How to Clone Object in Kotlin
- Generate a Kotlin Project
-
Use the
copy()
Method of Data Class to Clone Object in Kotlin -
Use the
clone()
Method of ArrayList to Clone Object in Kotlin - Create an Extension Function to Clone Object in Kotlin
- Implement the Cloneable Interface to Clone Object in Kotlin
- Conclusion
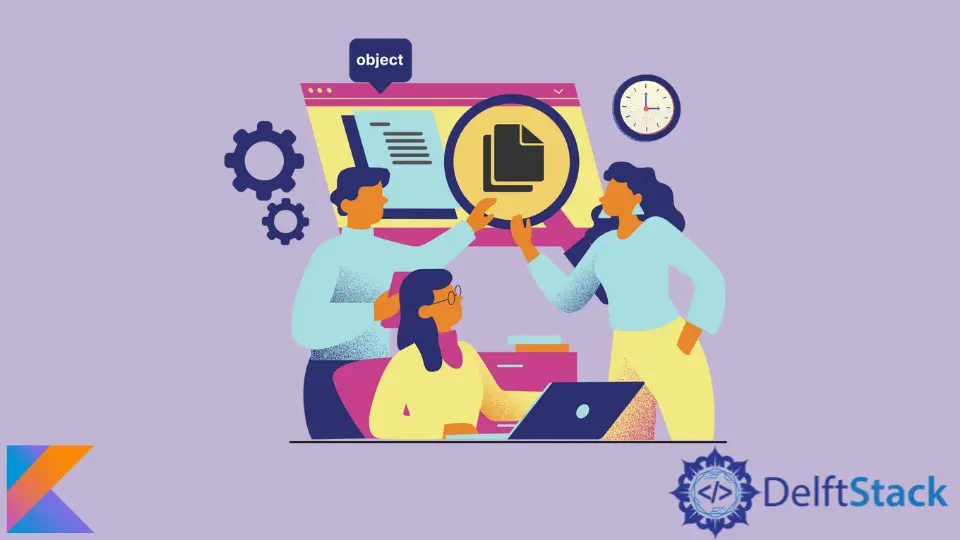
The cloning functionality is usually used in object-oriented programming languages. Cloning is defined as creating a copy of an object, which means that the copied object has the exact properties and methods of the original object.
Copying an object helps to avoid wastage of resources because it reuses the existing object without taking the whole process of creating an object and adding it to the Heap.
This tutorial will teach different approaches we can leverage to create a copy or a clone of an object.
Generate a Kotlin Project
Go to the IntelliJ development environment and select File>New>Project
. Enter the project name as kotlin-copy-object
, select Kotlin
in the Language section, and select Intellij
in the Build system section.
Create a file named Main.kt
under the folder src/main/kotlin
. Copy and paste the following code into the file.
data class Movie(var id: Int,
var name: String,
var genre: String,
var duration: Int);
fun fetchMovies(): ArrayList<Movie>{
return arrayListOf(
Movie(1,"Paws of fury","animation",60),
Movie(2,"Green lantern","animation",80),
Movie(3,"Hot seat","action",70),
Movie(4,"Chip n Dale","fantasy",60),
Movie(5,"Bullet train","mystery",80)
);
}
In this code, we have created a method named fetchMovies()
that returns an ArrayList of Movie
objects. The movie objects are created using a data class
that we have also defined in this code.
The properties of the movie objects include id
, name
, genre
, and duration. The id
property is of type int, and the other properties are of type string.
We will use the fetchMovies()
method in some examples covered in this tutorial to show how to make a copy of an object in Kotlin.
Use the copy()
Method of Data Class to Clone Object in Kotlin
A data class is a class used to hold data temporarily and provides the developer with some out-of-the-box functionalities. The data class provides the following methods that the developer can leverage without implementing their logic: equals()
, hashCode()
, toString()
, and copy()
.
Copy and paste the following code into the Main.kt
file after the fetchMovies()
method.
fun usingCopyOnDataClass(){
val movie: Movie = fetchMovies()[2];
val copyOfMovie: Movie = movie.copy();
println(copyOfMovie);
}
fun main(){
usingCopyOnDataClass();
}
In this code, we have used the fetchMovies()
method that returns an ArrayList of the Movie
objects to get the Movie
object at index 2.
Our objective is to make a copy of the object by leveraging the copy()
method provided by the data class. The copy()
method of the data class provides us with the same copy of our object.
Note: No new objects are created to realize this.
Note that you can also change some of the object’s properties while maintaining the rest by passing the values of the properties you want to change as the argument of the copy()
method.
For example, if you pass the argument copy(name = "Avengers")
, the name of the new copy of the object will be changed to the one you have provided, and the rest will not change.
Run this code and ensure the output is as shown below.
Movie(id=3, name=Hot seat, genre=action, duration=70)
Use the clone()
Method of ArrayList to Clone Object in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after the comment.
fun usingCloneWithArrayList(){
@Suppress("UNCHECKED_CAST")
val movies: ArrayList<Movie> =
fetchMovies().clone() as ArrayList<Movie>;
movies.forEach(::println);
}
fun main(){
usingCloneWithArrayList();
}
In this code, we have used the fetchMovies()
method to create a copy of the returned ArrayList instance using the clone()
method.
The clone()
method of ArrayList is inherited from the Object
class. This method returns the list instance, meaning the elements are not copied.
Since the clone()
method returns an Object
, we have used the as
keyword to cast it to ArrayList<Movie>
. Run this code and ensure the output is as shown below.
Movie(id=1, name=Paws of fury, genre=animation, duration=60)
Movie(id=2, name=Green lantern, genre=animation, duration=80)
Movie(id=3, name=Hot seat, genre=action, duration=70)
Movie(id=4, name=Chip n Dale, genre=fantasy, duration=60)
Movie(id=5, name=Bullet train, genre=mystery, duration=80)
Create an Extension Function to Clone Object in Kotlin
An extension function is a function that allows us to add functionality to an existing class without modifying the underlying code. Comment out the previous code and copy and paste the following code into the Main.kt
file after the comment.
fun Movie.clone(): Movie{
val movie = Movie(
this.id,
this.name,
this.genre,
this.duration);
return movie;
}
fun usingCloneExtensionFunction(){
val movie = fetchMovies()[4];
val clonedMovie = movie.clone();
println(clonedMovie);
}
fun main(){
usingCloneExtensionFunction();
}
In this code, we have created an extension function of the Movie
data class named clone()
. When the clone extension function is invoked, it returns the current instance of the object.
To use the clone()
extension function, we have used the fetchMovies()
method to retrieve the movie at index 4 and then invoked the clone()
function on it. This creates a copy of the same object as the object at index 4.
Run this code and ensure the output is as shown below.
Movie(id=5, name=Bullet train, genre=mystery, duration=80)
Implement the Cloneable Interface to Clone Object in Kotlin
When developing applications and you want a particular class to be copied, we usually add this functionality to the class by implementing the Cloneable
interface.
The Cloneable
interface has a method named clone()
that returns the string representation of your class. The only thing that we need to do is override the clone()
and toString()
methods.
Note that you must also make the clone()
method public
.
Comment out the previous example and copy and paste the following code into the Main.kt
file after the comment.
class Student(
private val firstName: String,
private val lastName: String): Cloneable{
public override fun clone(): Any {
return super.clone()
}
override fun toString(): String {
return "Student(firstName='$firstName', lastName='$lastName')"
}
}
fun implementingCloneable(){
val student: Student = Student("john","doe");
val clonedStudent = student.clone();
println(clonedStudent.toString());
}
fun main(){
implementingCloneable();
}
In this code, we have created a class named Student
with the properties firstName
and lastName
. This class implements the Cloneable
interface and overrides its clone()
method.
We have also overridden the toString()
method so that we can be able to log the cloned object to the console.
implementingCloneable()
creates an instance of a Student
object and invokes the clone()
method to make a copy of the object. Run this code and ensure the output is as shown below.
Student(firstName='john', lastName='doe')
Conclusion
In this tutorial, we have learned how to create a copy of an object in Kotlin. The approaches we have covered include using the copy()
method of data class, employing the clone()
method of ArrayList, creating an extension function, and using the Cloneable
interface.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub