jQuery의 map() 대 each() 함수
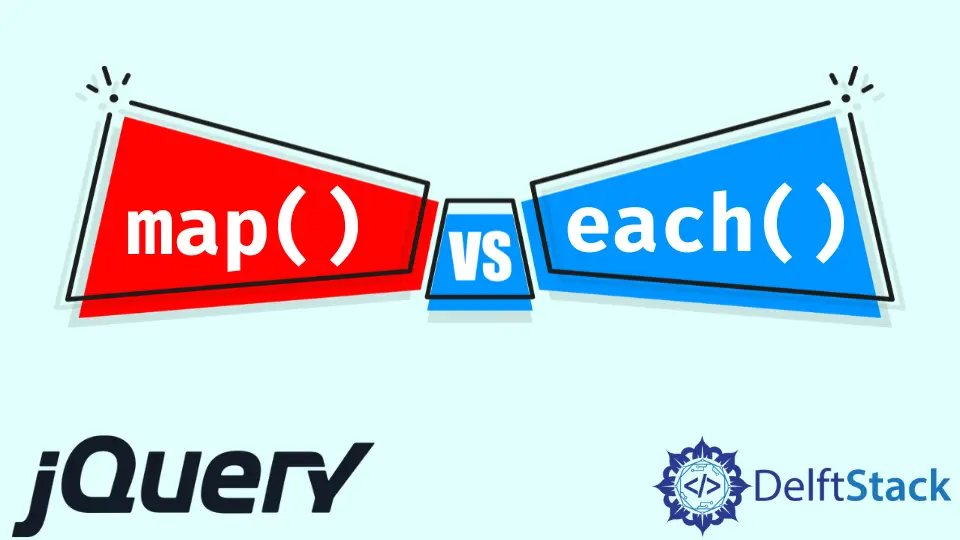
jQuery의 map()
메서드는 배열 객체의 모든 항목을 다른 항목 배열로 변환할 수 있습니다. 반면에 each()
메서드는 일치하는 각 요소에 대해 실행할 함수를 지정합니다.
이 튜토리얼은 jQuery에서 map()
및 each()
메소드를 사용하는 방법을 보여줍니다.
jQuery map()
함수
map()
메서드는 하나의 배열 또는 개체의 항목을 새 배열로 변환할 수 있습니다. map()
메서드의 구문은 다음과 같습니다.
map( array/object, callback )
어디에:
array/object
는 번역될 것입니다.콜백
은 배열이 번역될 기반이 되는 기능입니다.
콜백 함수의 구문은 다음과 같습니다.
Function( Object elementOfArray, Integer indexInArray ) => object
여기서 첫 번째 인수는 배열의 요소이고 두 번째 인수는 배열의 인덱스입니다. 이 콜백 함수는 모든 값을 반환할 수 있습니다.
평평한 배열을 반환합니다. =>
는 this
를 나타내고 => object
는 전역 개체입니다.
map()
메서드를 사용하여 정수 배열의 각 구성원에 10을 추가하는 예를 시도해 보겠습니다.
<!DOCTYPE html>
<html>
<head>
<title> jQuery map() Function Demo </title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
</head>
<body>
<h1> Welcome to DELFTSTACK </h1>
<h3> This is an example to use jQuery map() method </h3>
<p> <b> The original array before using map method is: </b> [100, 110, 120, 130, 140, 150] </p>
<p> Click Button to run the Map() method: </p>
<button> Click here </button>
<p id = "para"> </p>
<script>
$(document).ready(function(){
$("button").click(function(){
var Original_Array = [100, 110, 120, 130, 140, 150];
var New_Array = jQuery.map(Original_Array, function(add_number) {
return add_number + 10;
});
document.getElementById('para').innerHTML = "<b> The new array after using map() is: </b>" + JSON.stringify(New_Array);
});
});
</script>
</body>
</html>
위의 코드는 주어진 배열의 각 구성원에 10을 추가합니다. 출력 참조:
map()
메서드는 정수 배열과 함께 작동할 뿐만 아니라 모든 유형의 배열에서도 작동할 수 있습니다. 다른 예를 시도해 보겠습니다.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title> jQuery map() Function Demo </title>
<script src="https://code.jquery.com/jquery-3.4.1.js">
</script>
</head>
<body style="text-align:center;">
<h1 style="color: blue">
This is DELFSTACK
</h1>
<h3>JQuery map() Function Demo</h3>
<b>String = "delftstack.com"</b>
<br> <br>
<button onclick="delftstack()">Click Here</button>
<br> <br>
<b id="b_id"></b>
<script>
function delftstack() {
var Text_Place = document.getElementById('b_id');
var Demo_String = "delftstack.com";
Demo_String = Demo_String.split("");
// Here map method will create a new array my concatenating each character from the string with D
var New_String = jQuery.map(Demo_String, function(Array_Item) {
return Array_Item + 'D ';
})
Text_Place.innerHTML = New_String;
}
</script>
</body>
</html>
위의 코드는 주어진 문자열을 분할하고 문자 D
와 연결된 각 문자의 배열로 변환합니다. 출력 참조:
jQuery each()
함수
each()
메서드는 배열과 객체를 반복하는 데 사용되는 일반적인 반복기 함수입니다. 이러한 배열 및 유사한 개체는 숫자 인덱스로 반복되고 다른 하나는 명명된 속성으로 반복됩니다.
each()
의 구문은 다음과 같습니다.
each( array/object, callback )
어디에:
array/object
는 반복할 배열 또는 개체입니다.callback
기능은 모든 값에 대해 실행될 기능입니다.
$(selector).each()
및 $.each()
함수는 유사하지 않습니다. $(selector).each()
함수는 jQuery 객체에 대해서만 반복하는 데 사용됩니다.
반면에 $.each()
함수는 배열이든 객체이든 상관없이 모든 컬렉션을 반복하는 데 사용됩니다. each()
메서드의 예를 살펴보겠습니다.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery.each demo</title>
<style>
div {
color: green;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="Delftstack1"></div>
<div id="Delftstack2"></div>
<div id="Delftstack3"></div>
<div id="Delftstack4"></div>
<div id="Delftstack5"></div>
<script>
var Demo_Array = [ "Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5" ];
var Demo_Object = { Delftstack1: 10, Delftstack2: 20, Delftstack3: 30, Delftstack4: 40, Delftstack5: 50 };
jQuery.each( Demo_Array, function( x, Delft_Value ) {
$( "#" + Delft_Value ).text( "The value is " + Delft_Value + "." );
});
jQuery.each( Demo_Object, function( x, Delft_Value ) {
$( "#" + x ).append( document.createTextNode( " : " + Delft_Value ) );
});
</script>
</body>
</html>
위의 코드는 배열과 객체의 값을 동시에 인쇄합니다. 출력 참조:
The value is Delftstack1. : 10
The value is Delftstack2. : 20
The value is Delftstack3. : 30
The value is Delftstack4. : 40
The value is Delftstack5. : 50
jQuery의 map()
과 each()
메소드의 차이점
map()
과 each()
메서드에는 몇 가지 차이점이 있습니다.
map()
메서드는 반복자로 사용할 수 있지만 실제 용도는 배열을 조작하고 새 배열을 반환하는 것입니다. 반면each()
메서드는 변경할 수 없는 반복자입니다.- 두 함수 모두 콜백 함수를 매개변수로 받습니다. 콜백 함수의 매개변수는 위치가 다릅니다.
map()
에서 콜백 함수는function(element, index){}
이고each()
에서 콜백 함수는function(index, element){}
입니다. 콜백 함수에서 매개변수의 순서를 변경하면 메서드가 제대로 작동하지 않습니다.map()
메서드는 배열에서 일부 작업을 수행하고 새 배열을 반환합니다.each()
메서드는 특정 작업을 수행하는 데 사용할 수 있지만 여전히 원래 배열을 반환합니다.map()
메서드의this
키워드는 현재 창 개체를 나타내는 반면each()
메서드의this
키워드는 현재 요소를 나타냅니다.map()
메서드에서는 반복을 종료할 수 있는 방법이 없지만each
메서드에서는 반복을 종료할 수 있습니다.
두 방법 모두 사용자의 사용 사례에 따라 jQuery에서 유용합니다. filter()
및 reduce()
와 같은 다른 메서드에 대한 새 배열을 생성하려면 map()
메서드를 사용할 수 있습니다.
요소에 대해 일부 작업만 수행하려는 경우 each()
메서드를 사용할 수 있습니다.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook