map() vs each() Functions in jQuery
-
jQuery
map()
Function -
jQuery
each()
Function -
Differences Between the
map()
andeach()
Methods in jQuery
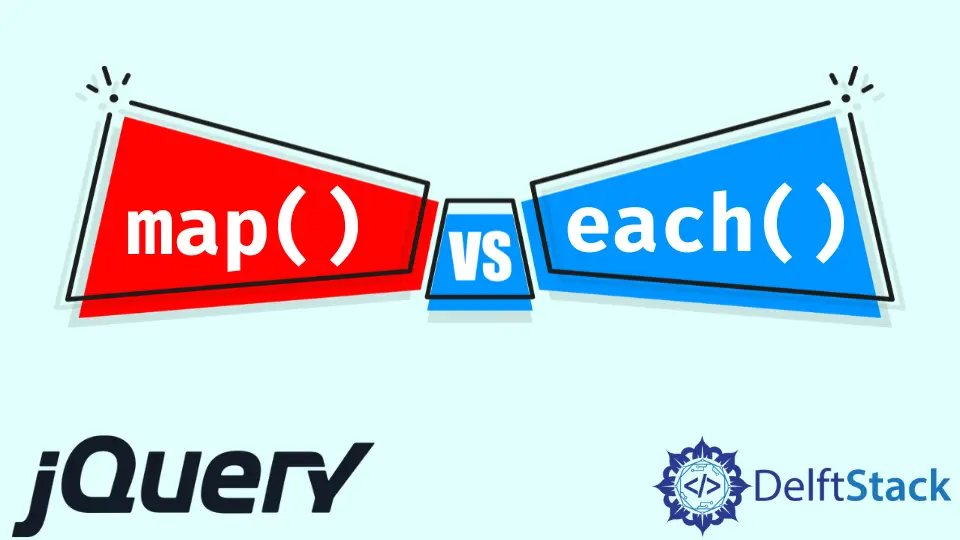
The map()
method in jQuery can translate all items of an array object to another array of items. On the other hand, the each()
method specifies a function to run for each matched element.
This tutorial demonstrates how to use the map()
and each()
methods in jQuery.
jQuery map()
Function
The map()
method can translate items of one array or object to a new array. The syntax for the map()
method is:
map( array/object, callback )
Where:
- The
array/object
is the one that will be translated. - The
callback
is the function on which basis an array will be translated.
The syntax for the callback function can be:
Function( Object elementOfArray, Integer indexInArray ) => object
Here, the first argument is the element of the array, and the second argument is the index of the array. This callback function can return any value.
It will return a flattened array. =>
refers to this
, and => object
is the global object.
Let’s try an example that will add 10 to each member of an integer array using the map()
method:
<!DOCTYPE html>
<html>
<head>
<title> jQuery map() Function Demo </title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
</head>
<body>
<h1> Welcome to DELFTSTACK </h1>
<h3> This is an example to use jQuery map() method </h3>
<p> <b> The original array before using map method is: </b> [100, 110, 120, 130, 140, 150] </p>
<p> Click Button to run the Map() method: </p>
<button> Click here </button>
<p id = "para"> </p>
<script>
$(document).ready(function(){
$("button").click(function(){
var Original_Array = [100, 110, 120, 130, 140, 150];
var New_Array = jQuery.map(Original_Array, function(add_number) {
return add_number + 10;
});
document.getElementById('para').innerHTML = "<b> The new array after using map() is: </b>" + JSON.stringify(New_Array);
});
});
</script>
</body>
</html>
The code above will add 10 to each member of the given array. See output:
The map()
method doesn’t only work with the integer array but can also work with any type of array. Let’s try another example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title> jQuery map() Function Demo </title>
<script src="https://code.jquery.com/jquery-3.4.1.js">
</script>
</head>
<body style="text-align:center;">
<h1 style="color: blue">
This is DELFSTACK
</h1>
<h3>JQuery map() Function Demo</h3>
<b>String = "delftstack.com"</b>
<br> <br>
<button onclick="delftstack()">Click Here</button>
<br> <br>
<b id="b_id"></b>
<script>
function delftstack() {
var Text_Place = document.getElementById('b_id');
var Demo_String = "delftstack.com";
Demo_String = Demo_String.split("");
// Here map method will create a new array my concatenating each character from the string with D
var New_String = jQuery.map(Demo_String, function(Array_Item) {
return Array_Item + 'D ';
})
Text_Place.innerHTML = New_String;
}
</script>
</body>
</html>
The code above will split the given string and convert it to an array of each character concatenated with a character D
. See output:
jQuery each()
Function
The each()
method is a generic iterator function used to iterate over arrays and objects. These arrays and similar objects are iterated by numeric index and the other with the named properties.
The syntax for each()
is:
each( array/object, callback )
Where:
- The
array/object
is the array or object to iterate over. - The
callback
function is the function that will be executed on every value.
The $(selector).each()
and $.each()
functions are not similar. The $(selector).each()
function is used iterate only over a jQuery object.
On the other hand, the $.each()
function is used to iterate over any collection, no matter if it is an array or an object. Let’s try an example for the each()
method:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery.each demo</title>
<style>
div {
color: green;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="Delftstack1"></div>
<div id="Delftstack2"></div>
<div id="Delftstack3"></div>
<div id="Delftstack4"></div>
<div id="Delftstack5"></div>
<script>
var Demo_Array = [ "Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5" ];
var Demo_Object = { Delftstack1: 10, Delftstack2: 20, Delftstack3: 30, Delftstack4: 40, Delftstack5: 50 };
jQuery.each( Demo_Array, function( x, Delft_Value ) {
$( "#" + Delft_Value ).text( "The value is " + Delft_Value + "." );
});
jQuery.each( Demo_Object, function( x, Delft_Value ) {
$( "#" + x ).append( document.createTextNode( " : " + Delft_Value ) );
});
</script>
</body>
</html>
The code above will print values from an array and an object together simultaneously. See output:
The value is Delftstack1. : 10
The value is Delftstack2. : 20
The value is Delftstack3. : 30
The value is Delftstack4. : 40
The value is Delftstack5. : 50
Differences Between the map()
and each()
Methods in jQuery
There are several differences between the map()
and each()
methods:
- The
map()
method can be used as an iterator, but its real use is manipulating the array and returning the new one. On the other hand, theeach()
method is an immutable iterator. - Both functions take the callback function as a parameter. The parameters of the callback functions have different positions.
- In
map()
, the callback function isfunction(element, index){}
, and ineach()
, the callback function isfunction(index, element){}
. If we change the order of the parameters in the callback function, the methods will not work properly. - The
map()
method will perform some operations on an array and return a new array. While theeach()
method can be used to perform a particular action and will still return the original array. - The
this
keyword in themap()
method denotes the current window object, while thethis
keyword in theeach()
method denotes the current element. - There is no way to terminate an iteration in the
map()
method, while in theeach
method, we can terminate an iteration.
Both methods are useful in jQuery based on the use case of the user. If we want to create a new array for the other methods like filter()
and reduce()
, we can use the map()
method.
While if we only want to perform some action on the elements, then the each()
method can be used.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook