How to Compare Numbers in JavaScript
- Use Comparison Operators to Compare Numbers in JavaScript
- Use Logical Operators to Compare Numbers in JavaScript
- Conclusion
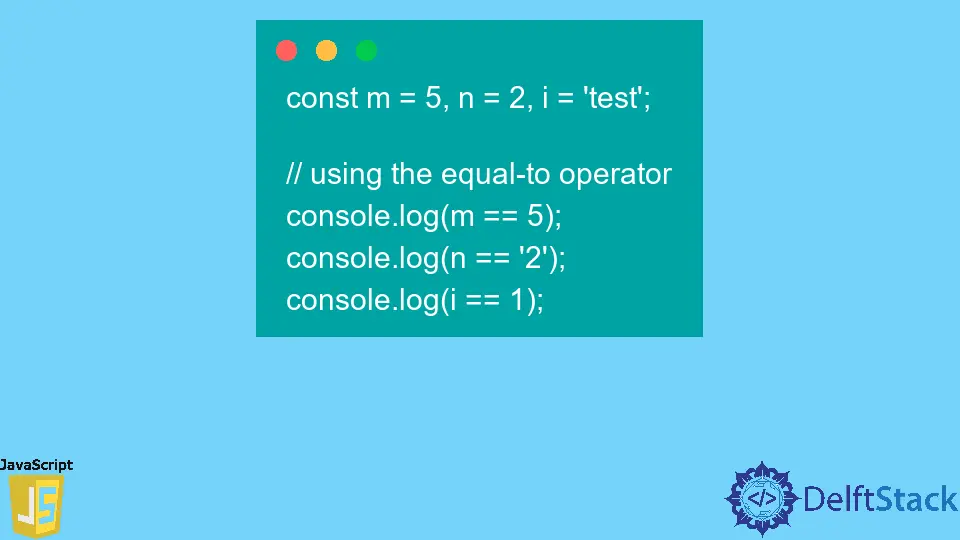
JavaScript offers numerous methods to compare basic values utilizing language constructs called operators. The JavaScript standard defines two distinct fundamental operator types: Comparison and Logical.
- Comparison operators show how different values are interconnected.
- Logical operators express the logical relationships between values.
These operators cannot manipulate complex values such as JavaScript objects, arrays, or functions. They can only manipulate simple values like strings, numbers, or JavaScript data types.
Ensure that the numbers or variables being compared are of the same data type; otherwise, you can receive an unexpected result. There are distinct guidelines for proper comparisons for each operator type.
Use Comparison Operators to Compare Numbers in JavaScript
The comparison operators in JavaScript assess both numeric and qualitative qualities. They initiate the relative status of values and whether they are overlapping or distinct.
The outcomes of comparison operators can either be true or false, which are Boolean values and compare two values. In loops and decision-making, comparison operators are utilized.
Use the JavaScript Greater-Than Operator (>
)
This operator determines the size of one value relative to another. Let’s use this operator and see the output.
const number = 5;
// using the greater-than operator
console.log(number > 3);
The output of the above snippet.
Use the JavaScript Less-Than Operator (<
)
This operator can check whether a value is smaller than another value. Let’s try using the less-than operator.
const number = 6;
// using the less-than operator
console.log(number < 10);
The outcome of the above code.
Use the JavaScript Equal Operators
There are two separate operators in JavaScript that check whether values are equal.
Use the JavaScript Equal-To Operator (==
)
Regardless of the data type of two values, the non-strict equals operator determines if they are indeed equal.
Let us try the below code:
const m = 5, n = 2, i = 'test';
// using the equal-to operator
console.log(m == 5);
console.log(n == '2');
console.log(i == 1);
The result of the above code.
Use the JavaScript Strict Equal-To Operator (===
)
The operator establishes whether the data type and value of two values are the same. Let’s look into the below snippet:
const number = 4;
// using the strict equal-to operator
console.log(number === 4);
console.log(number === '4');
We can get the result of the above code.
Use the JavaScript Not-Equal Operators
Two distinct operators in JavaScript can also be used to check whether two values are equal. They resemble equal operators, but in addition to the equal signs, they also have an exclamation point.
Use the JavaScript Not-Equal-To Operator (!=
)
Regardless of the data format, this operator determines whether two values are equal. Take the below code into consideration:
const m = 3, n = 4;
// using the not-equal-to operator
console.log(m != 2);
console.log(n != '4');
The result of the above code.
Use the JavaScript Strict Not-Equal-To Operator (!==
)
The operator determines whether two values differ in both data type and value. Let’s run the code below:
const m = 3, n = 4;
// using the strict not-equal-to operator
console.log(m !== 2);
console.log(n !== '4');
The outcome of the code above.
Use the JavaScript Greater-Than or Equal-To Operator (>=
)
Using this operator, we can see whether a provided value is equal to or greater than another. Let’s see how this comparison operator makes into use.
const m = 3, n = 4;
// using the greater-than or equal-to operator
console.log(m >= 3);
console.log(n >= 2);
Here, we can get an output as follows.
Use the JavaScript Less-Than or Equal-To Operator (<=
)
Determine whether a number is lower than or equal to another using JavaScript’s greater-than or equal-to operator.
Let’s see the functionality of this operator.
const number = 2;
// using the less-than or equal-to operator
console.log(number <= 3);
console.log(number <= 2);
Here, we can get an output as shown below.
Use Logical Operators to Compare Numbers in JavaScript
JavaScript evaluates logical operators in the order they appear, starting from the left. The true and false values are always evaluated using logical operators, and the final logical conclusion is frequently altered when grouping evaluations with parenthesis to shift their order.
Use the JavaScript AND
Operator (&&
)
The logical AND operator outputs true
if both conditions are true. It returns false
if not.
Let’s look into the functionality of this logical operator.
const number = 4;
// using the logical AND operator
console.log((number > 2) && (number < 2));
We can get an output as follows.
Use the JavaScript OR
Operator (||
)
The OR operator in JavaScript returns true
if its inputs are evaluated as true. In a situation where both or any of its values are false, it outputs false
.
Let’s use an example to show how to use the OR operator.
const number = 4;
// using the logical OR operator
console.log((number > 2) || (number < 2));
The code above produces the results listed below.
Use the JavaScript NOT
Operator (!
)
JavaScript’s NOT operator negates its defense. To negate a comparison’s result, place the operator directly in front of the parenthesis after and before the comparison.
Let’s look into the usage of the NOT operator.
const number = 4;
// using the NOT operator to get the negation
console.log(!((number > 2) || (number < 2)));
The outcome of the above code is as follows.
Conclusion
This article has explained different operators that can be used to compare numbers, including examples showing each operator’s functionality. The comparison and logical operators in JavaScript give programmers a lot of flexibility when exploring the connections between values and variables, and many JavaScript code structures, such as switch
statements and if-else
statements, depend on them for correct operation.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.