How to Add Elements to Array Using jQuery
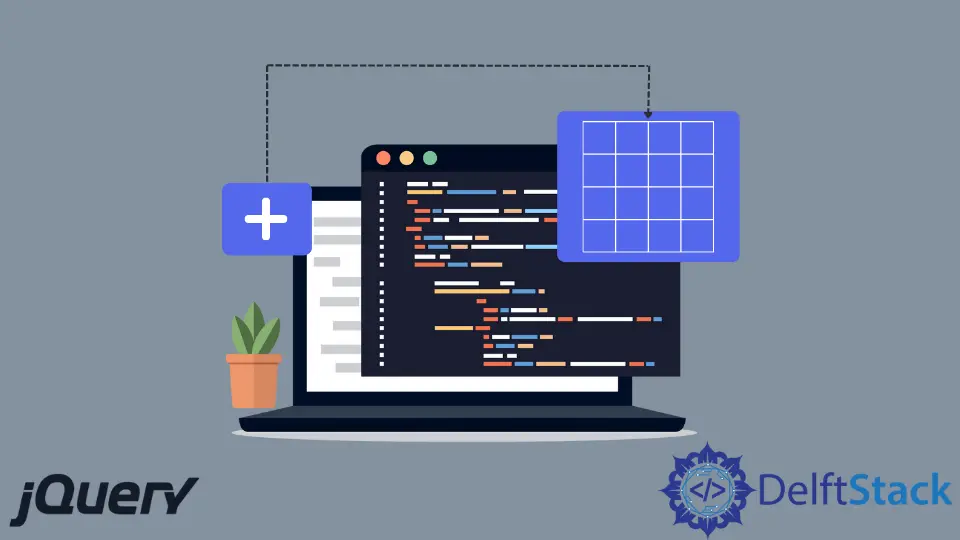
To add elements to an array in jQuery, you can use the .push()
and .merge()
methods in jQuery. This article teaches you how to use both methods using practical code examples.
Use the .push()
Method to Add Array Elements
In jQuery, you can use the .push()
method to add elements to an array. As the method’s name implies, it will push the element to the end of the array.
The following example shows you how to do this; in it, we have an empty array called array_1
. Afterward, you’ll use the .push()
method to add two numbers to the array.
$(document).ready(function() {
let array_1 = [];
console.log('The array before adding new elements: ', array_1);
// Push elements into the array using the
// push method.
array_1.push('23', '22');
console.log('The array after adding new elements: ', array_1);
});
Output:
The array before adding new elements: Array []
The array after adding new elements: Array [ "23", "22" ]
Use the .merge()
Method to Add Array Elements
The .merge()
method takes two arrays as arguments and combines them into a single array. With this, you can combine two arrays into an array containing elements from both arrays.
The following example shows you how to do it; what follows is the output in your web browser’s console.
$(document).ready(function() {
let first_array = [9, 3, 6, 8];
console.log('The array before addition: ', first_array)
let second_array = [23, 12];
// Merge the first and second arrays using
// the merge function in jQuery
$.merge(first_array, second_array);
console.log('The array after addition: ', first_array);
});
Output:
The array before addition: Array(4) [ 9, 3, 6, 8 ]
The array after addition: Array(6) [ 9, 3, 6, 8, 23, 12 ]
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn