JavaScript === vs ==
- What is Loose Equality (==)?
- What is Strict Equality (===)?
- When to Use Which Operator
- Conclusion
- FAQ
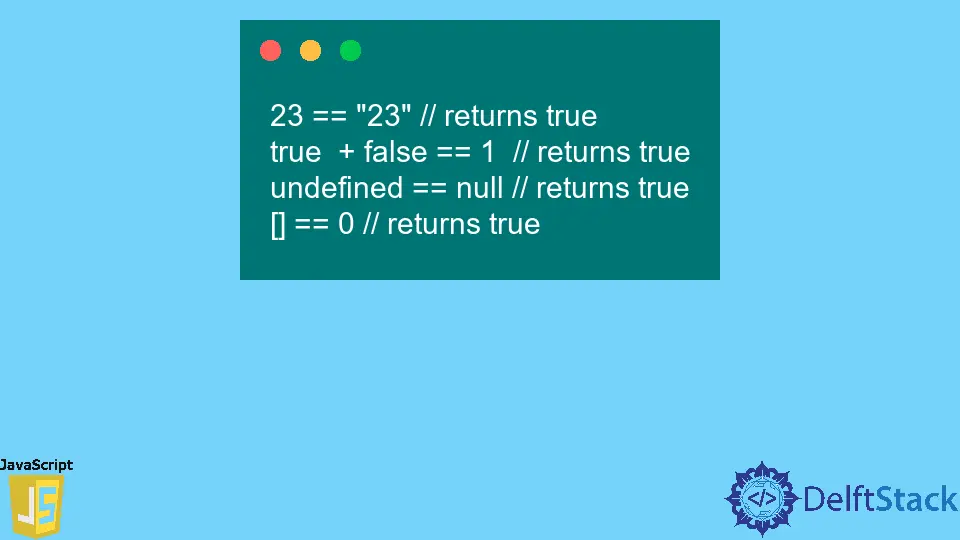
JavaScript is a versatile and widely-used programming language that powers much of the web today. One of the fundamental aspects of JavaScript is its comparison operators, particularly the difference between ==
(loose equality) and ===
(strict equality). While they may seem similar at first glance, understanding when to use each operator is crucial for writing efficient and bug-free code.
In this tutorial, we will explore the nuances of these two operators, providing clear examples and explanations to help you master their use. By the end of this article, you will know when to opt for ==
or ===
, ensuring that your comparisons yield the intended results.
What is Loose Equality (==)?
The ==
operator, known as loose equality, compares two values for equality after converting them to a common type. This means that if the values being compared are not of the same type, JavaScript will perform type coercion to make them comparable. While this can be convenient, it can also lead to unexpected results if you’re not careful.
Example of Loose Equality
console.log(5 == '5'); // true
console.log(null == undefined); // true
console.log(0 == false); // true
In the examples above, the first comparison converts the string ‘5’ into a number before comparing it to the number 5, resulting in true
. Similarly, null
and undefined
are considered equal in loose equality, which can sometimes lead to confusion. The comparison of 0
and false
also returns true
due to type coercion.
Using loose equality can be risky, especially in complex applications where you may not expect JavaScript to convert types. Therefore, it’s essential to understand the implications of using ==
in your code to avoid unintended behavior.
What is Strict Equality (===)?
On the other hand, the ===
operator, known as strict equality, checks for equality without performing type conversion. This means that both the value and the type must be the same for the comparison to return true
. Using strict equality is generally recommended because it leads to more predictable and reliable code.
Example of Strict Equality
console.log(5 === '5'); // false
console.log(null === undefined); // false
console.log(0 === false); // false
In these examples, strict equality does not perform any type coercion. The first comparison returns false
because the types of 5
(a number) and '5'
(a string) are different. Similarly, null
and undefined
are not considered equal, and 0
is not strictly equal to false
since they are different types.
By using strict equality, you can ensure that your comparisons are more accurate and reduce the chances of encountering unexpected bugs in your code. It is advisable to default to using ===
unless you have a specific reason to use ==
.
When to Use Which Operator
Choosing between ==
and ===
often depends on the context of your code. Here are some guidelines to help you decide:
-
Use
===
for Comparisons: Whenever possible, opt for strict equality to avoid type coercion issues. This practice leads to cleaner and more maintainable code. -
Use
==
with Caution: If you are working with values that may be of different types and you specifically want to allow type coercion, then using==
might be appropriate. However, be aware of the potential pitfalls. -
Consistency is Key: Establish a coding standard within your team or project to ensure that everyone is on the same page regarding which operator to use. Consistency helps improve readability and reduces confusion.
- Testing and Debugging: During testing and debugging, pay close attention to the types of values being compared. This practice will help you catch errors that may arise from using the wrong equality operator.
By following these guidelines, you can make informed decisions about which equality operator to use, ultimately leading to more robust and error-free JavaScript code.
Conclusion
In conclusion, understanding the difference between ==
and ===
in JavaScript is essential for any developer. While ==
may offer convenience through type coercion, it can also introduce unexpected behavior in your code. On the other hand, ===
provides a safer and more predictable way to compare values. By defaulting to strict equality, you can write cleaner, more reliable code that is easier to maintain. As you continue your journey with JavaScript, remember these principles, and you’ll be well on your way to mastering comparisons in this powerful language.
FAQ
-
What is the main difference between
==
and===
in JavaScript?
The main difference is that==
performs type coercion, while===
checks for both value and type equality without coercion. -
When should I use
==
in my JavaScript code?
You should use==
only when you specifically want to allow type coercion and are aware of the potential risks involved. -
Is it a good practice to use
===
all the time?
Yes, using===
is generally recommended as it leads to more predictable and reliable comparisons. -
Can you give an example where
==
produces unexpected results?
Yes, comparing0 == false
returnstrue
due to type coercion, which can be misleading. -
How can I ensure consistency in my team regarding equality operators?
Establish a coding standard or style guide that clearly defines when to use==
and===
, and share it with your team.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn