How to Validate Zip Code Validation in JavaScript
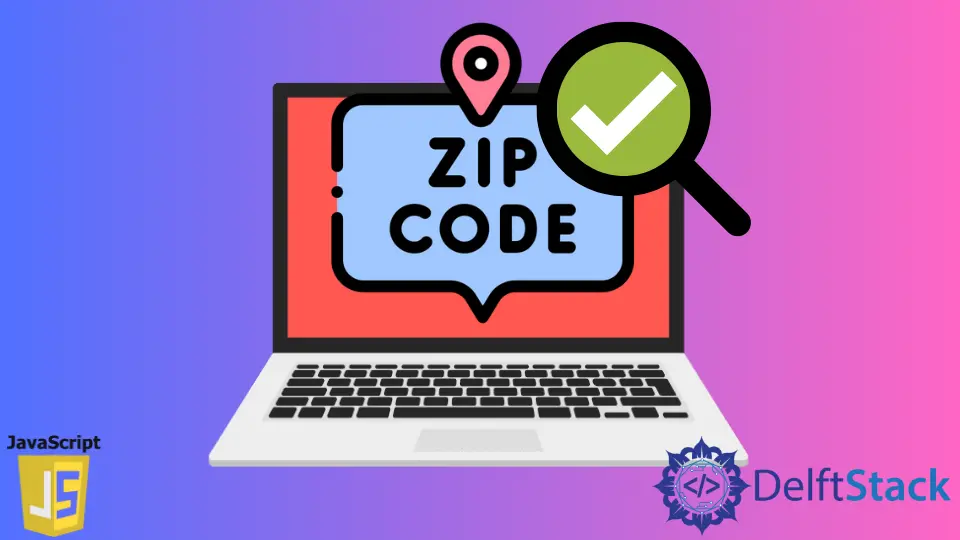
This article explains using regular expressions to validate zip codes using JavaScript code with different examples.
Zip Code Validation Using Regular Expression in JavaScript
We usually do string validations using regular expressions and the default test()
method in JavaScript.
We simply need to declare a regular expression known as regex like let regex = /(^\d{10}$)|(^\d{4}-\d{8}$)/;
and call a test()
method, passing our string as argument. That method will generate Boolean results (True/False).
The test()
method is a feature of ECMAScript1 (ES1), and all browsers fully support it. This method required a string to be searched as a parameter.
It will return the Boolean type value True
if it finds the correct match; otherwise, it will return False
.
Syntax:
let result = RegExp.test(anyString)
As we already know, zip codes may vary from country to country, and most of them contain a combination of five numeric values. So to validate the zip code string, we must declare a regular expression that will have only numeric values (0-9) and the length of five numbers.
Regex to validate zip code:
let validZipTest = /(^\d{5}$)|(^\d{5}-\d{4}$)/;
Example:
<!DOCTYPE html>
<html>
<head>
<title>
HTML | JavaScript zip code validation example
</title>
<script type="text/javascript"></script>
</head>
<body>
<script>
var validZipTest = /(^\d{5}$)|(^\d{5}-\d{4}$)/;
function checkValid()
{
let userZipCode = document.getElementById("zipcode").value;
//check validation of zip code
if(!validZipTest.test(userZipCode)) {
alert("Failed....! Please enter valid zip code...! ");
} else {
alert("Success....! Zip code Verified.");
}
}
</script>
<h1 style="color:blueviolet">DelftStack</h1>
<h3> JavaScript Zip code Validation </h3>
<form onsubmit ="return checkValid()">
<!-- zip code input -->
<td> Enter Zip code : </td>
<input id = "zipcode" value = "">
<br><br>
</form>
<button onclick="checkValid()">Check Valid</button>
</body>
<html>
- In the above HTML page source, we have created a form containing an input field to get the zip code from the user.
- Then, there is a button called
Check Valid
. We called thecheckValid()
function on that button’sclick
event. - We have initialized regex for zip code validation in the
validZipTest
variable. - We have already declared the
checkValid()
function; in that function, we are storing the user input value in theuserZipCode
variable. - Using the
if
condition, we have called thetest()
method on our regex and passed the user input zip code. - If the
test
method returns the Boolean valuetrue
, an alert will display the messageSuccess....! Zip code Verified.
- If the
test
method returns the Boolean valuefalse
, an alert will display the messageFailed....! Please enter valid zip code...!
.