The Ultimate Guide to Initializing Lists in Kotlin
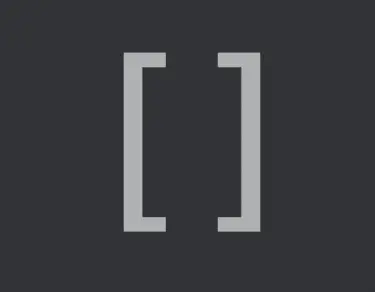
A Kotlin List<T>
refers to a collection of multiple elements. The <T>
in the syntax denotes an undefined data type. This means that a list initialized using List<T>
has no specific data type.
We can easily access and modify the items from a List
whenever we want using predefined functions from the Collections
class.
We can initialize two types of lists in Kotlin, which are immutable and mutable. There are different ways to initialize these lists, and we will see them in this article.
Initialize Immutable Lists in Kotlin
Immutable lists are collections that cannot be changed once they are created. They are defined using the List
interface and represented by the List
implementation classes such as List
and ArrayList
.
We will learn about three ways to initialize immutable lists. These three methods include the following:
listOf()
emptyList()
listOfNotNull()
Initialize Kotlin Lists With the listOf()
Function
The listOf()
is the top-level function from the Collections
class. This method creates an immutable list of items. It is similar to the use of Arrays.asList
in Java.
You can initialize immutable Kotlin lists using the following syntax.
val listName = listOf(item1, item2, item3)
Now, we will create a simple Kotlin program using the above syntax. In this program, we will create an immutable Kotlin List
named Fruits
and add a few fruits.
fun main(args: Array<String>) {
val Fruits = listOf("Apple", "Mango", "Strawberry")
println(Fruits)
}
This is an immutable list. Hence, using predefined methods to alter the list will result in an error.
We will see this in action by using the add()
function to try and add an element to the same immutable list we defined in the previous example.
fun main(args: Array<String>) {
val Fruits = listOf("Apple", "Mango", "Strawberry")
println(Fruits)
Fruits.add("Kiwi")
println(Fruits)
}
As we see in the output, the add()
method throws an unresolved reference: add
error.
Initialize Kotlin Lists With the emptyList()
Function
The next way to create an immutable Kotlin List
is by using the emptyList()
function. It works similarly to the listOf()
function, the only difference being that it creates an empty list.
This means it will create a list, but there will be no initialization, as the list will be empty.
The syntax of using emptyList()
function is like this:
val listName: List<String/Int> = emptyList()
Here’s a program to demonstrate the use of emptyList()
to create an empty immutable list in Kotlin.
fun main() {
val emptyImmutableList: List<String> = emptyList()
println(emptyImmutableList)
}
Initialize Kotlin Lists With the listOfNotNull()
Function
The listOfNotNull()
function returns a new list from an existing collection without null
.
If you have a list with some String
and some null
values, this method will give you a new list with only the String
values. But if the list only has null
values, the function will return an empty list.
The resulting list is read-only. Hence, the listOfNotNull()
function acts as a way to initialize an immutable Kotlin list.
Here’s the syntax of the listOfNotNull()
function.
fun <T : Any> listOfNotNull(element: T?): List<T>
In the below program, we will create three lists. One will have only null
values, the second will have only non-null
values, and the third will have a mix of null
and non-null
values.
fun main(args: Array<String>) {
val nullValuesList = listOfNotNull(null, null)
println(nullValuesList)
val nonNullValuesList = listOfNotNull(13, "Hello", "Hi", 96)
println(nonNullValuesList)
val mixList = listOfNotNull(13, null, "Hello", null, 96)
println(mixList)
}
The first println()
function results in an empty list because there are only two null
values in the first list, nullValuesList
. The second println()
function prints all the elements of the second list nonNullValuesList
because it only has non-null
values.
However, the third println()
function prints a list containing only non-null
values from mixList
.
Initialize Mutable Lists in Kotlin
Mutable lists are changeable collections that we can access and alter when required. This section will look at two ways to initialize Kotlin lists.
The two methods we will go through are the following:
mutableListOf()
arrayListOf()
Initialize Kotlin Lists With the mutableListOf()
Function
The first way to initialize mutable Kotlin lists is with the mutableListOf()
method. Like the listOf()
function, mutableListOf()
also allows creating and initializing a Kotlin List
.
However, this function creates a mutable list, meaning you can add or delete the elements from this list.
The syntax of mutableListOf()
is:
var listName = mutableListOf(item1, item2, item3)
We will create a Kotlin program below to create and initialize a mutable list named Fruits
. Next, we will add a new element to the list and remove another item.
fun main(args: Array<String>) {
var Fruits = mutableListOf("Apple", "Mango", "Strawberry")
println(Fruits)
// Adding a new element to the list
Fruits.add("Kiwi")
println(Fruits)
// Removing an element from the list
Fruits.remove("Mango")
println(Fruits)
}
As we see in the output, the add()
function does not throw an unresolved reference
error this time. This is because the list we created is mutable.
Initialize Kotlin Lists With the arrayListOf()
Function
The other way to initialize a mutable Kotlin list
is with the arrayListOf()
function. This method creates and initializes an array.
The syntax of arrayListOf()
function is:
var listName = arrayListOf(item1, item2, item3)
We will use the above syntax to create a mutable Kotlin ArrayList
named Cars
and initialize it with a few automotive brands. We will then add new elements to the ArrayList
to confirm that it is mutable.
fun main(args: Array<String>) {
var Cars = arrayListOf("Mercedes Benz", "BMW", "Bentley")
println(Cars)
Cars += listOf("Ferrari", "Lamborghini")
println(Cars)
}
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn