The outerHTML Property in JavaScript
-
Get the HTML of an Element Using
outerHTML
in JavaScript -
Set the HTML of an Element Using
outerHTML
in JavaScript - Conclusion
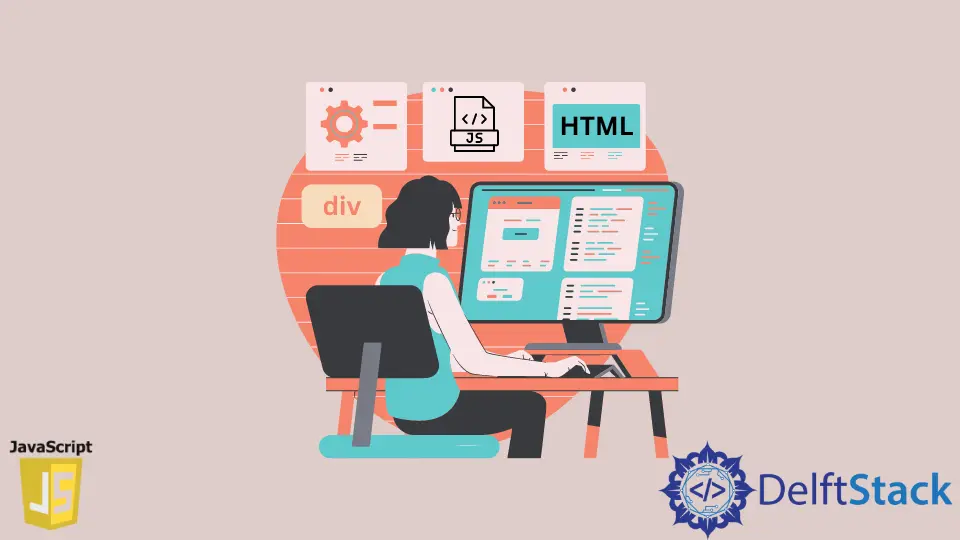
outerHTML
is a JavaScript property that allows you to get and set the HTML of an element. For example, if you have an HTML tag as follows then using the outerHTML
property you will get the <div> </div>
as the output.
<div>Text</div>
If the div
has attributes like class
or id
, you will get all such details with the HTML. The outerHTML
is used to return or set the HTML of an element.
Opposite to this is the innerHTML
property that sets or returns the text present within the tags. In this case, the innerHTML
property will return the word Text
which is enclosed with the div
HTML tag.
Let’s take an example of how to get and set the HTML of an element using the outerHTML
property. We currently have a single div
element inside our HTML with a class
of oldElement
.
<body>
<div class="oldElement">Text</div>
</body>
We have added some text inside the div
element. First, we will see how to get the HTML of the above element using the outerHTML
property, and then we will see how to replace or set this HTML div
with a different HTML element.
Get the HTML of an Element Using outerHTML
in JavaScript
To get the HTML of the div
element, we first have to store the reference of the div
inside the JavaScript code using its class
attribute and use the getElementsByClassName()
method, which is a part of DOM API.
<!DOCTYPE html>
<html>
<body>
<div class="oldElement">This is an old element.</div>
</body>
<script>
var oldElement = document.getElementsByClassName('oldElement')[0];
console.log(oldElement.outerHTML)
</script>
</html>
Since we are accessing the element using its class name, we also have to use [0]
at the end because the getElementsByClassName()
returns an array since we only have one element with that class name inside our DOM.
Therefore, we will access the [0]
element of the array. Then we will store that element inside the oldElement
variable.
To get the HTML of this element stored inside the oldElement
variable, we will use the outerHTML
on that variable as shown above code print it on the console.
Output:
Set the HTML of an Element Using outerHTML
in JavaScript
Let’s now see how to set the HTML for the same div
element, which we have already stored inside the oldElement
variable using the outerHTML
property.
We will use the assignment (=
) operator; since we want to set or assign a value to this element, we will use the assignment (=
) operator.
The outerHTML
only accepts HTML elements in the form of a string. Therefore, we have assigned an h1
tag as a string with some text inside it to the oldElement
variable using the outerHTML
property.
<!DOCTYPE html>
<html>
<body>
<div class="oldElement">This is an old element.</div>
</body>
<script>
var oldElement = document.getElementsByClassName('oldElement')[0];
oldElement.outerHTML = "<h1>This is an new element.</h1>";
</script>
</html>
This will replace the entire div
element and its value with this new HTML element. If you look at the DOM tree, you will see that the div
HTML element has been replaced with the h1
HTML element.
See the below image for details.
Conclusion
To get the HTML of any element, we use the outerHTML
property. This property returns the outer HTML of an element. It can replace an HTML element with a new one, just bypassing the new HTML element as a string to this property.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn