How to Set the Scroll Position in JavaScript
-
Use the
scrollTo()
Method to Set the Scroll Position in JavaScript -
Use the
scrollBy()
Method to Set the Scroll Position in JavaScript -
Use the
scrollLeft
andscrollTop
Properties Along With HTML Attributeonscroll
-
Use
eventListener
to Set the Scroll Position in JavaScript
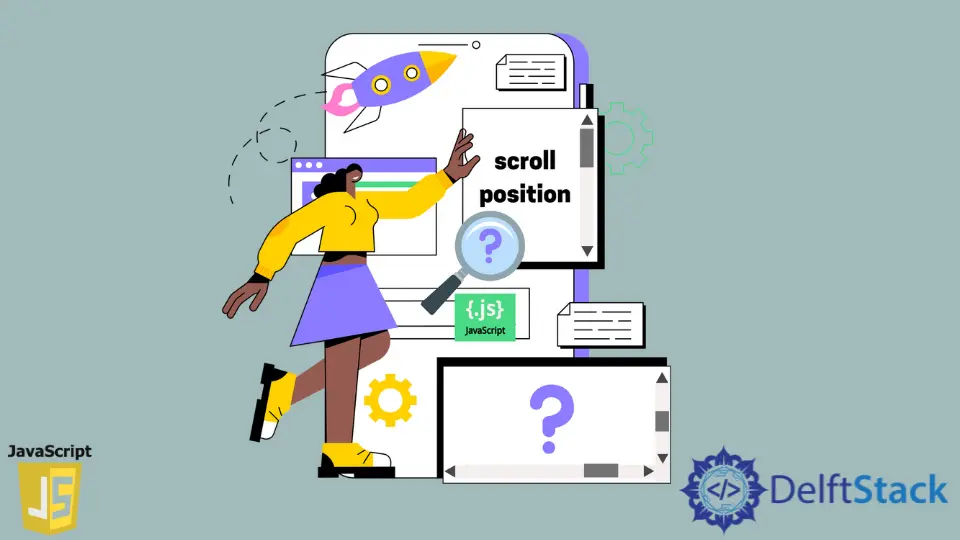
This tutorial will introduce how to set up the scroll position in an interface in JavaScript.
Use the scrollTo()
Method to Set the Scroll Position in JavaScript
In this example, we will initially set the dimension of the body element. For the next step, we will use a button
element with an onclick
attribute to trigger the function scrollL()
.
We will also set the position of this button to be fixed. We will add the function definition in the script tag, and there will be the command window.scrollTo()
.
The scrollTo()
method will manipulate the window object. Specifically, it will make a left jump to 20 units as we have set the value.
The former parameter is for the horizontal movement, aka left scroll, and the latter is for the vertical, top scroll.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<style>
body {
width: 2000px;
height: 2000px;}
</style>
</head>
<body>
<p>Example of ScrollTo</p>
<button onclick="scrollL()" style="position:fixed">Scroll Horizontally!</button><br><br>
<script>
function scrollL() {
window.scrollTo(20, 0);
}
</script>
</body>
</html>
Output:
As we have set it to shift up to 20 units, the window object will move horizontally in the output.
Since the scrollTo()
method performs the task only once, the button will make the viewport move only for a single time.
As we can see, we get to shift the viewport frame from (0,0) to (20,0). Similarly, we can set values for the vertical displacement.
Use the scrollBy()
Method to Set the Scroll Position in JavaScript
The scrollBy()
method works similarly as the scrollTo()
method. But in this case, the movement is not based on one step; rather, it will scroll as much as we want.
In the code below, we have set the horizontal value to 10. This implies that the frame will move from (0,0) to (10,0).
Also, after each press on the button element, we will get a displacement of 10 units (px).
Check the provided example for a better understanding.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<style>
body {
width: 2000px;
}
</style>
</head>
<body>
<p>Example of ScrollBy</p>
<button onclick="scrollL()" style="position:fixed">Scroll Horizontally!</button><br><br>
<script>
function scrollL() {
window.scrollBy(10, 0);
}
</script>
</body>
</html>
Output:
Use the scrollLeft
and scrollTop
Properties Along With HTML Attribute onscroll
We will use these two properties on the element we wish to add the scroll positions.
In our example, the parent div=div1
has a smaller dimension than the child div=content
. This will create an overflow, so we must set the properties.
Also, notice the HTML attribute onscroll
that invokes a function keepUpTheScroll()
.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<style>
#div1 {
height: 250px;
width: 250px;
overflow: auto;
}
#content{
width:500px;
height:1250px;
background:black;
color:white;
}
</style>
</head>
<body>
<div id="div1" onscroll="keepUpTheScroll()">
<div id="content"><br> Scroll!</div>
</div>
<p id="show"></p>
<script>
function keepUpTheScroll(){
var div = document.getElementById('div1');
var x = div.scrollLeft;
var y = div.scrollTop;
document.getElementById ("show").innerHTML = "Horizontally: " + x + "px<br>Vertically: " + y + "px";
}
</script>
</body>
</html>
Output:
The scrollLeft
and scrollTop
return the exact frame position, aka scroll position relative to other dimensions. In our output, we get the horizontal scroll 9.6 and the vertical scroll 14.39.
Even though scrollTo()
and scrollBy()
require minimal lines of code to implement, these properties hand over the entire panel to your desired position.
Use eventListener
to Set the Scroll Position in JavaScript
For the addEventListener()
, we will again use the scrollLeft
and scrollTop
properties to deal with the scroll position values. The method uses scroll
to apply the functionalities.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<style>
#div1 {
height: 250px;
width: 250px;
overflow: auto;
}
#content{
width:500px;
height:1250px;
background:purple;
color:white;
}
</style>
</head>
<body>
<div id="div1" onscroll="keepUpTheScroll()">
<div id="content"><br> Scroll!</div>
</div>
<p id="show"></p>
<script>
var div = document.getElementById('div1');
var show = document.getElementById('show');
div.addEventListener("scroll", event =>{
show.innerHTML = `scrollTop: ${div.scrollTop} <br>
scrollLeft: ${div.scrollLeft}`;
});
</script>
</body>
</html>
Output:
In the example, we have considered the div
element and manipulated it to get the horizontal moved value to 4 and the vertical moved value to 9.6.
However, we used a small portion of the jQuery
command for scrollLeft
and scrollTop
. Another way is to use the scroll()
method which functions similar to scrollTo()
.