How to Use the with Keyword in Kotlin
- Generate a Kotlin Project
- Invoke an Object Explicitly in Kotlin
-
Use the
with
Keyword to Call an Object Implicitly Without a Return Value in Kotlin -
Use the
with
Keyword to Call an Object Implicitly With a Return Value in Kotlin - Conclusion
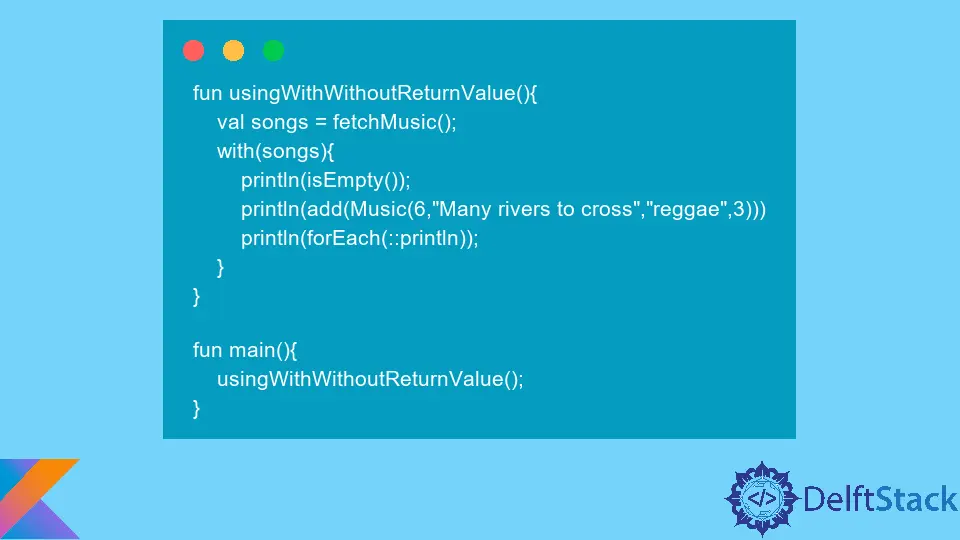
In object-oriented programming, we learned that objects are composed of properties and methods. To access these details, we call them explicitly by using the .
operator and then providing the field or property name.
This is the commonly used approach in most programming languages when working with objects, but it is not the only way. We can implicitly call an object’s member properties and methods using generics and lambda expressions.
This tutorial will teach us how to implicitly call an object’s member properties and methods.
Generate a Kotlin Project
Open IntelliJ development environment and select File > New > Project
. On the window that opens, enter the project name as kotlin-with
, select Kotlin on the Language section, and Intellij on the Build system section.
Create a file named Main.kt
under the src/main/kotlin
folder and copy and paste the following code into the file.
data class Music(val id: Int,
val title: String,
val genre: String,
val duration: Int);
fun fetchMusic(): ArrayList<Music>{
return arrayListOf(
Music(1,"Passing me by","Hip hop",5),
Music(2,"Lose yourself","Hip hop",3),
Music(3,"Smoke on the water","Classic songs",6),
Music(4,"Born to the wild","Classic songs",2),
Music(5,"Real love","Rhythm and blues",6)
);
}
This code creates a method named fetchMusic()
that returns an ArrayList of objects created using the Music
data class. We will use the list returned by the fetchMusic()
music method to illustrate our examples in the next sections.
Invoke an Object Explicitly in Kotlin
Copy and paste the following code into the Main.kt
file after the fetchMusic()
method.
fun callingAnObject(){
val songs = fetchMusic();
songs.forEach(::println);
}
fun main(){
callingAnObject();
}
The function named callingAnObject()
uses the list we have created above to explicitly call the forEach()
method to log all the items in the list to the console. Note how we explicitly call the list object songs.forEach()
as mentioned in the previous section.
In the next sections, we will see how to implicitly invoke the songs
object to get access to any member variables or methods. Run this example and ensure the output is as shown below.
Music(id=1, title=Passing me by, genre=Hip hop, duration=5)
Music(id=2, title=Lose yourself, genre=Hip hop, duration=3)
Music(id=3, title=Smoke on the water, genre=Classic songs, duration=6)
Music(id=4, title=Born to the wild, genre=Classic songs, duration=2)
Music(id=5, title=Real love, genre=Rhythm and blues, duration=6)
Use the with
Keyword to Call an Object Implicitly Without a Return Value in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after the comment.
fun usingWithWithoutReturnValue(){
val songs = fetchMusic();
with(songs){
println(isEmpty());
println(add(Music(6,"Many rivers to cross","reggae",3)))
println(forEach(::println));
}
}
fun main(){
usingWithWithoutReturnValue();
}
We usually use the with()
function in Kotlin when we want to invoke an object implicitly, as shown in this example. Note how we implicitly call the isEmpty()
, add()
, and forEach()
methods the list without using its object.
The with()
function uses this list as the receiver and calls any field or method of the list behind the scenes once we ask for them, and the result is returned. In this use case, the with()
function is not returning any values but logging them to the console.
In the next section, we will see how to return the result of the call made behind the scenes. Run this example and ensure the output is as shown below.
false
true
Music(id=1, title=Passing me by, genre=Hip hop, duration=5)
Music(id=2, title=Lose yourself, genre=Hip hop, duration=3)
Music(id=3, title=Smoke on the water, genre=Classic songs, duration=6)
Music(id=4, title=Born to the wild, genre=Classic songs, duration=2)
Music(id=5, title=Real love, genre=Rhythm and blues, duration=6)
Music(id=6, title=Many rivers to cross, genre=reggae, duration=3)
Use the with
Keyword to Call an Object Implicitly With a Return Value in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after the comment.
fun usingWithContainingReturnValue(): Music {
val songs = fetchMusic();
val song = with(songs) {
get(1)
}
return song;
}
fun main(){
println(usingWithContainingReturnValue());
}
In the previous example, we mentioned that with()
invokes a property or method of this list behind the scenes and returns the result of the call. This example shows to retrieve the result returned by the call.
In this example, we have used the with()
method to invoke the songs
object explicitly to return the object at index 1
using the get()
method. Note how we invoke the get()
method inside the with()
function without using its object.
Note that the object returned by the enclosing function is of type Music
. Run this code and ensure the output is as shown below.
Music(id=2, title=Lose yourself, genre=Hip hop, duration=3)
Conclusion
In this tutorial, we’ve learned how to use the with()
method that helps us implicitly invoke an object. The two use cases we have covered include calling an object implicitly without a return value and calling an object implicitly with a return value.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub