How to Use setTimeout() in jQuery
- Understanding setTimeout in jQuery
- Basic Usage of setTimeout
- Chaining setTimeout Calls
- Canceling a setTimeout Call
- Conclusion
- FAQ
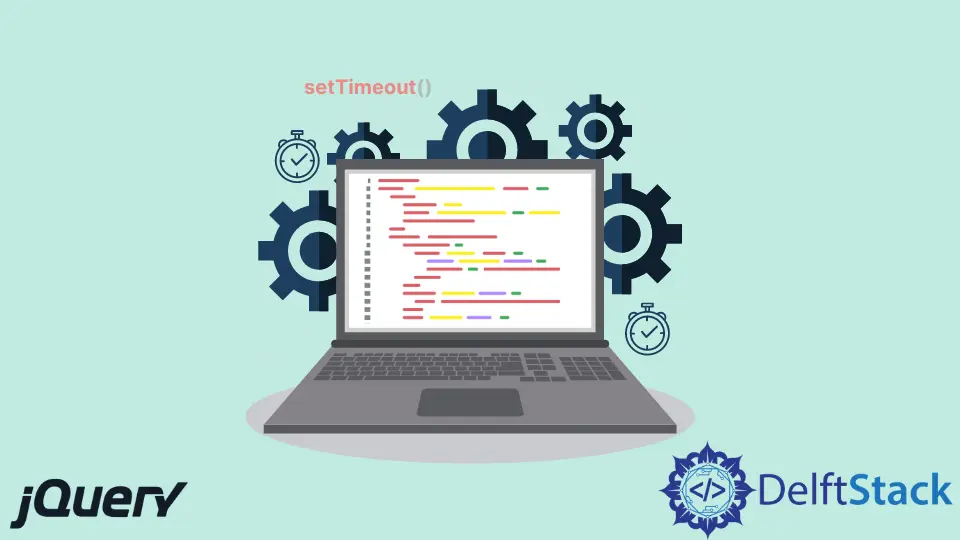
In today’s post, we’ll learn about the setTimeout function in jQuery. This powerful feature is essential for managing asynchronous operations in web development. Whether you’re looking to delay an action, create animations, or manage user interactions, understanding how to use setTimeout can significantly enhance your jQuery projects. By the end of this article, you’ll have a solid grasp of how to implement this function effectively, along with practical examples that illustrate its use in real-world scenarios. Let’s dive in!
Understanding setTimeout in jQuery
setTimeout is a built-in JavaScript function that allows you to execute a piece of code after a specified delay. While jQuery doesn’t provide its own version of setTimeout, it works seamlessly with this JavaScript function. The syntax is straightforward:
setTimeout(function, milliseconds);
Here, the function
is the code you want to execute after the delay, and milliseconds
is how long to wait before executing the function. This can be particularly useful for creating timed animations, delaying user notifications, or managing time-sensitive interactions in your web applications.
Basic Usage of setTimeout
To illustrate the basic usage of setTimeout in jQuery, let’s take a look at a simple example where we change the text of a paragraph after a delay.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setTimeout Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p id="message">Hello, World!</p>
<button id="changeText">Change Text After 3 Seconds</button>
<script>
$("#changeText").click(function() {
setTimeout(function() {
$("#message").text("Text changed after 3 seconds!");
}, 3000);
});
</script>
</body>
</html>
In this example, when the user clicks the button, the text in the paragraph will change after a delay of three seconds. The setTimeout function takes two arguments: the function to execute and the delay in milliseconds. Here, we’re using jQuery to select the paragraph and change its text.
This simple application demonstrates how you can use setTimeout to delay actions in your jQuery projects. It enhances user experience by providing a smooth transition between states.
Chaining setTimeout Calls
One of the powerful features of setTimeout is that you can chain multiple calls together to create a sequence of actions. This can be particularly useful for animations or timed events. Let’s explore how to implement this.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chaining setTimeout</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<div id="box" style="width:100px; height:100px; background-color:red;"></div>
<script>
$("#box").css("position", "relative");
setTimeout(function() {
$("#box").animate({left: '+=100px'}, 1000);
}, 1000);
setTimeout(function() {
$("#box").animate({top: '+=100px'}, 1000);
}, 2000);
setTimeout(function() {
$("#box").animate({left: '-=100px'}, 1000);
}, 3000);
setTimeout(function() {
$("#box").animate({top: '-=100px'}, 1000);
}, 4000);
</script>
</body>
</html>
In this example, we animate a red box to move right, down, left, and up in sequence. Each setTimeout call is set to trigger after a certain delay, creating a smooth animation effect.
Chaining setTimeout calls allows you to create complex animations or interactions without overwhelming the user. This technique is especially beneficial when you want to maintain a clear sequence of events in your web applications.
Canceling a setTimeout Call
Sometimes, you might want to cancel a scheduled setTimeout call before it executes. This can be useful in scenarios where user interactions change the flow of your application. To cancel a timeout, you can use the clearTimeout function. Here’s how you can do it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Canceling setTimeout</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p id="message">Waiting for the text to change...</p>
<button id="start">Start Timer</button>
<button id="cancel">Cancel Timer</button>
<script>
let timer;
$("#start").click(function() {
timer = setTimeout(function() {
$("#message").text("Text changed after 3 seconds!");
}, 3000);
});
$("#cancel").click(function() {
clearTimeout(timer);
$("#message").text("Timer canceled!");
});
</script>
</body>
</html>
In this example, we have two buttons: one to start the timer and another to cancel it. When the user clicks “Start Timer,” the setTimeout function is initiated. However, if they click “Cancel Timer” before the three seconds are up, the timer is canceled, and a message is displayed.
This functionality is crucial in creating responsive web applications. It allows you to manage user interactions effectively and avoid executing code that is no longer relevant.
Conclusion
In summary, the setTimeout function is a versatile tool in jQuery that allows developers to control the timing of code execution. By mastering its use, you can enhance your web applications with smooth animations, timed interactions, and responsive user experiences. Whether you’re chaining multiple calls for complex animations or canceling timers based on user actions, setTimeout provides the flexibility needed to create dynamic web applications. Start experimenting with setTimeout today, and see how it can elevate your jQuery projects!
FAQ
-
What is setTimeout in jQuery?
setTimeout is a JavaScript function used to execute code after a specified delay. It can be used in jQuery to manage timed events and animations. -
Can I cancel a setTimeout call?
Yes, you can cancel a scheduled setTimeout call using the clearTimeout function. -
How do I chain multiple setTimeout calls?
You can chain setTimeout calls by placing them sequentially with increasing delay times, allowing for complex animations or timed actions. -
Is setTimeout specific to jQuery?
No, setTimeout is a standard JavaScript function and can be used in any JavaScript code, including jQuery. -
How does setTimeout improve user experience?
By allowing you to delay actions or create timed animations, setTimeout can make web applications feel more responsive and engaging.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn