How to Use the by Keyword in Kotlin
-
Uses of the
by
Keyword in Kotlin -
Use the
by
Keyword to Delegate Implementation of a Property to Another Object in Kotlin -
Use the
by
Keyword to Delegate Implementation of an Interface to Another Object in Kotlin
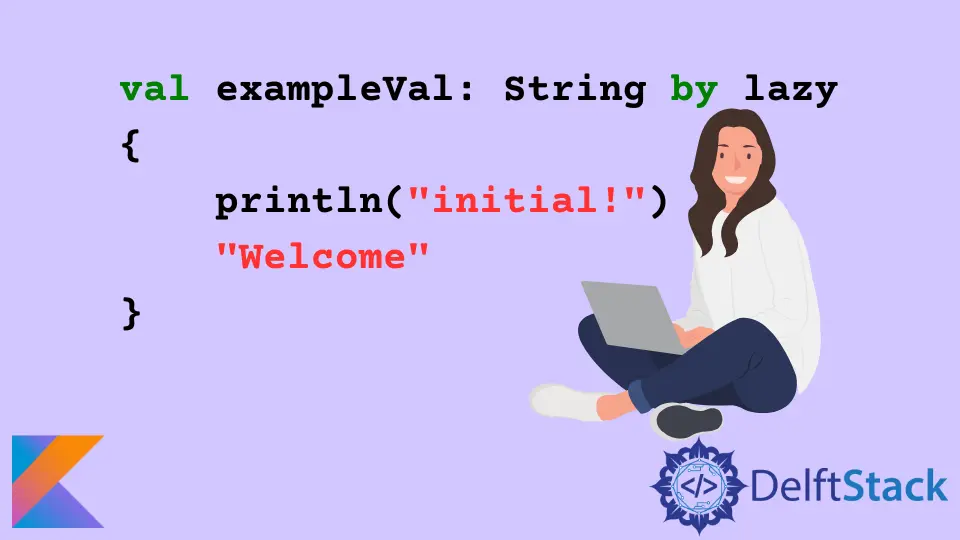
by
in Kotlin is a soft keyword, meaning it is helpful as both a keyword and an identifier based on the context. The by
keyword means provided by.
Kotlin automatically creates getter()
and setter()
for every property. When we define a var
property, it gets a get()
and set()
methods, and a val
property gets a get()
method by default.
The Kotlin by
keyword becomes helpful when we want to define these methods somewhere else, like for a function and not during a property initialization.
When we use the by
keyword, instead of the default way, the get()
and set()
methods are provided by the function following the keyword, meaning they are delegated.
Uses of the by
Keyword in Kotlin
The by
keyword is helpful in two places: (i) delegating the implementation of interface and (ii) accessors of a property to another object.
As mentioned above, it is used to delegate the implementation of a property.
Syntax:
val/var <property_Name>: <Type> by <expression>
The most common uses of the Kotlin by
keyword with properties are:
- Lazy properties
- Observable properties
- Storing properties in a map
When it comes to implementing an interface to another object, it works similarly with a few primary differences.
- Instead of function, the
by
keyword is defined where a function is provided - Since it is used with an interface, it is also an effective alternative for implementation inheritance
Use the by
Keyword to Delegate Implementation of a Property to Another Object in Kotlin
This section will go through examples of the Kotlin by
keyword to delegate properties for each use case.
Use the by
Keyword With Lazy Properties in Kotlin
In Kotlin, the lazy()
function takes a lambda as an input and returns an instance of Lazy
When using the by
keyword, the first get()
function call executes the lambda expression and stores the result temporarily. The second get()
function call returns the result.
Here’s an example to demonstrate how it works.
val exampleVal: String by lazy {
println("initial!")
"Welcome"
}
fun main() {
println(exampleVal)
println(exampleVal)
}
Output:
Use the by
Keyword With Observable Properties in Kotlin
Here’s an example demonstrating how the Kotlin by
keyword works with observable properties.
import kotlin.properties.Delegates
class Student {
var Name: String by Delegates.observable("David") {
value, oldValue, newValue ->
println("$oldValue -> $newValue")
}
}
fun main() {
val stud = Student()
stud.Name = "John"
stud.Name = "Doe"
}
Output:
As seen in the output, the observable properties take two arguments: initial value and modification handler.
The handler runs every time we assign a value to the property. Hence, we have three parameters after the assignment: the initial property used for the assignment, the old value, and the new value.
Use the by
Keyword for Storing Properties in a Map in Kotlin
Storing properties in a map allows performing dynamic tasks like parsing JSON. Here’s an example that shows how we can delegate the properties and store them on a map.
class Student(val map_example: Map<String, Any?>) {
val name: String by map_example
val ID: Int by map_example
}
fun main() {
val s = Student(mapOf(
"name" to "John Doe",
"ID" to 301
))
println(s.name)
println(s.ID)
}
Output:
Use the by
Keyword to Delegate Implementation of an Interface to Another Object in Kotlin
Besides delegating to properties, the Kotlin by
keyword allows the delegation of an interface to another object. Here’s an example to demonstrate that.
When a class is used with the by
keyword, it implements an interface by delegating all public members to a specific object.
interface Parent {
fun print()
}
class ParentImpl(val x: Int) : Parent {
override fun print() { print(x) }
}
class Child(p: Parent) : Parent by p
fun main() {
val p = ParentImpl(25)
Child(p).print()
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn