How to Use the for Loop in jQuery
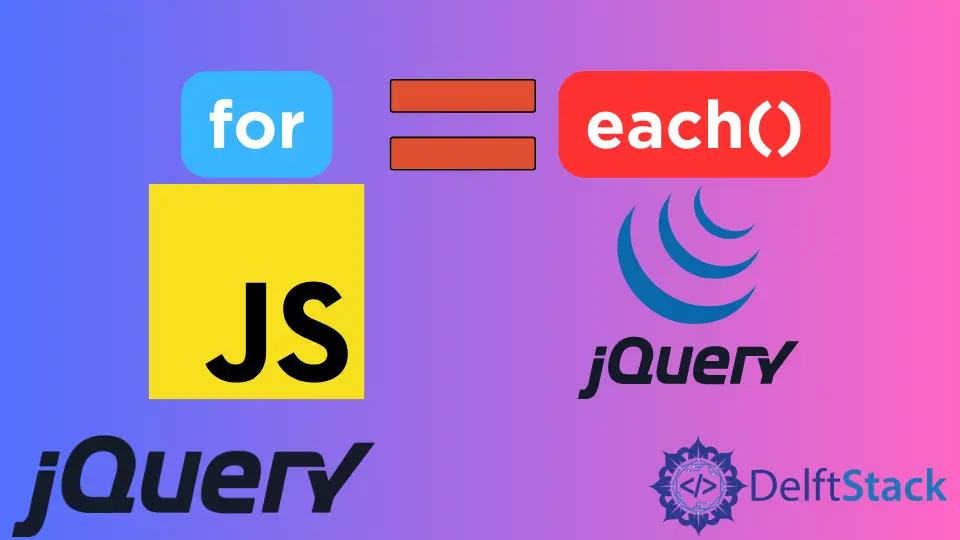
Though the jQuery supports the for
loop, it is the functionality of core JavaScript. jQuery has a method, each()
, which can iterate through arrays and objects both.
This tutorial demonstrates how to use the for
loop in jQuery.
Use the for
Loop in jQuery
The each()
method in jQuery works similarly to JavaScript’s for
loop. The each()
method can iterate through arrays and objects.
While, to iterate through objects in JavaScript, we need to use the for in
Loop. Let’s see an example for the for
loop from JavaScript:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript For Loop</h2>
<p id="DemoPara"></p>
<script>
const Demo_Array = ["Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"];
let Demo_String = "";
for (let i = 0; i < Demo_Array.length; i++) {
Demo_String += Demo_Array[i] + "<br>";
}
document.getElementById("DemoPara").innerHTML = Demo_String;
</script>
</body>
</html>
The code above will print the array members using JavaScript’s for
loop. See output:
JavaScript For Loop
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Delftstack6
The each()
method can also perform this operation for both arrays and objects. The syntax for the each()
method is:
.each( function )
The function
will be executed for each matched element, and the each()
method is used to make the DOM looping construct less error-prone. It will iterate through the DOM elements.
Let’s try examples for each()
method in jQuery. Here is a similar example to JavaScript’s for
loop with jQuery’s each()
method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each() Method</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
var Demo_Array = ["Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"];
$.each(Demo_Array, function(index, value){
$("#DemoDiv").append(value + '<br>');
});
});
</script>
</head>
<body>
<h2>JQuery Each() Method</h2>
<div id="DemoDiv"></div>
</body>
</html>
The output for the code above is:
JQuery Each() Method
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Delftstack6
Here is another example for the each()
method that will iterate over the div
elements. See example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each() Method</title>
<style>
div {
color: black;
text-align: center;
cursor: pointer;
font-weight: bolder;
width: 500px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div>Hello, This is Delftstack</div>
<div>Demo to Iterate Over Divs</div>
<div>Click here to iterate over Divs</div>
<div>Www.delftstack.com</div>
<script>
$( document.body ).click(function() {
$( "div" ).each(function( x ) {
if ( this.style.color !== "lightblue" ) {
this.style.color = "lightblue";
}
else {
this.style.color = "green";
}
});
});
</script>
</body>
</html>
The code above will iterate through divs
and change their color on click. See output:
What if we want to stop the iteration at some point in the loop? That is also possible with the jQuery each()
method.
We can just return false
at the particular iteration. See example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each Method</title>
<style>
div {
width: 50px;
height: 50px;
margin: 10px;
float: left;
border: 4px blue solid;
text-align: center;
}
span {
color: red;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div id="StopChanging"></div>
<div></div>
<div></div>
<div></div>
<h2></h2>
<button>Click here to Change Colors</button>
<script>
$( "button" ).click(function() {
$( "div" ).each(function( DivIndex, DivElement ) {
$( DivElement ).css( "backgroundColor", "lightblue" );
if ( $( this ).is( "#StopChanging" ) ) {
$( "h2" ).text( "Stopped at div number #" + DivIndex );
$( "#StopChanging" ).text( "STOP" );
return false;
}
});
});
</script>
</body>
</html>
The example code above will change the background color for all the div
until a particular div
with ID comes in the iteration. It will stop at the given iteration.
See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook