How to Get the Instance of an Object in Kotlin
-
Use
is
With Generics to Get the Instance of an Object in Kotlin -
Use
is
With thewhen
Keyword to Get the Instance of an Object in Kotlin - Conclusion
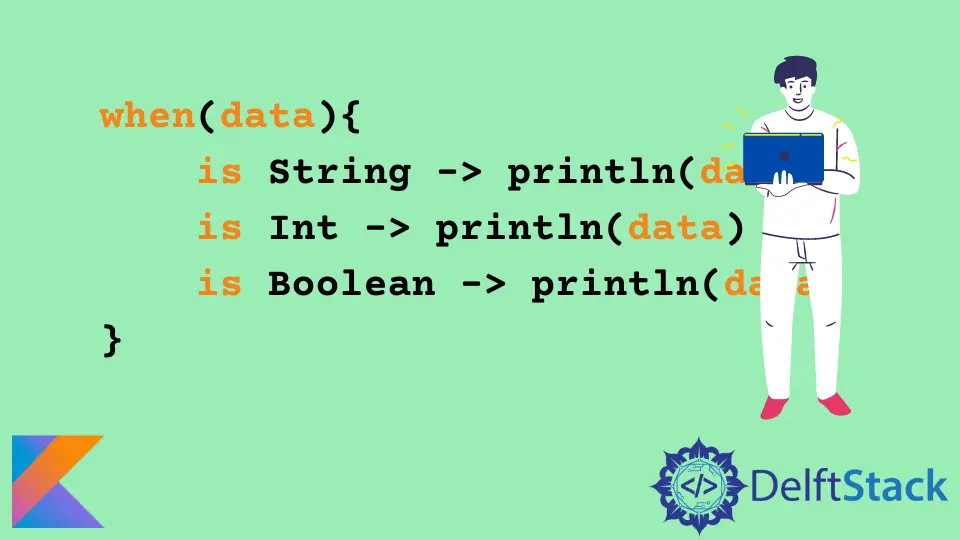
Getting the instance of an object is a common development practice we will encounter in most programming languages such as Kotlin, Java, TypeScript, JavaSript, and others. An example of a situation where you might want to find the instance of an element is when you want to sort elements based on their type.
When finding the instance of an element, the result is true if the element has the same type as the element being matched against; otherwise, it returns false.
In this tutorial, we will learn how to get the instance of an object at runtime using Kotlin.
Use is
With Generics to Get the Instance of an Object in Kotlin
Generic is a development approach used to design our code so it can be reused using any type.
Go to IntelliJ and select File > New Project
to create a new Kotlin project. Enter the project name as kotlinInstancOf
or any name you prefer.
Select Kotlin on the Language section and Intellij on the Build System section. Press the Create button to create the project.
Once the project is created, create the folder structure com/instanceOf
under the kotlin folder. Create a file named Main.kt
under the instanceOf
folder, and copy and paste the following code into the file:
package com.instanceOf
interface Employee{
fun work(): String;
}
class Manager(private val name: String): Employee{
override fun work(): String {
return "$name is preparing the project schedule";
}
}
class Janitor(private val name: String): Employee{
override fun work(): String {
return "$name is cleaning the office";
}
}
fun <T> doWork(Object: T): String {
if (Object is Employee){
return Object.work();
}else{
throw RuntimeException("$Object is not of type Employee")
}
}
fun main() {
println(doWork(Manager("John")));
println(doWork(Janitor("Peter")));
println(doWork("mary"));
}
In this example, we have created an interface named Employee
and two concrete implementations named Manager
and Janitor
.
The doWork()
is a generic method defined above, which uses the is
keyword to find whether the object passed as an argument is of the same type as Employee
. If the condition returns true, we invoke the work()
method of the object; otherwise, we throw a RuntimeException()
.
In the main method, we call the doWork()
method 3 times and pass the Manager
, Janitor
, and a string as arguments, respectively.
The first two invocations of the method are evaluated as true because they have a relationship with the Employee
. But the last invocation is evaluated as false because the string argument does not have a relationship with the Employee
.
Run the code to verify the output is as shown below.
Exception in thread "main" java.lang.RuntimeException: mary is not of type Employee
John is preparing the project schedule
Peter is cleaning the office
Since the is
keyword returns a boolean, we can return the reverse of the is
keyword by adding the character !
before the keyword. The character !
is usually pronounced as not
, which is common when working with logic gates.
Comment the doWork()
method and replace it with the following code:
fun <T> doWork(Object: T): String{
if (Object !is Employee){
throw RuntimeException("$Object is not of type employee");
}
return Object.work();
}
Rerun the code and note that the result is the same as the output in the previous example:
John is preparing the project schedule
Peter is cleaning the office
Exception in thread "main" java.lang.RuntimeException: mary is not of type employee
Use is
With the when
Keyword to Get the Instance of an Object in Kotlin
The when
keyword works the same way the switch
keyword works with Java. The only difference is the syntax.
Comment on the previous example, and copy and paste the following code into the Main.kt
file after the comment.
fun fetchData() = listOf<Any>("book",3,true,"computer");
fun main() {
for (data in fetchData()) {
when(data){
is String -> println(data)
is Int -> println(data)
is Boolean -> println(data)
}
}
}
In the above example, we have created a fetchData()
method that returns a list of type Any
by assigning it to the listOf()
helper method. The listOf()
method returns a list containing String, Int, and Boolean types.
In the main
method, we determined the element type at runtime by iterating through the list using a loop and delegating each value to the when()
method. Inside the when()
block, the type of the value passed is determined using is
, and if the condition evaluates to true, the value is logged to the console.
You should note that the value will not be logged to the console if you add another type in the list without finding its type inside the when()
block.
Run the code and verify that the output is as shown below:
book
3
true
computer
Conclusion
In this tutorial, we have learned how to get the instance of an element at runtime leveraging the Kotlin programming language. The approaches covered include: using the is
keyword with generics and the is
keyword with the when
keyword.
Note that when using generics, we must ensure that we are using reified
types, which means that the type information is available at runtime; otherwise, the type-check will not work.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub