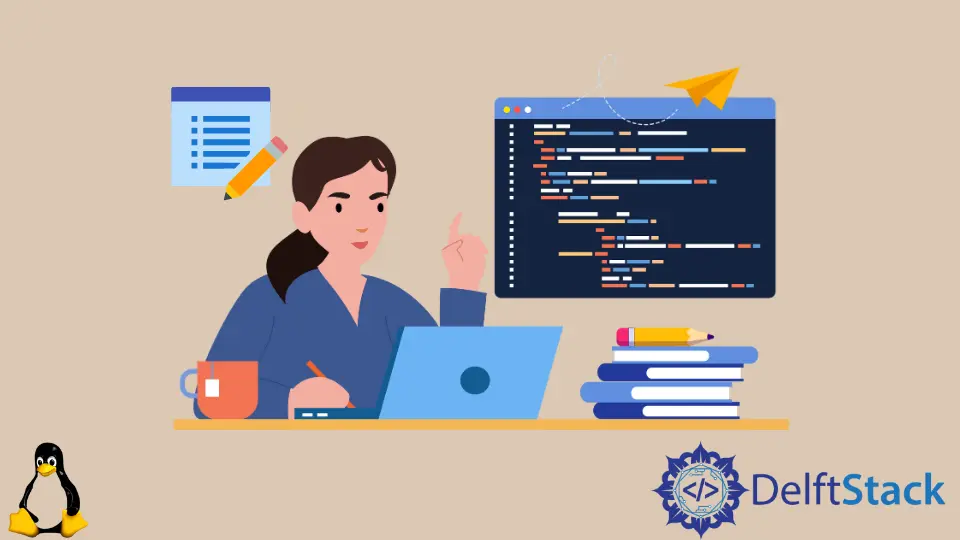
With the help of Bash script, you can automate your task. File renaming is a common task for all kinds of systems.
You can rename all the files one by one manually. But if you have a sequence on your filename, it’s better to automate this task.
This way, you can easily rename all files in a directory with a proper sequence.
In this article, we are going to introduce a way through which you can easily rename all the files in a directory. Also, we will see necessary examples and explanations to make the topic easier.
Use the mv
Command to Rename a File in Bash
The Bash script contains a built-in command known as mv
. The mv
command is mainly used to move directories and files from one location to another.
It also can be used for renaming a file and directory. An important thing about this command is that if you don’t specify the file’s new name, the filename will be the same in its new location.
The general syntax for this command is $ mv [OPTIONS] SOURCE DESTINATION
. The available options for this command are:
mv -f
- This flag will force to move by overwriting a destination file without prompt.mv -i
- This flag is for the interactive prompt before overwriting.mv -u
- This flag is for the update. This will only move the file when it’s a new source than the destination.mv -v
- This flag will print all the files of a source and destination.man mv
- This flag will open the help manual.
Suppose we have a list of files with the following sequence.
1_file.txt
2_file.txt
3_file.txt
4_file.txt
5_file.txt
6_file.txt
Now, look at the below example of code.
for file in *.txt
do
mv "$file" "${file/_file.txt/_Textfile.txt}"
done
In the example above, we renamed all the files with the type of .txt
. To do that, we used a for
loop with the command mv
.
Now the general syntax for this purpose is mv "$LoopVar" "${LoopVar/PreviousName.txt/NewName.txt}"
.
Here you have to note that you need to include a loop variable with the file name; otherwise, it may cause overwrite as it’s a system-generated name.
Now when you run the above code, you will see that all of your files are renamed like the below.
1_Textfile.txt
2_Textfile.txt
3_Textfile.txt
4_Textfile.txt
5_Textfile.txt
6_Textfile.txt
An important thing for this command is to specify a common part of the filename to identify the files. It can be the file type or any other common pattern in the filename.
Otherwise, the command may not successfully rename all the files.
Use the rename
Command to Rename a File in Bash
There is also a third-party command named rename
in Bash script. But you need to install it before using it.
To install this command with your shell environment, you can follow the below command for Ubuntu and Debian.
sudo apt install rename
After that, you can rename files like the one below.
rename [Your Options] 's/[Current Filename]/[New Filename]/' [Filename]
This command also contains some options like the one below.
-a
- This option will replace all the filename except the first one.-f
- This option will force an overwrite of existing files.-h
- This option will display the help text.-i
- This option will display a prompt notification before overwriting existing files.-l
- This option will replace the last occurrence element instead of the first one.-n
- This option performs a dry run.-s
- This option renames the target instead of the symlink.-v
- This option shows the output in the verbose version.-V
- This option displays the command version.
We can rename the above set of files by using the command below.
rename -v 's/_Testfile/_Test/' *.txt
And it will show the below output.
1_Testfile.txt renamed as 1_Test.txt
2_Testfile.txt renamed as 2_Test.txt
3_Testfile.txt renamed as 3_Test.txt
4_Testfile.txt renamed as 4_Test.txt
5_Testfile.txt renamed as 5_Test.txt
6_Testfile.txt renamed as 6_Test.txt
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn