Radio Button Validation in JavaScript
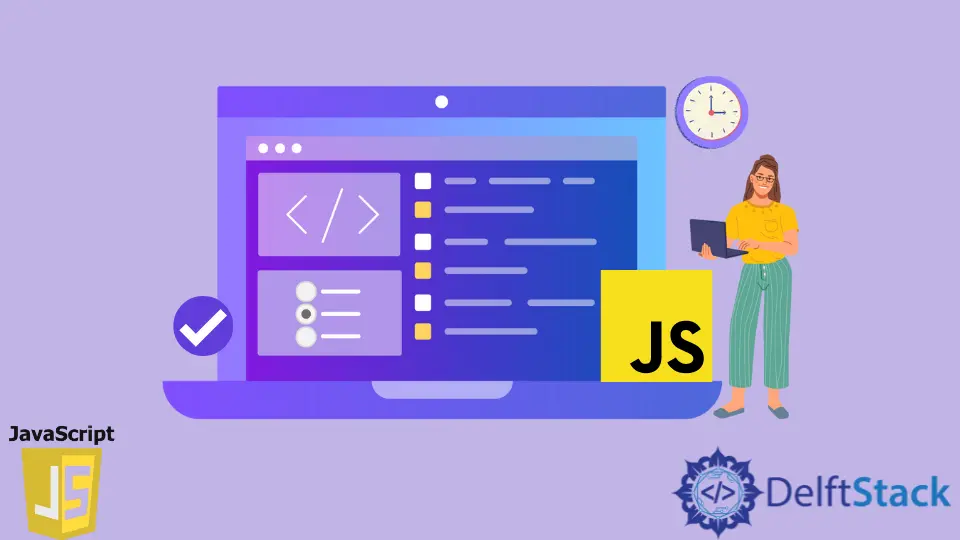
This article will discuss radio button validation in JavaScript.
Radio Button Validation in JavaScript
<input>
Radio items are typically used in radio groups, collections of radio buttons that describe a set of related options. Only one option in a given group can be selected at a time.
If you want to select multiple options, use Checkbox
instead of RadioButton
. Radio buttons are usually represented as small circles that fill or highlight when selected.
Syntax:
<input type="radio">
An option group is defined by giving each option button in the group the same name. Once an option group is set, selecting an option button deselects all currently chosen option buttons in the same group.
You can have any number of radio groups on a page, as long as each has its unique name. When submitting the form, you should always specify the value attribute to deliver the information to the server.
If the value attribute is not specified, the form data will assign the value on
to the entire radio group.
Let’s take an example where we are asking for the gender of the user.
Create three radio buttons with the name
property set to gender
with Male
, Female
, and Other
values. The form should be validated with gender, and without gender, it should give an alert.
<form onsubmit="return (checkForm())" name="userForm">
<input type="radio" id="male" name="gender" value="Male">
<label for="male">Male</label><br>
<input type="radio" id="female" name="gender" value="Female">
<label for="female">Female</label><br>
<input type="radio" id="other" name="gender" value="Other">
<label for="other">Other</label>
<br>
<input type="submit" value="Submit">
</form>
function checkForm() {
let chosenOption = '';
const len = document.userForm.gender.length;
for (i = 0; i < len; i++) {
if (document.userForm.gender[i].checked) {
chosenOption = document.userForm.gender[i].value
}
}
if (chosenOption == '') {
alert('Please choose your gender!');
return false;
} else {
console.log(chosenOption)
}
}
In the example, we first checked the length of the options. After that, we iterated through the options and checked if any options were checked.
If not, we return false
and show the alert to the user to fill in the options.
When you run the code in any browser, it will render the form. Select the gender and press submit; it will print the gender.
Without gender selection, it will give an alert message to choose the gender.
Output: (when an option is selected)
"Female"
Output: (when no option is selected)
You can run the code discussed in this tutorial here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn