How to Validate a Credit Card Number in JavaScript
- Identify the Format of a Credit Card
- Check Credit Card With Luhn’s Algorithm in JavaScript
- Display the Credit Card Issuer
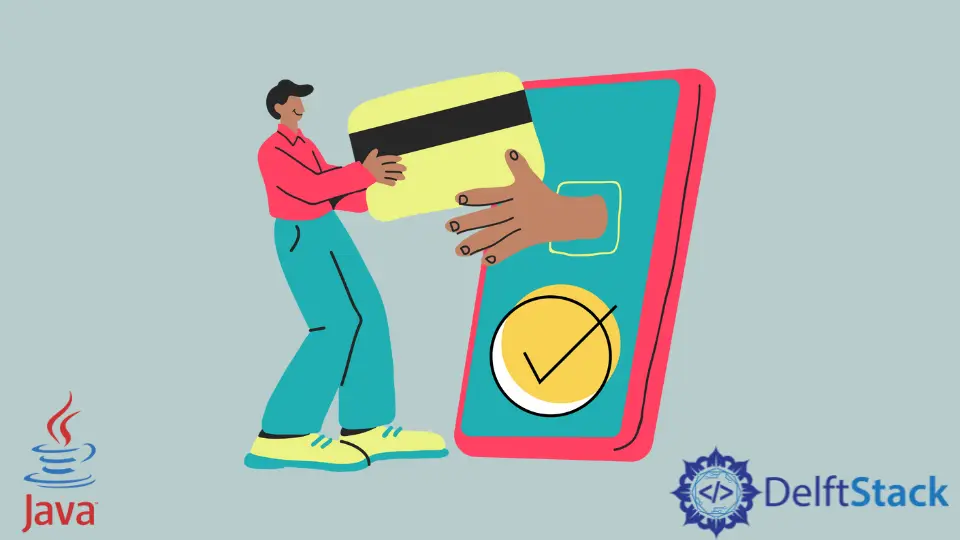
A verifying credit card is a crucial element when collecting money using an HTML form. This article will explain how to verify a credit card number (in a different format) using JavaScript.
There are different firms in the financial industry that sell credit cards. But there is no consistent format in the credit card numbers system, and it varies from company to business.
We are not sure that all the forms discussed here are accurate. From time to time, a firm might alter its numbering system. You may easily alter a format by changing the regular expression we have used for different cards.
Here are some formats of good credit cards.
Identify the Format of a Credit Card
Like any other magnetic stripe card, credit or debit card numbers have an identification format established by ISO/IEC 7812. Identifiers are generally 13 to 19 digits long, used for many reasons.
There are three pieces of information to the identification.
- IIN (Issuer identity number): This is a six-digit number that identifies the institution that issued the number. It usually starts with 4 or 5 for banks, so most credit cards begin with it.
- Account Number: A six- to twelve-digit number that serves as a unique identity.
- Check Digit: A single-digit is used to verify the identifier’s total.
Check Credit Card With Luhn’s Algorithm in JavaScript
IBM scientist Hans Peter Luhn created this technique to safeguard numeric IDs against unintentional errors. It is a straightforward algorithm.
- Begin with the number’s final digit.
- Make a double of each alternative digit, then add the remainder to 1 if the double value is more than 9.
- Divide the total of the doubled values, remainders, and each digit by ten.
- It is legitimate if it is divisible.
The following is a JavaScript implementation of Luhn’s algorithm for credit card validation.
const validCardNumber = numb => {
const regex = new RegExp('^[0-9]{13,19}$');
if (!regex.test(numb)) {
return false;
}
return luhnck(numb);
} const luhnck = val => {
let validsum = 0;
let k = 1;
for (let l = val.length - 1; l >= 0; l--) {
let calck = 0;
calck = Number(val.charAt(l)) * k;
if (calck > 9) {
validsum = validsum + 1;
calck = calck - 10;
}
validsum = validsum + calck;
if (k == 1) {
k = 2;
} else {
k = 1;
}
}
return (validsum % 10) == 0;
} console.log(validCardNumber('0987654321456012'));
Output:
false
Keep in mind that this method checks the format. You must still check on the server to see whether a credit card with this number exists.
Display the Credit Card Issuer
Each firm that provides the card has a unique identifying number that we may use to establish that this credit card belongs to them.
This is, for example, each organization’s format.
Now that we’ve grasped this format, we’ll write a JavaScript function to validate credit cards and identify their kind.
const validCreditcard =
cardnumb => {
const ccErrors = [];
ccErrors[0] = 'Unknown card type';
ccErrors[1] = 'No card number provided';
ccErrors[2] = 'Credit card number is in invalid format';
ccErrors[3] = 'Credit card number is invalid';
ccErrors[4] = 'Credit card number has an inappropriate number of digits';
ccErrors[5] =
'Warning! This credit card number is associated with a scam attempt';
const response = (success, message = null, type = null) =>
({message, success, type});
const validCardnumb =
numb => {
const regex = new RegExp('^[0-9]{13,19}$');
if (!regex.test(numb)) {
return false;
}
return luhnCheck(numb);
}
const luhnCheck =
val => {
let validsum = 0;
let k = 1;
for (let l = val.length - 1; l >= 0; l--) {
let calck = 0;
calck = Number(val.charAt(l)) * k;
if (calck > 9) {
validsum = validsum + 1;
calck = calck - 10;
}
validsum = validsum + calck;
if (k == 1) {
k = 2;
} else {
k = 1;
}
}
return (validsum % 10) == 0;
}
const cards = [];
cards[0] =
{name: 'Visa', length: '13,16', prefixes: '4', checkdigit: true};
cards[1] = {
name: 'MasterCard',
length: '16',
prefixes: '51,52,53,54,55',
checkdigit: true
};
cards[2] = {
name: 'DinersClub',
length: '14,16',
prefixes: '36,38,54,55',
checkdigit: true
};
cards[3] = {
name: 'CarteBlanche',
length: '14',
prefixes: '300,301,302,303,304,305',
checkdigit: true
};
cards[4] =
{name: 'AmEx', length: '15', prefixes: '34,37', checkdigit: true};
cards[5] = {
name: 'Discover',
length: '16',
prefixes: '6011,622,64,65',
checkdigit: true
};
cards[6] = {name: 'JCB', length: '16', prefixes: '35', checkdigit: true};
cards[7] = {
name: 'enRoute',
length: '15',
prefixes: '2014,2149',
checkdigit: true
};
cards[8] = {
name: 'Solo',
length: '16,18,19',
prefixes: '6334,6767',
checkdigit: true
};
cards[9] = {
name: 'Switch',
length: '16,18,19',
prefixes: '4903,4905,4911,4936,564182,633110,6333,6759',
checkdigit: true
};
cards[10] = {
name: 'Maestro',
length: '12,13,14,15,16,18,19',
prefixes: '5018,5020,5038,6304,6759,6761,6762,6763',
checkdigit: true
};
cards[11] = {
name: 'VisaElectron',
length: '16',
prefixes: '4026,417500,4508,4844,4913,4917',
checkdigit: true
};
cards[12] = {
name: 'LaserCard',
length: '16,17,18,19',
prefixes: '6304,6706,6771,6709',
checkdigit: true
};
if (cardnumb.length == 0) {
return response(false, ccErrors[1]);
}
cardnumb = cardnumb.replace(/\s/g, '');
if (!validCardnumb(cardnumb)) {
return response(false, ccErrors[2]);
}
if (cardnumb == '5490997771092064') {
return response(false, ccErrors[5]);
}
let lengthValid = false;
let prefixValid = false;
let cardCompany = '';
for (let l = 0; l < cards.length; l++) {
const prefix = cards[l].prefixes.split(',');
for (let k = 0; k < prefix.length; k++) {
const exp = new RegExp('^' + prefix[k]);
if (exp.test(cardnumb)) {
prefixValid = true;
}
}
if (prefixValid) {
const lengths = cards[l].length.split(',');
for (let k = 0; k < lengths.length; k++) {
if (cardnumb.length == lengths[k]) {
lengthValid = true;
}
}
}
if (lengthValid && prefixValid) {
cardCompany = cards[l].name;
return response(true, null, cardCompany);
}
}
if (!prefixValid) {
return response(false, ccErrors[3]);
}
if (!lengthValid) {
return response(false, ccErrors[4]);
}
return response(true, null, cardCompany);
}
console.log(validCreditcard('4111 1111 4321 1234'));
console.log(validCreditcard('3400 0000 0000 009'));
Output:
{
message: "Credit card number is in the invalid format",
success: false,
type: null
}
{
message: null,
success: true,
type: "AmEx"
}
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn