How to Loop Through Elements in jQuery
-
Use the
.each()
Method to Loop Through List Elements -
Use the
.each()
Method to Count Table Data With Same Class
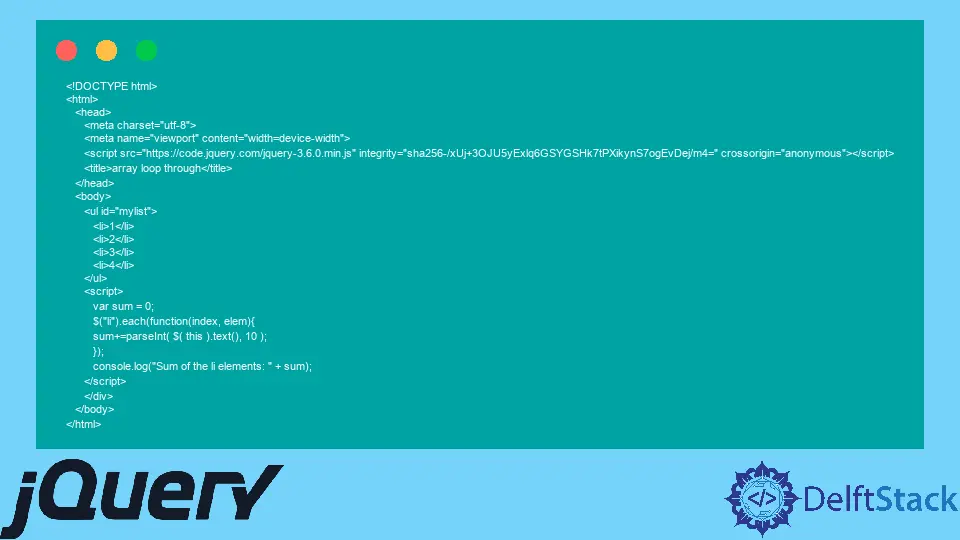
To loop through HTML elements, we use .each()
method. In the initial of this function, we select the element tree that is needed to be traversed.
And in the parenthesis of the method, we add up a function having parameters, the index and the value.
In this process, the value we get is mostly in the text()
format, aka string. If we are to deal with any numeric list, it will be fetched as a string, and later, based upon preference, we can convert it.
In the following examples, we will visualize a similar instance that clarifies the concept of the .each()
method of iterating elements.
Use the .each()
Method to Loop Through List Elements
Here, we will set an example where we have a stack of lists. And focusing on the li
, we will perform iteration.
So, just before the .each()
method, we will set the looping element’s name. And later add up a function that takes the account to hold the index and values.
Let’s see how it works.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>array loop through</title>
</head>
<body>
<ul id="mylist">
<li>1</li>
<li>2</li>
<li>3</li>
<li>4</li>
</ul>
<script>
var sum = 0;
$("li").each(function(index, elem){
sum+=parseInt( $( this ).text(), 10 );
});
console.log("Sum of the li elements: " + sum);
</script>
</div>
</body>
</html>
Output:
In this example, we have grabbed the instances of the li
and used .each()
. The index
and the elem
parameters will store the index numbers and the HTML object detail for each element.
So, it will be cumbersome to deal with the elem
. So we retrieved the li
content as text(string)
and later parsed it into an integer.
We will see another example that will define how the indexes are working.
Use the .each()
Method to Count Table Data With Same Class
As we already know the functionality of the .each()
method, here we will jump directly to the code segment for visualizing the results.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>array loop through</title>
</head>
<body>
<table class="tbl" border=2>
<tr>
<td>D1</td>
<td>D2</td>
</tr>
<tr>
<td>D3</td>
<td>D4</td>
</tr>
</table>
<script>
var cnt = 0;
var ar = []
$("td").each(function(index, elem){
$(elem).addClass('table');
});
$(".table").each(function(index, elem){
ar[cnt]=index;
cnt++;
});
console.log("Total data count: " + cnt);
console.log("The indexes: " + ar);
</script>
</div>
</body>
</html>
Output:
So, the index
parameter is acquiring the numeric values, and thus we get the number of table data and the representation in an array.
Also, you can name the same categorized data in a specific class by grabbing the elem
parameter like below.
$('td').each(function(index, elem) {
$(elem).addClass('table');
});