How to Pass JavaScript Function as Parameter
- Pass a General JavaScript Function as a Parameter
- Pass Both Function and Value to a JavaScript Function
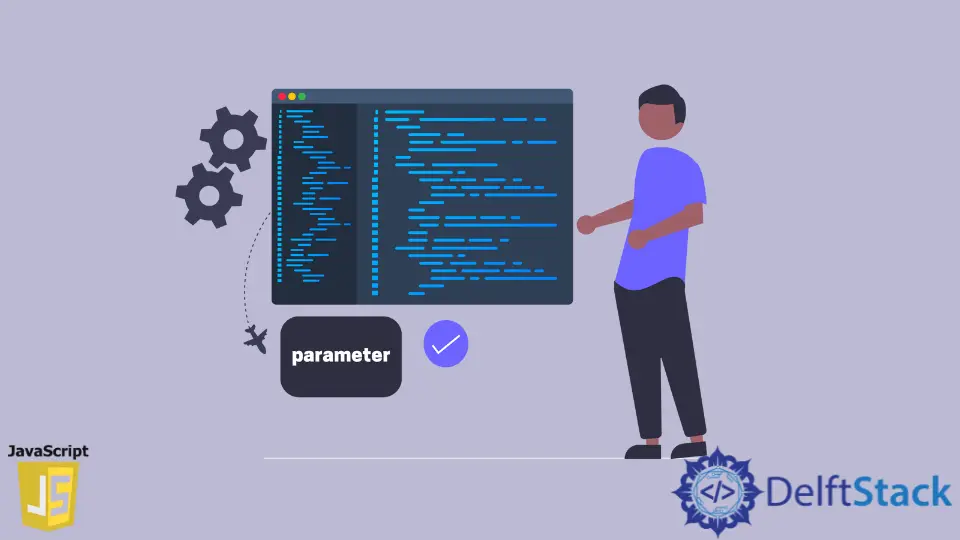
In JavaScript, passing a function as a parameter to another function is similar to passing values. The way to pass a function is to remove the parenthesis ()
of the function when you assign it as a parameter.
In the following sections, a function pass is demonstrated as a parameter.
Pass a General JavaScript Function as a Parameter
For this drive, we will initiate a function func2
with a code body and directly pass it to the function func1
. Later, after assigning func2
to func1
, we will call the func2
aka function_parameter
.
function func1(function_parameter) {
function_parameter();
}
function func2() {
console.log('okay!');
}
func1(func2);
Output:
The example depicts that func2
is passed to func1
. And when func1
is called, it checks its argument (func2)
and previews the code fenced by func2
.
Pass Both Function and Value to a JavaScript Function
JavaScript allows the passing of function and value together in another function, making functions more dynamic. This declaration will require an input of integer
, bool
, string
, or maybe even a function, and the other parameter is the function parameter.
We will see two examples of this category.
Function and value as function parameters:
function pass(value) {
return ('Hello ' + value);
}
function receive_pass(x, func) {
console.log(func(x));
}
receive_pass('David', pass);
Output:
Two functions as function parameters:
function pass1(value) {
return ('Hello ' + value);
}
function pass2() {
return (' Howdy!');
}
function receive_pass(func1, func2) {
console.log(func1('world!') + func2());
}
receive_pass(pass1, pass2);
Output:
As per the code instances, JavaScript takes functions as a parameter like any other regular data type. The core difference is when a function is called in the parenthesis of the argument, functions should be removed; otherwise, this can cause an error while running.
Related Article - JavaScript Function
- JavaScript Return Undefined
- Self-Executing Function in JavaScript
- How to Get Function Name in JavaScript
- JavaScript Optional Function Parameter
- The Meaning of => in JavaScript
- Difference Between JavaScript Inline and Predefined Functions