在 Kotlin 中获取对象的实例
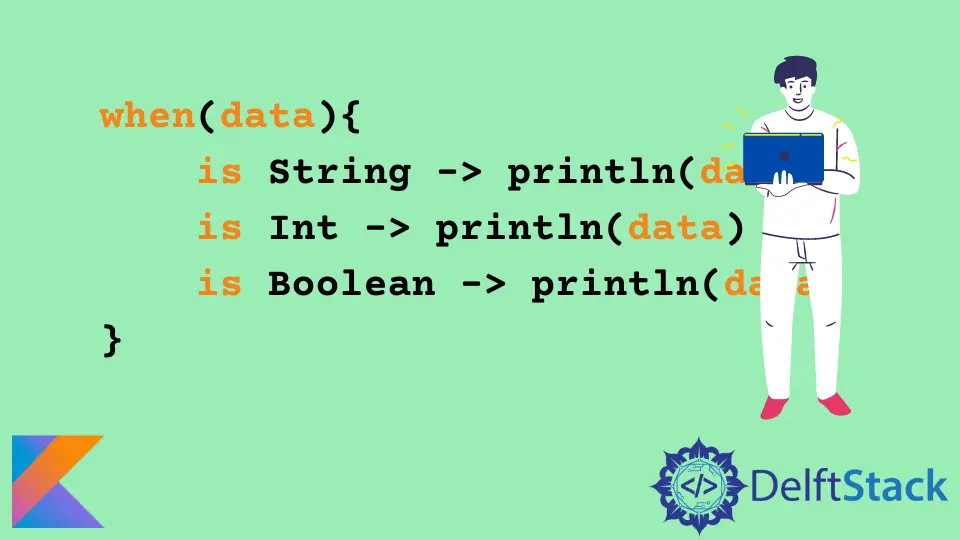
获取对象的实例是我们将在大多数编程语言(如 Kotlin、Java、TypeScript、JavaSript 等)中遇到的常见开发实践。你可能想要查找元素实例的情况的一个示例是,当你想要根据元素的类型对元素进行排序时。
在查找元素的实例时,如果该元素与被匹配的元素具有相同的类型,则结果为真;否则,它返回 false。
在本教程中,我们将学习如何使用 Kotlin 在运行时获取对象的实例。
在 Kotlin 中使用 is
和泛型获取对象的实例
泛型是一种用于设计我们的代码的开发方法,因此可以使用任何类型重用它。
转到 IntelliJ 并选择 File > New Project
创建一个新的 Kotlin 项目。将项目名称输入为 kotlinInstancOf
或你喜欢的任何名称。
在 Language 部分选择 Kotlin,在 Build System 部分选择 Intellij。按创建按钮创建项目。
创建项目后,在 kotlin 文件夹下创建文件夹结构 com/instanceOf
。在 instanceOf
文件夹下创建一个名为 Main.kt
的文件,并将以下代码复制并粘贴到该文件中:
package com.instanceOf
interface Employee{
fun work(): String;
}
class Manager(private val name: String): Employee{
override fun work(): String {
return "$name is preparing the project schedule";
}
}
class Janitor(private val name: String): Employee{
override fun work(): String {
return "$name is cleaning the office";
}
}
fun <T> doWork(Object: T): String {
if (Object is Employee){
return Object.work();
}else{
throw RuntimeException("$Object is not of type Employee")
}
}
fun main() {
println(doWork(Manager("John")));
println(doWork(Janitor("Peter")));
println(doWork("mary"));
}
在这个例子中,我们创建了一个名为 Employee
的接口和两个名为 Manager
和 Janitor
的具体实现。
doWork()
是上面定义的通用方法,它使用 is
关键字来查找作为参数传递的对象是否与 Employee
的类型相同。如果条件返回 true,我们调用对象的 work()
方法;否则,我们抛出一个 RuntimeException()
。
在 main 方法中,我们调用 doWork()
方法 3 次,并分别传递 Manager
、Janitor
和一个字符串作为参数。
该方法的前两次调用被评估为 true,因为它们与 Employee
有关系。但是最后一次调用被评估为假,因为字符串参数与 Employee
没有关系。
运行代码验证输出如下所示。
Exception in thread "main" java.lang.RuntimeException: mary is not of type Employee
John is preparing the project schedule
Peter is cleaning the office
由于 is
关键字返回一个布尔值,我们可以通过添加字符!
返回 is
关键字的反转。在关键字之前字符!
通常发音为 not
,这在使用逻辑门时很常见。
注释 doWork()
方法并将其替换为以下代码:
fun <T> doWork(Object: T): String{
if (Object !is Employee){
throw RuntimeException("$Object is not of type employee");
}
return Object.work();
}
重新运行代码,注意结果和上一个例子的输出一样:
John is preparing the project schedule
Peter is cleaning the office
Exception in thread "main" java.lang.RuntimeException: mary is not of type employee
使用 is
和 when
关键字在 Kotlin 中获取对象的实例
when
关键字的工作方式与 switch
关键字在 Java 中的工作方式相同。唯一的区别是语法。
对前面的示例进行注释,并将以下代码复制并粘贴到注释后的 Main.kt
文件中。
fun fetchData() = listOf<Any>("book",3,true,"computer");
fun main() {
for (data in fetchData()) {
when(data){
is String -> println(data)
is Int -> println(data)
is Boolean -> println(data)
}
}
}
在上面的示例中,我们创建了一个 fetchData()
方法,通过将其分配给 listOf()
辅助方法来返回 Any
类型的列表。listOf()
方法返回一个包含 String、Int 和 Boolean 类型的列表。
在 main
方法中,我们在运行时通过使用循环遍历列表并将每个值委托给 when()
方法来确定元素类型。在 when()
块中,传递的值的类型使用 is
确定,如果条件评估为真,则将值记录到控制台。
你应该注意,如果你在列表中添加另一种类型而没有在 when()
块中找到它的类型,则该值不会记录到控制台。
运行代码,验证输出如下图:
book
3
true
computer
结论
在本教程中,我们学习了如何利用 Kotlin 编程语言在运行时获取元素的实例。涵盖的方法包括:将 is
关键字与泛型一起使用,将 is
关键字与 when
关键字一起使用。
请注意,在使用泛型时,我们必须确保我们使用的是 reified
类型,这意味着类型信息在运行时可用;否则,类型检查将不起作用。
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub