How to Filter a List in Kotlin
-
Use the
filter()
Function to Filter a List in Kotlin -
Use the
filterIndexed()
Function to Filter a List in Kotlin -
Use the
filterNot()
Function to Filter a List in Kotlin -
Use the
filterIsInstance()
Function to Filter a List in Kotlin -
Use the
filterNotNull()
Function to Filter a List in Kotlin -
Use the
Partition()
Function to Filter a List in Kotlin
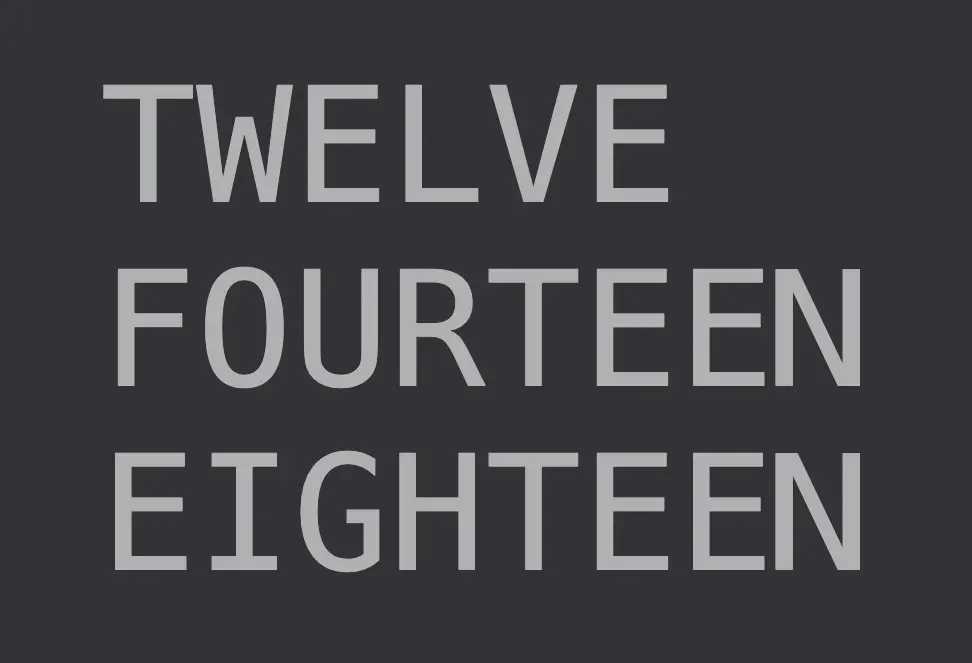
Suppose you have a collection of details of people, and you only want to get people above 20 years old from the entire collection. How will you do that? By filtering!
Filtering is a concept of processing collections in Kotlin. Kotlin allows filtering collections with the help of predicates.
Predicates are lambda functions that take an element of a collection and match it with the function’s output. If the output matches, the predicate returns true
; otherwise, it returns false
.
Kotlin’s standard library has some functions that allow filtering without changing the initial collection. Hence, filtering is possible with both mutable and read-only collections.
We can also use the resultant collection in functions or operations by assigning it to a variable or chaining them after filtering the required results. This article will take you through different functions to use along with predicate expressions for filtering lists in Kotlin.
Use the filter()
Function to Filter a List in Kotlin
The filter()
function is the most basic Standard Library function for filtering. It accepts a predicate expression and returns a collection with the elements that match the predicate’s executed value.
fun main() {
val num = arrayListOf("two", "eleven", "eighteen", "thirty-nine", "fourty-five")
val newNum = num.filter { it.length > 6 }
println(newNum)
}
Output:
Use the filterIndexed()
Function to Filter a List in Kotlin
The Kotlin filter()
function can match the values of the predicate and the element. However, it cannot check the values of elements at specific indexes.
The filterIndexed()
function can check for both value and index of the list element. The predicate expression here takes two arguments: for index and the value.
In this example, we will use the same code as above. But this time, we will only eliminate the element whose length is less than 6 and is at index value 1.
fun main() {
val num = arrayListOf("two", "eleven", "eighteen", "thirty-nine", "fourty-five")
val newNum = num.filterIndexed { index, it -> (index!=1) && (it.length > 6) }
println(newNum)
}
Use the filterNot()
Function to Filter a List in Kotlin
We can also apply negative filters using the filterNot
function for the Kotlin filter. Consider the below example to understand this concept.
The filterNot()
function reverses the filter()
function’s operations. It will return a list with all the elements that will yield false against the predicate.
fun main() {
val num = arrayListOf("two", "eleven", "eighteen", "thirty-nine", "fourty-five")
val newNum = num.filterNot { it.length > 6 }
println(newNum)
}
Use the filterIsInstance()
Function to Filter a List in Kotlin
We have filtered Kotlin lists based on values and indexes until now. The filterIsInstance()
allows us to filter based on elements’ data type.
Here, we will create a list of multiple data types and then filter out only String values from it.
fun main() {
val Str = listOf(12, null, "five", "nine", 11.0)
println("Print only string values from the list:")
Str.filterIsInstance<String>().forEach {
println(it)
}
}
Output:
Use the filterNotNull()
Function to Filter a List in Kotlin
This function filters only non-null elements. All the null
elements will be filtered out when we use the Kotlin filterNotNull()
function.
Here’s an example of creating a list with some null
and non-null values. We will then use the filterNotNull()
function to filter only non-null elements in the new list.
fun main() {
val Num = listOf("twelve", null, null, "fourteen", null, "eighteen")
Num.filterNotNull().forEach {
println(it.uppercase())
}
}
Output:
Use the Partition()
Function to Filter a List in Kotlin
Besides the filter function, Kotlin also has the partition()
function that enables filtering. The primary difference between the two is the number of lists they return.
The filter function returns only one list with the elements that match the predicate. But the partition()
function returns two lists: one with the elements that match the predicate and the other with the elements that don’t match the predicate.
We will create a list and filter all the elements in two separate lists using the partition()
function.
fun main() {
val Num = listOf("eleven", "twelve", "thirteen", "seventeen", "eighteen")
val (matchList, nonMatchList) = Num.partition { it.length < 7 }
println(matchList)
println(nonMatchList)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn