When and Where to Use the Kotlin Companion Object
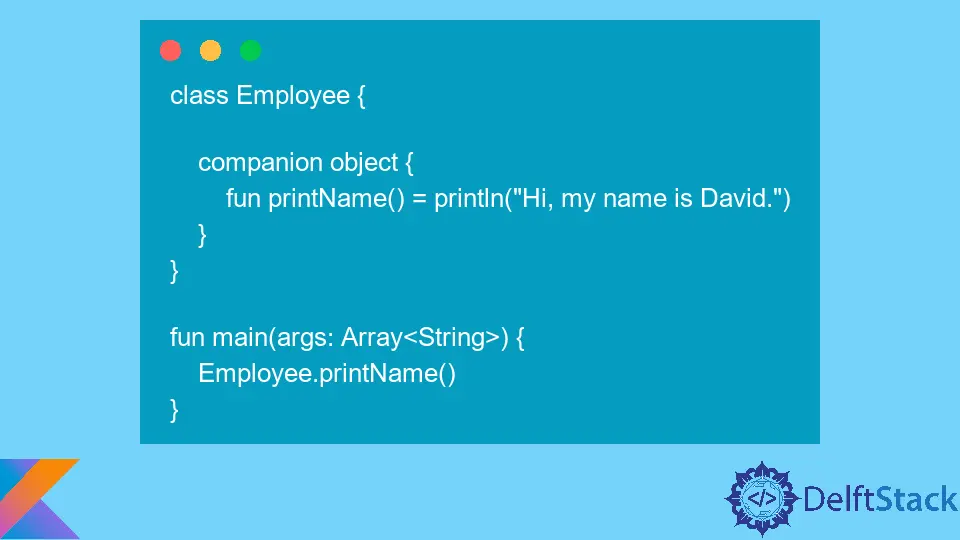
In any object-oriented programming language like Kotlin, Java, or C#, we have to create objects of a class to access its members. In Kotlin, it will look something like this:
class className{
fun funName() = // code will come here
}
fun main(){
val obj = className()
obj.funName() // calling the function using the class's object
}
However, there is a way to access a class’s method without creating an object. And that’s where Java’s static
and Kotlin’s companion
objects come into the picture.
In this article, we will learn about the companion
object and how to use it in Kotlin. But before getting into that, let’s look at an example of Java’s static
methods.
Java static
Methods
Java allows following an object-oriented programming approach without creating any object. It does so with the use of static
objects or instances.
The static
keyword enables the creation of static variables or methods inside a class. These variables or methods remain constant, and we cannot change them after initialization.
The most significant use of the static
keyword in Java is memory management. We can access static variables and methods without creating an object, which saves memory.
We can access such methods with the help of class names.
The Java program below demonstrates the same. The cubeNumber()
in this program is a static method.
It will take an integer parameter and return its cube.
import java.util.*;
public class Example {
public static void main(String[] args) {
int res = Example.cubeNumber(3);
System.out.println("Cube of 3 is: " + res);
}
public static int cubeNumber(int i) {
return i * i * i;
}
}
As we see, the cubeNumber()
is the static method in the above example. Hence, we could call it using the class name.
Kotlin companion
Object
Java has static
methods, but there is no static
in Kotlin. There is still a way to create such variables, but the approach is different.
In Kotlin, we use the keyword companion
object to create such methods.
The keyword companion
will work the same as the static
keyword in Java. It will allow access to methods created using the companion
keyword without any object.
Here’s an example to demonstrate the use of companion
objects in Kotlin. In this example, we will create a class Employee
.
We will then create a function to print the employee’s name using the companion
keyword and access it without the class’s object.
class Employee {
companion object {
fun printName() = println("Hi, my name is David.")
}
}
fun main(args: Array<String>) {
Employee.printName()
}
We can also omit the function’s name. In that case, we can use the name Companion
.
When Should We Use a Kotlin companion
Object?
The best scenario to use a Kotlin companion
object is when it does not interfere with the state of the class’s object. This means if you are using pure functions or final values that need to be accessed from outside the class itself, then you can use the Kotlin companion
object.
However, if the object interferes with the state of the class’s object, it is advised not to use the companion
object. This is because its use can lead to numerous errors.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn