How to Find File Size in Bash
-
Use the
ls
Keyword to Find the File Size in Bash -
Use the
wc
Keyword to Find the File Size in Bash -
Use the
stat
Keyword to Find the File Size in Bash
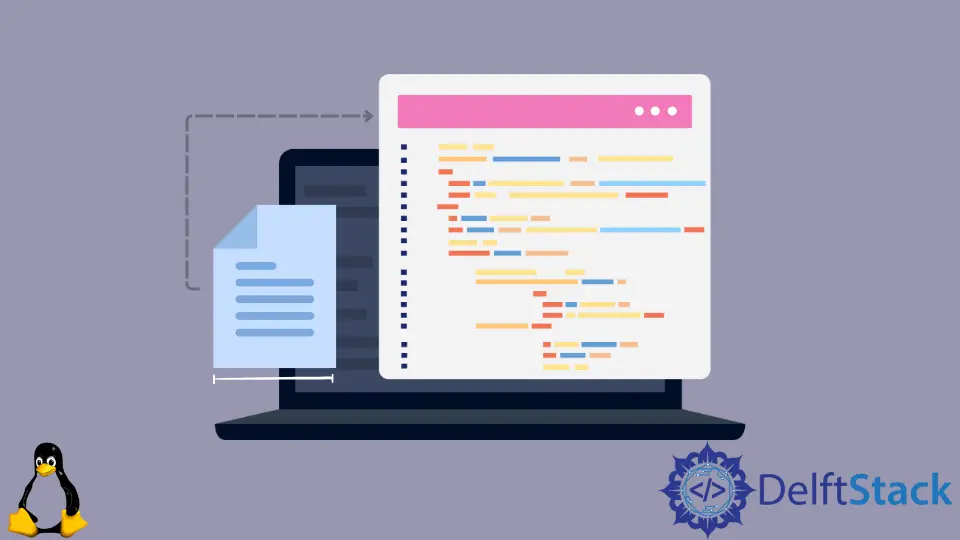
If you are creating a Bash script that can transfer files from one location to another, you may need to know the size of the file you are working on.
In a Bash script, there are several ways to find the size of a file. This article will show how to find the size of a file through Bash.
We will discuss three different methods here, and you can choose the perfect one based on your needs. Also, we will see necessary examples and explanations to make the topic easier.
Use the ls
Keyword to Find the File Size in Bash
We can find the size of a file by using a built-in command on Bash, which is the keyword ls
. Through this keyword, you can find the size of a file by following the example below:
ls -lh Test.txt | awk '{print $5}'
After running this command, you will get an output like the one below:
99
Above, we shared a very simple format of this method. In the example below, we will share an organized way to find out the file size.
The updated example is:
echo "Enter the directory or name of the file:"
read File
FileSize=$(ls -lh $File | awk '{print $5}')
echo "$File has a size of $FileSize"
In the example above, we take the filename from the user input and then provide the file size as an output.
After running this example, you will get the output below:
Enter the directory or name of the file:
Test.txt
Test.txt has a size of 99
Use the wc
Keyword to Find the File Size in Bash
Another keyword available in Bash that you can use for finding the file size is wc
. This simplest way to use this command is shared below:
wc -c Test.txt
After executing the above line of command, you will get the output below:
99 Test.txt
Now we are going to introduce an advanced example. In our below example, we will provide an option for users to select the file size format.
Our advanced example contains the below codes.
echo "Select the FORMAT for the size:"
echo """
1. Bytes
2. KiloBytes
3. MegaBytes
4. GigaBytes
"""
echo "--------------------------------------------------------"
read FORMAT
echo "Provide the Filename or Directory: "
read FILE
FileSize=$(wc -c $FILE | awk '{print $1}')
if [[("$FORMAT" == 1)]];
then
echo "$FILE is approx $FileSize Bytes"
elif [[("$FORMAT" == 2)]];
then
kb=$(bc <<<"scale=3; $FileSize / 1024")
echo "$FILE is approximately $kb KB"
elif [[("$FORMAT" == 3)]];
then
mb=$(bc <<<"scale=6; $FileSize / 1048576")
echo "$FILE is approximately $mb MB"
elif [[("$FORMAT" == 4)]];
then
gb=$(bc <<<"scale=12; $FileSize / 1073741824")
echo "$FILE is approximately $gb GB"
else
echo "Incorrect FORMAT."
exit
fi
In the example above, we first provided an option to the user and read the user input. In the next portion, we took the user input for the filename.
Afterward, we converted the file size based on the user selection and provided the result.
When you run this code, you will get the output below:
Select the FORMAT for the size:
1. Bytes
2. KiloBytes
3. MegaBytes
4. GigaBytes
--------------------------------------------------------
1
Provide the Filename or Directory:
Test.txt
Test.txt is approx 99 Bytes
Use the stat
Keyword to Find the File Size in Bash
Our last method will introduce another built-in keyword in Bash, stat
. This keyword mainly provides all the necessary information related to the file.
The simplest way to find the file size is as follows:
stat Test.txt
The single-line command shared above will provide you with the file size and other details like the name, birth, etc. You will get the output below after executing the command above:
File: Test.txt
Size: 99 Blocks: 0 IO Block: 4096 regular file
Device: 11h/17d Inode: 281474976715600 Links: 1
Access: (0777/-rwxrwxrwx) Uid: ( 1000/ aminul) Gid: ( 1000/ aminul)
Access: 2022-08-04 14:57:18.556623600 +0600
Modify: 2022-08-04 14:45:27.259462300 +0600
Change: 2022-08-04 14:45:27.259462300 +0600
Birth: -
The example shared above contains some unnecessary information about the file. But in the below example, you can eliminate these.
Our next updated code regarding this method is as follows:
echo "Enter the path or directory: "
read FilePath
FileSize=$(stat -c %s $FilePath)
echo "$FilePath is precise $FileSize bytes."
After executing the above example code, you will get the output below:
Enter the path or directory:
Test.txt
Test.txt is precise 99 bytes.
Please note that all the code used in this article is written in Bash. It will only be runnable in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn