Differences Between val and var in Kotlin
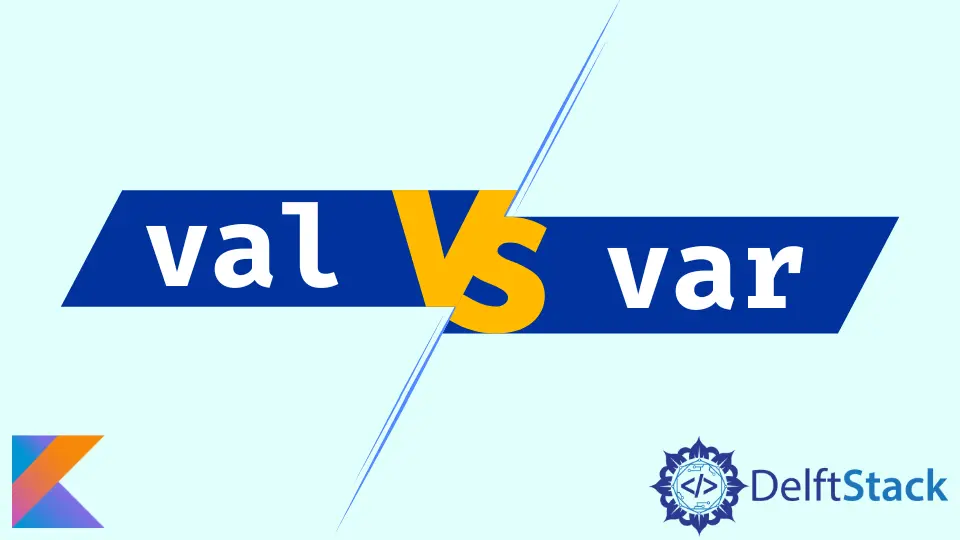
Kotlin allows declaring two variable types: val
and var
.
This article will discuss the difference between the two.
While both the keywords are used to declare variables, there are some key differences between them.
Differences Between val
and var
in Kotlin
The most significant difference between val
and var
properties is that the former is immutable. We can change the value of var
variables, but we cannot alter the value of a val
variable.
They are similar to the final
keyword in Java. Some other differences between the val
and var
variables are:
- The
var
variables can be assigned multiple times, whereas theval
variables can be assigned only once. - The
var
variables can be reassigned, but theval
variables cannot be reassigned.
Now that we know the difference between val
and var
, let’s look at some examples where we try to change their values.
Use the var
Keyword in Kotlin
In this example, we will use the var
keyword to declare a variable and try to change its value.
var v = "Hello!"
fun main() {
println("This is a var variable: "+v);
// Changing the value of var variable
v = "Welcome!";
println("New value of the var variable is: " +v);
}
Output:
Click here to check the demo of the example code above.
Use the val
Keyword in Kotlin
Here, we will create an immutable variable using the val
keyword and try to change its value.
val v = "Hello!"
fun main() {
println("This is a val variable: "+v);
// Changing the value of val variable
v = "Welcome!";
println("New value of the val variable is: " +v);
}
Output:
As we can see in the output, changing the val
variable throws an error: Val cannot be reassigned
.
Click here to check the demo of the example code.
Change the Properties of val
Variables in Kotlin
While we cannot change the value of the variables declared using the val
keyword, we can still change their properties. Let’s look at an example where we declare a variable using val
and change its properties.
fun main(args: Array<String>) {
val student = Student("David Shaw",1)
print(student)
student.name = "Joe Smith"
print("\n"+student)
}
data class Student(var name: String = "", var id: Int = 0)
Output:
As we can see, the properties of a val
variable are mutable.
Click here to check the demo of the example code.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn