How to Create Lists in Kotlin
- Create an Immutable List in Kotlin
- Create a Mutable List in Kotlin
-
Use
listOfNotNull()
Method to AvoidNull
in Kotlin List
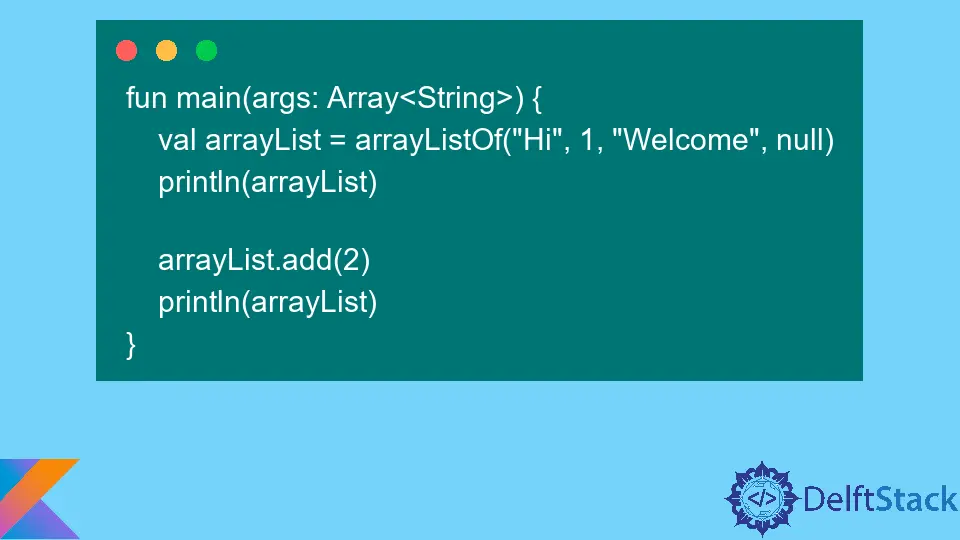
A Kotlin list stores different elements, also known as items, in order. We can access these elements using indices as they are stored in a specific order.
There are two types of lists: mutable and immutable. This article will teach us how to create mutable and immutable lists in Kotlin.
Create an Immutable List in Kotlin
Immutable lists in Kotlin allow only accessing and reading elements from a list. Once we create an immutable list, we cannot alter it.
We can create an immutable list in Kotlin using the standard library listOf()
. The example below demonstrates the use of listOf
to create an immutable list.
We will also access the second element of the list, whose index will be 1
.
Code:
fun main(args: Array<String>) {
val immutableList = listOf("Hi", 1, "Welcome", null)
println(immutableList)
println("\nSecond element: ${immutableList[1]}")
}
Output:
[Hi, 1, Welcome, null]
Second element: 1
If we try to add an element in the above list using the add()
function, it will throw an error. The reason is that the listOf()
library doesn’t have any such function, making it an immutable list.
Code:
fun main(args: Array<String>) {
val immutableList = listOf("Hi", 1, "Welcome", null)
println(immutableList)
immutableList.add(2)
}
Output:
Unresolved reference: add
Create a Mutable List in Kotlin
Mutable lists allow us to alter them after the creation. So, if you want to create a list that you might need to change later, mutable lists are the way to go.
There are two ways to create a mutable list in Kotlin. The first way is to use arrayListOf
() and the second is to use mutableListOf()
.
Use arrayListOf()
to Create a List in Kotlin
The arrayListOf()
function creates an ArrayList. This ArrayList is a mutable list.
We can alter the list using different functions, such as add()
, remove()
, removeLast()
, etc. We’ll use the same example above and add the number 2 to the list.
Code:
fun main(args: Array<String>) {
val arrayList = arrayListOf("Hi", 1, "Welcome", null)
println(arrayList)
arrayList.add(2)
println(arrayList)
}
Output:
[Hi, 1, Welcome, null]
[Hi, 1, Welcome, null, 2]
Use mutablListOf()
to Create a List in Kotlin
The mutableListOf()
library functions similar to the arrayListOf()
library. In fact, mutableListOf()
internally uses the ArrayList
.
Let’s create the same list above using the mutableListOf()
function.
Code:
fun main(args: Array<String>) {
val mutableList = mutableListOf("Hi", 1, "Welcome", null)
println(mutableList)
mutableList.add(2)
println(mutableList)
}
Output:
[Hi, 1, Welcome, null]
[Hi, 1, Welcome, null, 2]
Use listOfNotNull()
Method to Avoid Null
in Kotlin List
If we don’t want a list to have null
values, we can use the listOfNotNull()
function. This will create an immutable list that will not accept a null
value.
Code:
fun main(args: Array<String>) {
val notNullList = listOfNotNull("Hi", null, 1, "Welcome", null)
println(notNullList)
}
Output:
[Hi, 1, Welcome]
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn