The let Keyword in Kotlin
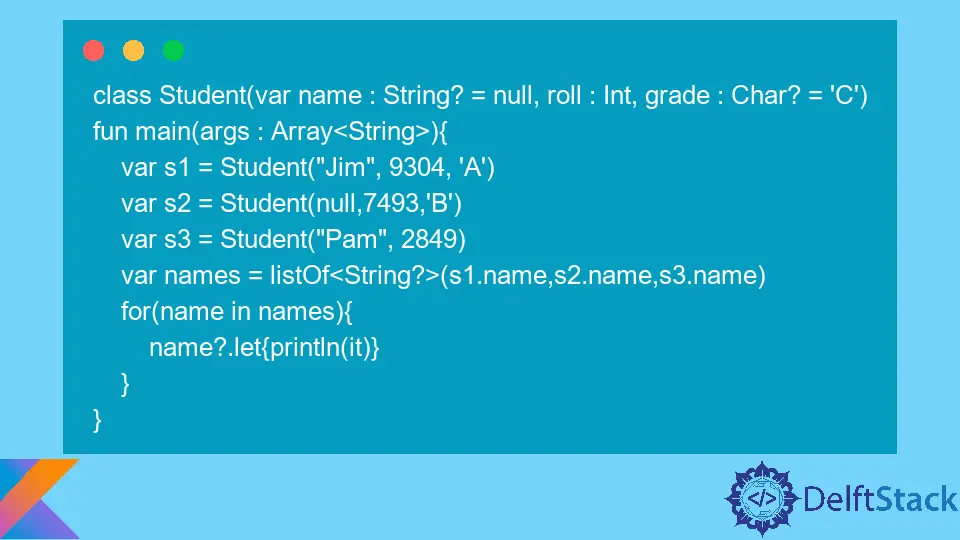
This tutorial teaches Scoping Function let
and how it is used in Kotlin.
the let
Function in Kotlin
Scoping functions provide a way to declare variables, perform required operations and return some value under temporary scope within the block of code, thereby resulting in a clean and compact code. let
is one of the 5 scoping functions, namely, run
, also
, apply
, with
, and let
.
let
function takes the object (on which it is called) as a parameter, performs operations on it, and returns the result of the lambda expression.
Variables can be declared inside the let expression, but these variables cannot be used outside the let expression as Kotlin let
is a scoping function.
The result can be of any data type. It is not necessary that result has to be of the same type as the object.
Syntax:
var var_name = object.let{
// lambda expression
// declare variables
// perform tasks
// return some value
}
We’ll use a var name
variable of the type var
, then we utilize a pre-defined type or a self-defined class. We can perform operations on these variables using the let
function, and access the object’s value by using the it
keyword, which refers to the object’s context.
Renaming the it
keyword will convert it to the lambda function. We can manipulate the variables and return the result of any data type that saves in var name
.
The let
function may or may not return any value. If the return value is not mentioned, it simply returns Kotlin.Unit
.
fun main(){
var str = "Hello"
// let function returning string
var temp1 = str.let {
var gm = "Good Morning"
"$it! $gm".toUpperCase()
}
// let function performing println function inside it
var temp2 = str.let{ greetings ->
for(i in 1..3){
println(greetings+" $i")
}
}
println(str)
println(temp1)
println(temp2)
}
Output:
Hello 1
Hello 2
Hello 3
Hello
HELLO! GOOD MORNING
kotlin.Unit
The above code shows that in the temp1
variable, we use the let
function using str
. Variable gm
is only accessible in the let
block.
The it
is a copy of str
, and we can also use another name using a lambda expression. let
function returns $str! $gm
in uppercase assigned to the temp1
variable.
In temp2
, greetings
is a variable with the same value as str
.
Lambda expression has a for
loop that prints greetings
value and i
. It doesn’t return any value.
So, when we print temp2
, Kotlin.Unit
is the output that denotes that variable is initialized with a unit value. Also, after two let
operations on the str
string, its value is not changed.
Use the let
Function for Null Checks in Kotlin
The let
function can perform operations only if the given variable is not null
. For example, if we want to print students’ names whose name is not null
in Student class.
class Student(var name : String? = null, roll : Int, grade : Char? = 'C')
fun main(args : Array<String>){
var s1 = Student("Jim", 9304, 'A')
var s2 = Student(null,7493,'B')
var s3 = Student("Pam", 2849)
var names = listOf<String?>(s1.name,s2.name,s3.name)
for(name in names){
name?.let{println(it)}
}
}
Output:
Jim
Pam
In the above example, we create a Student
class with three parameters, name
, roll
, and grade
. The student’s name is initially null
.
The ?
denotes that this parameter can be null
. Then, we create three objects of the Student class in the main function.
s1
has all the three parameters, not null
. s2
has two parameters, roll and grade, but the name is null
, and s3
has two parameters name and roll, but the grade is not mentioned.
The variable names
is a list of student names, and we visit each name using the for
loop. The name?.let{println(it)}
firstly checks if the name is null
, and if it is null
, let
won’t be executed.
If it is not null
, the student’s name is sent to let
, and let
will print the name. it
is a copy of the name object referring to the name itself.
Niyati is a Technical Content Writer and an engineering student. She has written more than 50 published articles on Data Structures, Algorithms, Git, DBMS, and Programming Languages like Python, C/C++, Java, CSS, HTML, KOTLIN, JavaScript, etc. that are very easy-to-understand and visualize.
LinkedIn