How to Overload Operator in JavaScript
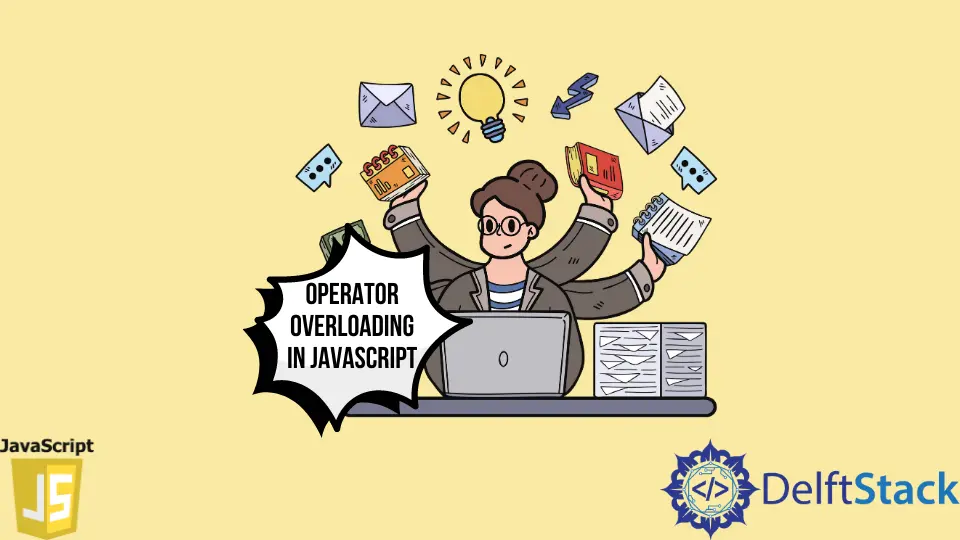
In the primitive stage, JavaScript did not have any explicit way of defining the operator overloading function. Later, there was an update where we would have to use the command npm install operator-overloading --save
to enable the syntax.
We would initiate a variable noting var overload = require('operator-overloading')
and then define the overload
function for our case. But the most recent use case has a more flexible way of defining the function scope for operator overloading.
We will depend on Babel Dev dependencies as it is easier for testing. We will explain the guide for installing its necessities in the following segment and showcase an example.
Implement Operator Overloading in JavaScript
The basic start is creating a directory and setting the necessary package.json
. So, we will first create a folder named operator overloading
and open it in Visual Studio Code.
In the terminal of VSCode, we will run the following commands.
npm init -y
npm install
After running these commands, the package.json
is not added to your folder; there will be one package-lock.json
. Unpack the contents of this file from this portal, copy the contents, and paste them into a new file called package.json
that you need to create in your base folder.
Next, follow the following commands in the terminal.
npm install --save-dev @babel/core @babel/preset-env @babel/cli @babel/node
npm install --save-dev @jetblack/operator-overloading
Now, create a .babelrc
file in the root directory and set its content like this:
{
"presets": [
[
"@babel/preset-env",
{
"targets" : {
"node": "current"
}
}
]
],
"plugins": ["module:@jetblack/operator-overloading"]
}
So, all your dependencies to enable the operator overloading are initiated. All you have to do is now create a JavaScript file and type codes to check the mechanism of operator overloading.
Code Snippet:
class Point {
constructor(x, y) {
this.x = x this.y = y
}
[Symbol.for('+')](other) {
const x = this.x + other.x
const y = this.y + other.y
return new Point(x, y);
}
}
// Built in operators still work.
const x1 = 2
const x2 = 3
const x3 = x1 - x2
console.log(x3)
// Overridden operators work!
const p1 = new Point(5, 5)
const p2 = new Point(2, 3)
const p3 = new Point(4, 2)
const p4 = p1 + p2
const p5 = p4 + p3
console.log(p5)
To test the output of the code above, try to open a Bash portal in VSCode and run the following. Technically, we will test our code via babel-node.cmd
.
./node_modules/.bin/babel-node.cmd ex.js
Here, ex.js
is our JavaScript file name. The output that infers is according to what is expected. Let’s check it out.
Output:
The class Point
is the initiated class with a constructor with two parameters. For the operator overloading case here, we have the syntax [Symbol.for(+)](other
).
For different operators, this syntax changes. However, the p1
and p2
objects have the corresponding x
summed up throughout the process.
Generally, the leftmost or starting instance is defined by this.x(x of p1 = 5)
, and the next x
of the p2 = 2
is denoted by other.x
. This is the basic concept of performing p1.add(p2)
.
In the final result of summing up all the user-defined classes, we get x=11
and y=10
.
There is another way of explaining operator overloading. ExtendScript is one of the facets that portrays the operation via the UX domain; also, PaperScript has a good way of defining the working principle.
You could use proxy
and Symbol
to complete the task. Also, the toString()
and valueOf()
work effortlessly to present the concept of this function.
The operators that do not support overloading are typeof
, ===
, !==
, &&
, ||
, and instanceof
.