jQuery keyup
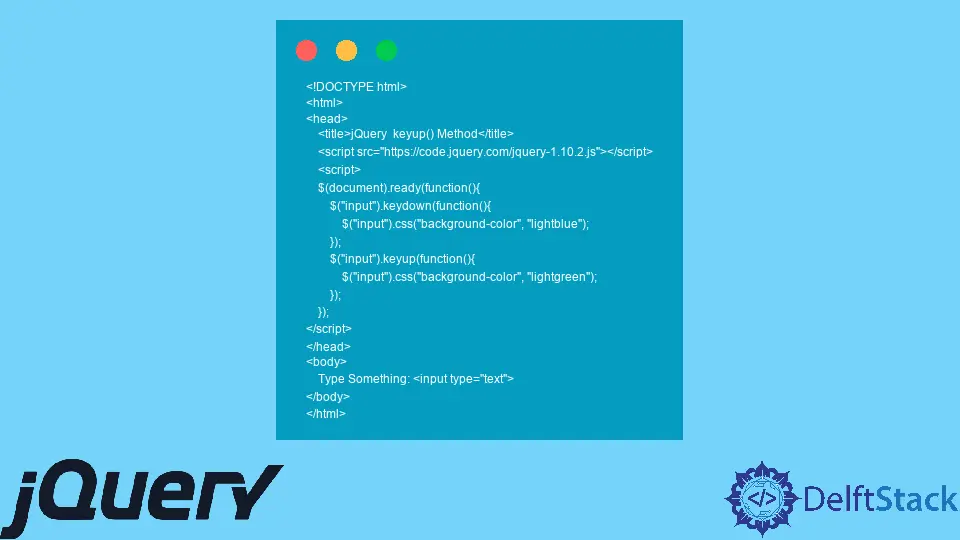
The keyup()
method in jQuery triggers the keyup event of JavaScript whenever a key is released from the keyboard.
This tutorial demonstrates how to use the keyup()
method in jQuery.
jQuery Keyup
The keyup()
method will trigger the keyup event when a key is released from the keyboard. The method is used to detect if any key is released from the keyboard.
The syntax for this method is below.
$(selector).keyup(function)
Where the selector
can be the id, class, or element name, and the function
is an optional parameter that gives us an idea if the key is pressed or not. The return value for this method is whether the key is pressed or not.
It will change the background color according to it. Let’s try an example for the keyup()
method.
<!DOCTYPE html>
<html>
<head>
<title>jQuery keyup() Method</title>
<script src="https://code.jquery.com/jquery-1.10.2.js"></script>
<script>
$(document).ready(function(){
$("input").keydown(function(){
$("input").css("background-color", "lightblue");
});
$("input").keyup(function(){
$("input").css("background-color", "lightgreen");
});
});
</script>
</head>
<body>
Type Something: <input type="text">
</body>
</html>
The code above will change the background color of the input field to light green when the key is released and light blue when the key is pressed. See output:
Let’s try another example that will change the background color of the div
every time the key is released. See example:
<html>
<head>
<title>jQuery keyup() Method</title>
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<style>
div {
width: 700px;
height: 500px;
padding: 30px;
font-size: large;
text-align: center;
margin: auto;
font-weight: bold;
border: 5px solid cornflowerblue;
margin-top: 50px;
margin-bottom: 20px;
}
</style>
</head>
<script>
var colors = ["lightblue", "lightgreen", "violet", "lightpink", "red", "forestgreen", "white", "indigo"];
var i = 0;
$(document).keyup(function(event) {
$("div").css("background-color", colors[i]);
i++;
i = i % 9;
});
</script>
<body>
<br />
<br />
<div>
<h1 style="color:teal; font-size:x-large; font-weight: bold;"> jQuery keyup event </h1>
<p style="color:maroon; font-size: large;">
Press and release the space or any other key <br /> The background of the div will change </p>
</div>
</body>
</html>
See the output for code above:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook