jQuery에서 for 루프 사용
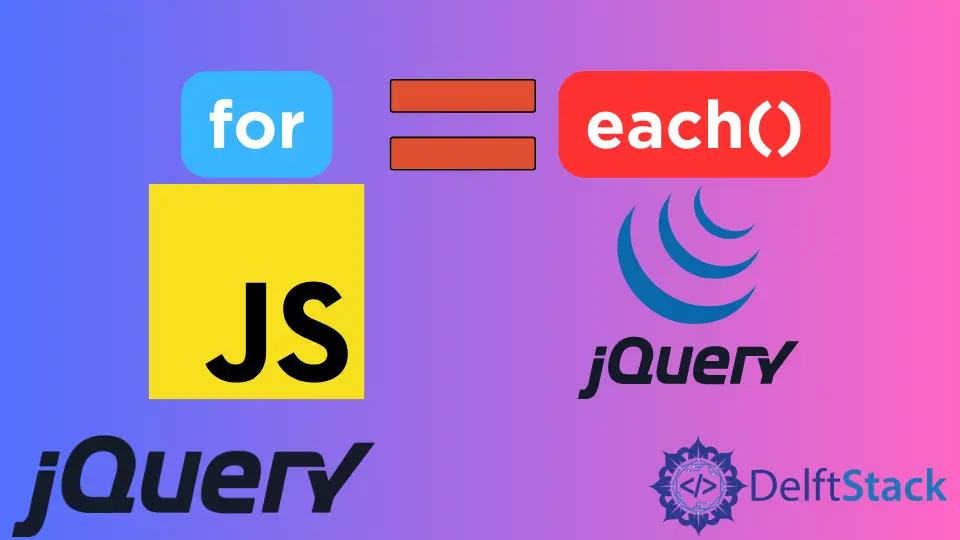
jQuery는 for
루프를 지원하지만 핵심 JavaScript의 기능입니다. jQuery에는 배열과 객체를 모두 반복할 수 있는 each()
메서드가 있습니다.
이 튜토리얼은 jQuery에서 for
루프를 사용하는 방법을 보여줍니다.
jQuery에서 for
루프 사용
jQuery의 each()
메소드는 JavaScript의 for
루프와 유사하게 작동합니다. each()
메소드는 배열과 객체를 반복할 수 있습니다.
while, JavaScript에서 객체를 반복하려면 for in
루프를 사용해야 합니다. JavaScript의 for
루프에 대한 예를 살펴보겠습니다.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript For Loop</h2>
<p id="DemoPara"></p>
<script>
const Demo_Array = ["Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"];
let Demo_String = "";
for (let i = 0; i < Demo_Array.length; i++) {
Demo_String += Demo_Array[i] + "<br>";
}
document.getElementById("DemoPara").innerHTML = Demo_String;
</script>
</body>
</html>
위의 코드는 JavaScript의 for
루프를 사용하여 배열 멤버를 인쇄합니다. 출력 참조:
JavaScript For Loop
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Delftstack6
each()
메소드는 배열과 객체 모두에 대해 이 작업을 수행할 수도 있습니다. each()
메서드의 구문은 다음과 같습니다.
.each( function )
function
은 일치하는 각 요소에 대해 실행되고 each()
메소드는 DOM 루핑 구조를 오류가 덜 발생하도록 만드는 데 사용됩니다. DOM 요소를 반복합니다.
jQuery에서 each()
메소드에 대한 예제를 시도해보자. 다음은 jQuery의 each()
메서드를 사용하는 JavaScript의 for
루프와 유사한 예입니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each() Method</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
var Demo_Array = ["Delftstack1", "Delftstack2", "Delftstack3", "Delftstack4", "Delftstack5", "Delftstack6"];
$.each(Demo_Array, function(index, value){
$("#DemoDiv").append(value + '<br>');
});
});
</script>
</head>
<body>
<h2>JQuery Each() Method</h2>
<div id="DemoDiv"></div>
</body>
</html>
위 코드의 출력은 다음과 같습니다.
JQuery Each() Method
Delftstack1
Delftstack2
Delftstack3
Delftstack4
Delftstack5
Delftstack6
다음은 div
요소를 반복하는 each()
메서드의 또 다른 예입니다. 예를 참조하십시오.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each() Method</title>
<style>
div {
color: black;
text-align: center;
cursor: pointer;
font-weight: bolder;
width: 500px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div>Hello, This is Delftstack</div>
<div>Demo to Iterate Over Divs</div>
<div>Click here to iterate over Divs</div>
<div>Www.delftstack.com</div>
<script>
$( document.body ).click(function() {
$( "div" ).each(function( x ) {
if ( this.style.color !== "lightblue" ) {
this.style.color = "lightblue";
}
else {
this.style.color = "green";
}
});
});
</script>
</body>
</html>
위의 코드는 div
를 반복하고 클릭 시 색상을 변경합니다. 출력 참조:
루프의 특정 지점에서 반복을 중지하려면 어떻게 해야 합니까? 이는 jQuery each()
메서드로도 가능합니다.
특정 반복에서 false
를 반환할 수 있습니다. 예를 참조하십시오.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Each Method</title>
<style>
div {
width: 50px;
height: 50px;
margin: 10px;
float: left;
border: 4px blue solid;
text-align: center;
}
span {
color: red;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div id="StopChanging"></div>
<div></div>
<div></div>
<div></div>
<h2></h2>
<button>Click here to Change Colors</button>
<script>
$( "button" ).click(function() {
$( "div" ).each(function( DivIndex, DivElement ) {
$( DivElement ).css( "backgroundColor", "lightblue" );
if ( $( this ).is( "#StopChanging" ) ) {
$( "h2" ).text( "Stopped at div number #" + DivIndex );
$( "#StopChanging" ).text( "STOP" );
return false;
}
});
});
</script>
</body>
</html>
위의 예제 코드는 ID가 있는 특정 div
가 반복될 때까지 모든 div
의 배경색을 변경합니다. 주어진 반복에서 멈출 것입니다.
출력 참조:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook