How to Convert List to Map in Kotlin
- Create a Kotlin Project
-
Use the
associate()
Method to Convert List to Map in Kotlin -
Use the
associateBy()
Method to Convert List to Map in Kotlin -
Use the
associateWith()
Method to Convert List to Map in Kotlin -
Use the
groupBy()
Method to Convert List to Map in Kotlin - Conclusion
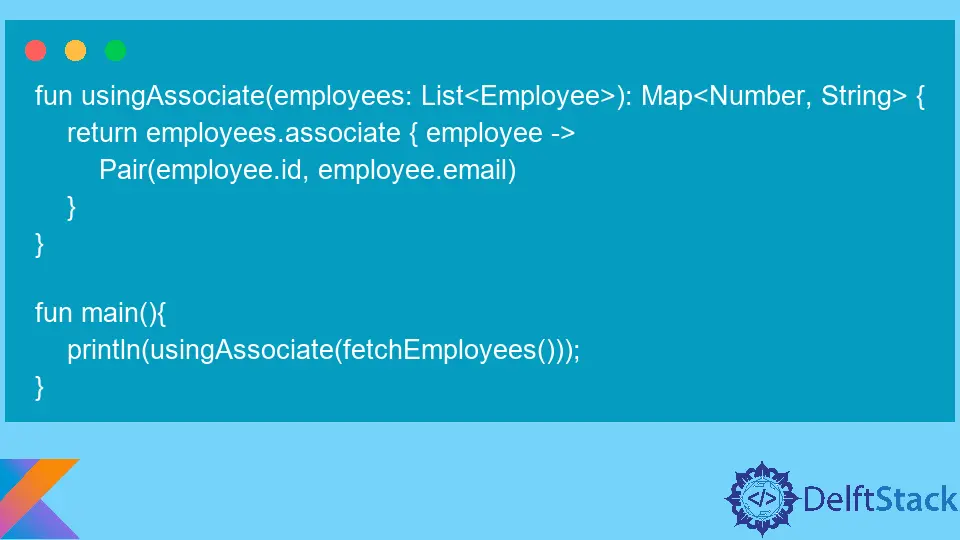
Kotlin provides different collections; the most common ones include List
, Map
, and Set
. When developing applications, there are situations whereby we are required to convert one type of collection to the other to achieve a particular objective.
For example, you might have a list that contains duplicate elements, and we know that a set does not allow duplicate elements. We can convert the list to a set, and the duplicates will be removed without writing any logic.
Kotlin allows us to do these operations by providing different methods that we can use on the collections to convert a collection to a different type of collection.
In this tutorial, we will learn the different ways we can leverage to convert a List data structure to a Map data structure.
Create a Kotlin Project
Open IntelliJ and select New > File> Project
. On the window that opens, enter the project name as list-to-map
, select Kotlin
in the Language section, and IntelliJ
in the Build System section.
Finally, press the Create
button to generate a Kotlin project. Create a Main.kt
file under the kotlin
folder and copy and paste the following code into the file.
data class Employee(val id: Number,
val firstName: String,
val email: String);
fun fetchEmployees(): List<Employee> {
return listOf(
Employee(1,"john", "john@gmail.com"),
Employee(2,"mary","mary@gmail.com"),
Employee(3,"jane","jane@gmail.com"),
Employee(3,"abdul","abdul@gmail.com")
);
}
In this example, we have created a data class
named Employee
which helps us create a List of employees List<Employee>
in the fetchEmployees()
method.
We will use the fetchEmployees()
method in all the examples we will cover in this tutorial.
Use the associate()
Method to Convert List to Map in Kotlin
Copy and paste the following code into the Main.kt
file after the fetchEmployees()
function.
fun usingAssociate(employees: List<Employee>): Map<Number, String> {
return employees.associate { employee ->
Pair(employee.id, employee.email)
}
}
fun main(){
println(usingAssociate(fetchEmployees()));
}
In this example, we have converted our list to a map containing the employee.id
as the key and the employee.email
as the string Map<Number, String>
. Note that the insertion order of our map is maintained as it was in the list.
The associate()
method is an extension function of the Iterable
interface that uses a transformation function on the list elements to create a pair and returns this pair as a map.
Note that we explicitly provided the values we want to return as both key and values in the created pair. If a pair’s key is duplicated, the old pair is replaced with the new pair.
For example, we have two objects in our list with the same id of 3
, the first being that of jane
and the second being that of abdul
. When the elements are inserted in the map, jane
will be replaced by abdul
.
Run the above code and ensure the output is as shown below:
{1=john@gmail.com, 2=mary@gmail.com, 3=abdul@gmail.com}
Use the associateBy()
Method to Convert List to Map in Kotlin
Comment out the previous example, and copy and paste the following code into the Main.kt
file after the comment.
fun usingAssociateBy(employees: List<Employee>): Map<Number, Employee> {
return employees.associateBy { employee: Employee ->
employee.id
}
}
fun main(){
println(usingAssociateBy(fetchEmployees()));
}
In this example, we have converted a list to a Map that contains the employee.id
as the key, and the employee object has the value Map<Number, Employee>
.
The associateBy()
method is also an extension function of the Iterable
interface. We only need to pass the value used as the key in our map.
The keySelector
function gets this key from each object in the list and applies it to the map. The values of the map become the objects in our list.
Note that the keySelector
function will retrieve two duplicates with value 3
, as we have seen above. The old element will get replaced by the new element.
Run this code and ensure the output is as shown below:
{1=Employee(id=1, firstName=john, email=john@gmail.com),
2=Employee(id=2, firstName=mary, email=mary@gmail.com),
3=Employee(id=3, firstName=abdul, email=abdul@gmail.com)}
Use the associateWith()
Method to Convert List to Map in Kotlin
Comment out the previous example, and copy and paste the following code into the Main.kt
file after the comment.
fun usingAssociateWith(employees: List<Employee>): Map<Employee, Number> {
return employees.associateWith { employee ->
employee.id
}
}
fun main(){
println(usingAssociateWith(fetchEmployees()));
}
In this example, we have converted a list into a map that contains the employee object as the key and the employee.id
as the value Map<Employee, Number>
.
The associateWith()
method is an extension function of the Iterable
interface, and we only need to pass the element that will be used as the value in our map. The valueSelector
function gets the values from each object in our list and applies them to our map.
The keys of the map become the elements in our list. Since the objects are keys in the map, we will not have duplicates, but two elements in our map will have the value 3
.
Run this code and ensure the output is as shown below:
{Employee(id=1, firstName=john, email=john@gmail.com)=1,
Employee(id=2, firstName=mary, email=mary@gmail.com)=2,
Employee(id=3, firstName=jane, email=jane@gmail.com)=3,
Employee(id=3, firstName=abdul, email=abdul@gmail.com)=3}
Use the groupBy()
Method to Convert List to Map in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after the comment.
fun usingGroupBy(employees: List<Employee>): Map<Number, List<Employee>> {
return employees.groupBy { employee: Employee ->
employee.id
}
}
fun main(){
println(usingGroupBy(fetchEmployees()));
}
In our first examples, we have seen that any duplicates get replaced when converting a list to a map. In situations where you want to maintain the duplicates, we use the groupBy()
method.
In this example, we have converted a list to a map that contains the key as the employee.id
, and the values are a list of employee objects grouped by their id Map<Number, List<Employee>>
.
The groupBy()
method is also an extension function of the Iterable
interface and only requires us to pass the value used as the key in our map. This method’s keySelector
function gets the key from each object in the list and applies it to our map.
Objects that have the same id are grouped in one list. Run this code and ensure the output is as shown below.
{1=[Employee(id=1, firstName=john, email=john@gmail.com)],
2=[Employee(id=2, firstName=mary, email=mary@gmail.com)],
3=[Employee(id=3, firstName=jane, email=jane@gmail.com),
Employee(id=3, firstName=abdul, email=abdul@gmail.com)]}
Conclusion
In this tutorial, we have learned how to convert a list to a map by leveraging different extension functions of the Iterable
interface. The methods we have covered include using the associate()
method, the associateBy()
method, the associateWith()
method, and the groupBy()
method.
Note that there are other grouping strategies that you can use, such as groupingBy()
and groupByTo()
.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub