JavaScript nextSibling Property
-
Use the
nextSibling
Property to Fetch Element Node in JavaScript -
Use the
nextSibling
Property to Fetch Text Node in JavaScript
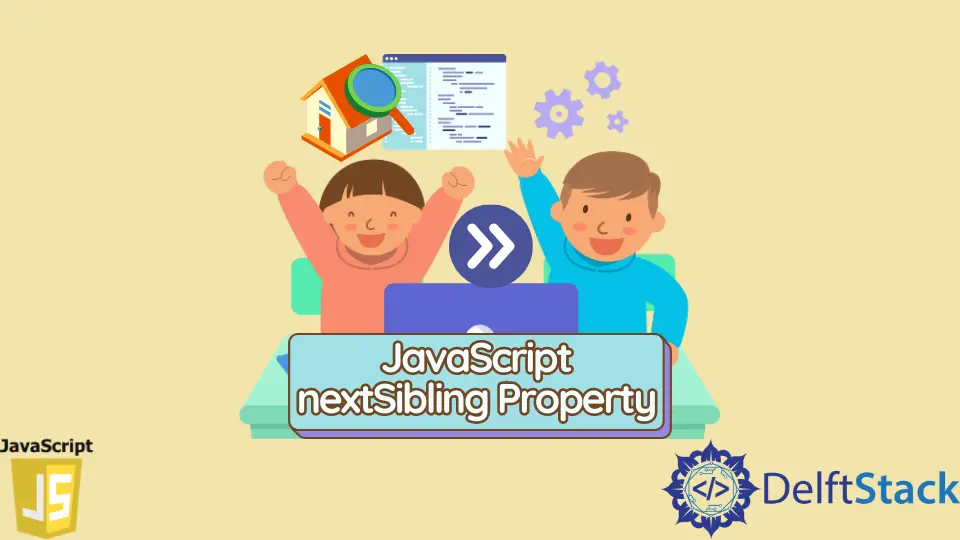
In JavaScript, we have the nextSibling
and nextElementSibling
properties. Technically, both have similar functionalities, but the nextSibling
is a little more explicit in defining nodes.
The nextSibling
property fetches the next node of a certain specified node. In this case, the node can be either an element node, text node, or comment node but the nextElementSibling
only focuses on grabbing the element nodes and discards all other characters, whitespace, etc.
Here, we will only visualize how the nextSibling
property performs and the retrieval of the upcoming nodes. This overall task is to get track of the HTML structure and a better purpose of use.
Use the nextSibling
Property to Fetch Element Node in JavaScript
We will create 3 p
tags in the following example, and each will have its id
. Let us consider that the p
elements do not have any whitespace or any other character in between and only the bare tags.
We will take the first
element in the script
tag and check what it shows for the later cases.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<p id="first">First</p><p id="second">Second</p><p id="third">Third</p>
<script>
console.log( document.getElementById('first').nextSibling.innerText);
console.log( document.getElementById('first').nextSibling.nextSibling.innerText);
</script>
</body>
</html>
Output:
As we have considered the first p
tag, the immediate next node is the id="second"
element node. Similarly, we consoled out the element node next to the "second"
that is the "third"
by using the nextSibling
property twice with the first node.
Use the nextSibling
Property to Fetch Text Node in JavaScript
The nextSibling
property has consideration for element node, text node, and comment node. So, if we can detect any text node and whitespaces, the nextSibling
property will count that as the next occurrence.
In our case, we will set the p
tag one after another, followed by a space
. Consequently, we will get undefined
, which refers to single whitespaces, spaces,
etc.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<p id="first">First</p>
<p id="second">Second</p>
<p id="third">Third</p>
<script>
console.log( document.getElementById('first').nextSibling.innerText);
console.log( document.getElementById('first').nextSibling.nextSibling.innerText);
</script>
</body>
</html>
Output:
So, the next node after the first node is a text node that is the space
, and thus we get undefined
as an output, and the next node is now the "second"
element node. This can be inferred that the nextSibling
property does not ignore any nodes.