Object vs Class in Kotlin
- Create Singleton Objects in Kotlin
- Create Objects Using a Class in Kotlin
- Create a Companion Object in Kotlin
- Create a Data Class in Kotlin
- Conclusion
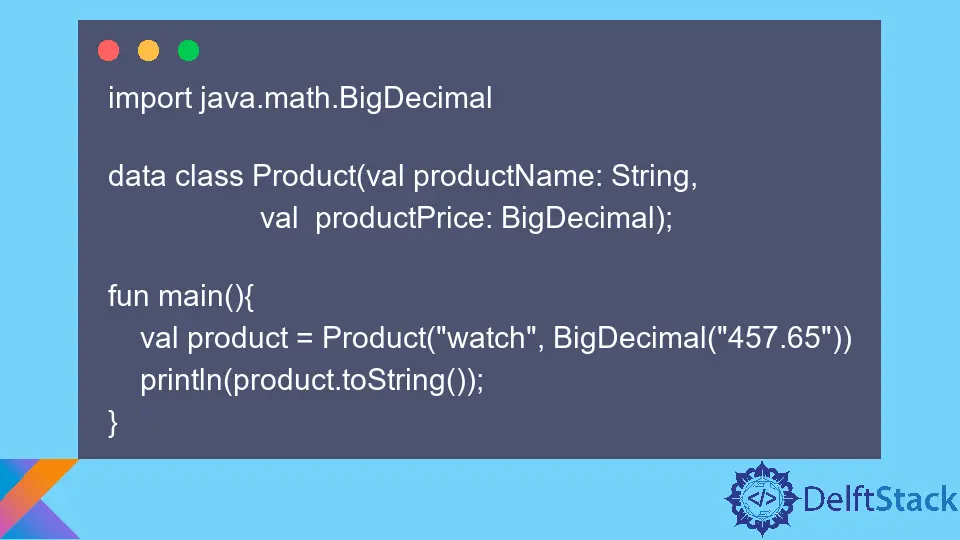
The class
and object
features are usually used in object-oriented programming, denoted as OOP.
Object-oriented programming is the programming approach where inheritance, polymorphism, abstraction, and encapsulation are the four basic principles of object-oriented programming.
Examples of languages that support object-oriented programming include Kotlin, Java, Python, JavaScript, TypeScript, and many others.
The main advantage of classes is that they help us to simulate real word things and objects and help us to create as many varying situations that behave differently and have different states.
For example, you might want to create an application that helps you design cars; we know that there are different types of cars, and they have different states such as color, number of seats, and others.
To achieve this, we use a class to create a car prototype, and from this prototype, we can create cars that have different states from the other. In this tutorial, we will learn how to use classes and objects in Kotlin.
Create Singleton Objects in Kotlin
There are different ways to create objects in Kotlin, which can exist in different forms. In this example, we will learn how to create a static object.
Open IntelliJ development environment and select File
> New
> Project
. On the window that opens, enter the project name
as kotlin-objects-and-classes
, select Kotlin
on the Language
section, and IntelliJ
on the Build system
section.
Create a Main.kt
file under the kotlin
folder, and copy and paste the following code into the file.
object DBConnection{
private const val url = "http://localhost";
private const val port = 8080;
private const val DBName = "customerDB";
override fun toString(): String {
return "Connected to database $DBName using port $port at $url";
}
}
fun main(){
println(DBConnection.toString());
}
In this example, we have created a static instance of a class, even though we have not defined any class, but if we decompile this code to Java, we will find that a class is created behind the scenes and a singleton returned.
When we define this object as a static class instance, we refer to a singleton, meaning that only one copy of the object is created in the application’s lifetime.
Note that you can invoke member functions and variables of this object without making any call to it, as shown in the main()
function.
We only need to append the .
symbol on the object name to invoke and make any call as we have done with the toString()
method. Run the above example, and note that it works as expected.
Connected to database customerDB using port 8080 at http://localhost
Create Objects Using a Class in Kotlin
We have mentioned that a class helps us simulate real-world things and their behaviors, which creates a blueprint for these things.
The instances are the different objects created from the blueprint, and the following example shows how to create a class and an instance of the class.
Comment out the previous example, and copy and paste the following code into the Main.kt
file after it.
class Employee(private val firstName: String,
private val lastName: String,
private val salary: Number){
override fun toString(): String {
return "$firstName $lastName earns a salary of $$salary"
}
}
fun main(){
val john = Employee("john","doe",60000);
println(john.toString());
}
In this example, we have created the blueprint of an employee using the class
keyword, and this is the syntax that is always used when you want to create a class.
The class has three properties, firstName
, lastName
, and salary
, and one method, toString()
, that returns the details of the employee.
In the main
method, we have created an object of an employee by calling the class name Employee()
, also known as the constructor and passed the concrete properties of the customer.
We can create as many instances as we want by calling the constructor again and passing different properties to create different objects. Run this example to verify it works as expected.
john doe earns a salary of $60000
Create a Companion Object in Kotlin
In the first example, we have seen how to create a static object that has only one copy in its entire lifetime and does not belong to any class.
In static within a class, we can create static properties and functions that belong to a class using a companion object. The following example shows how to realize this.
Comment out the previous example, and copy and paste the following code into the Main.kt
file after it.
class Customer(private val name: String,
private val email: String,
private val orderItem: String){
companion object{
const val creditCard = 1
}
override fun toString(): String {
return "$name bought a $orderItem using the email $email";
}
fun getName(): String{
return this.name;
}
}
fun main(){
val customer = Customer("mary", "mary@gmail.com", "watch")
println(customer.getName()+" has "+ Customer.creditCard+ " credit card")
println(customer.toString())
}
Note that the static properties and functions are defined in the companion object, and the companion object is defined in the class.
When you want to access the static properties and functions, we use the same approach we used in the first example.
For example, the Customer.creditCard
is used to access the class’s static property creditCard
. Run this example to verify that it works as expected.
mary has 1 credit card
mary bought a watch using the email mary@gmail.com
Create a Data Class in Kotlin
Comment out the previous example, and copy and paste the following code into the Main.kt
file after it.
import java.math.BigDecimal
data class Product(val productName: String,
val productPrice: BigDecimal);
fun main(){
val product = Product("watch", BigDecimal("457.65"))
println(product.toString());
}
A data class is used to hold data and created using the data
keyword, which is added in front of the class
keyword.
There are conditions for creating the data class and include ensuring the primary constructor has at least one parameter, ensuring the parameters are declared using val
or var
, and that the data class cannot be open
, sealed
, abstract
or inner
.
The data class helps developers to avoid boilerplate in their code as it generates common functions used with classes such as toString()
, equal()
, hashCode()
, and others.
In this example, we have created a data class for a product, and when we invoke the toString()
method, the customer’s details are returned to us for free. Run this example to ensure that it works as expected.
Product(productName=watch, productPrice=457.65)
Conclusion
This tutorial taught us how to create different types of objects in Kotlin and objects from classes. The topics covered include creating a singleton object, creating objects using a class, creating a companion object, and creating a data class.
If you want to read more about objects and classes, visit the Kotlin documentation, which covers the concepts in detail.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub