Short-Circuiting in JavaScript
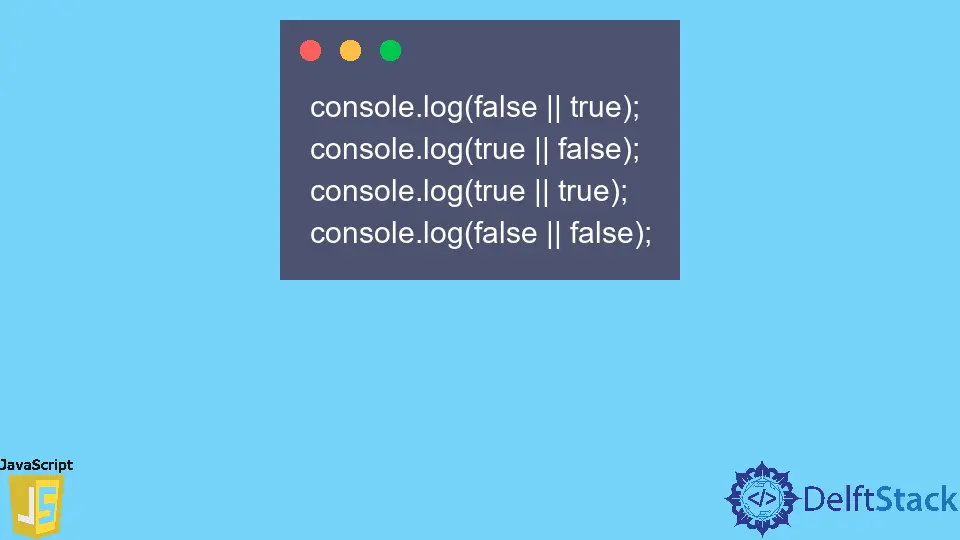
Every programming language supports various operators such as arithmetic, assignment, comparison, and logical operators. No difference with JavaScript, too; it supports all the operators that most programming languages support.
In this guide, the focus is on logical operators and their short-circuiting capability.
JavaScript Logical AND
Operator
Logical AND
and OR
operators are two popular logical operators that will evaluate to be true or false eventually. The logical AND
operator evaluates to true only when both operands are true; else, it will always return false.
Furthermore, the AND
operator will return false if either operand is false.
console.log(false && true);
console.log(false && false);
console.log(true && false);
console.log(true && true);
Output:
JavaScript Logical OR
Operator
In contrast to the logical AND
operator, the logical OR
operator returns true if either operand is true. Whenever both operands are false, this operator gives a false result.
console.log(false || true);
console.log(true || false);
console.log(true || true);
console.log(false || false);
Output:
Short Circuiting in JavaScript
JavaScript evaluates the above two operators from left to right within an expression. In the worst-case scenario, it starts from the beginning (left end) and evaluates to the right end until all the operands are assessed.
It is not the case when short-circuiting is in place. With JavaScript short-circuiting mechanism, the expression is evaluated from left to right until the remaining operand’s result will not affect the already assessed result value.
Short Circuiting in Logical AND
Operator
The logical AND
operator will be short-circuited if the first operand is false. Regardless of whether the subsequent operand is true or false, the expression will be evaluated as false.
Hence, the JavaScript run time will not assess the remaining operands in the expression.
Let’s look at the following example, which demonstrates the above behavior.
console.log(true && 'it should come here and print me.');
Output:
Since the first operand is true
, this expression will not short circuit. Hence, it will evaluate the next operand as well. As expected, the second operand value has been printed to the console, which means JavaScript has assessed both operands.
Next, we will be using false
as the first operand.
console.log(false && 'short-circuits and will not print me');
Output:
As expected, the output is false
, and JavaScript has not further evaluated the second operand. It means the expression has been short-circuited.
Short Circuiting in Logical OR
Operator
In contrast to the logical AND
operator, the logical OR
operator gets short-circuited when the first operand is true, as shown below.
console.log(true || 'i will not be printed');
Output:
As you can see, the output is true
, so the second operand is not even assessed.
Short-circuiting is a useful feature in JavaScript that ignores the unwanted portions of code and assist in efficient processing.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.