How to Create Private Properties in JavaScript
- The ES6 Classes in JavaScript
- Public Properties in JavaScript
- Private Properties in JavaScript
- Different Methods to Create Private Properties in JavaScript
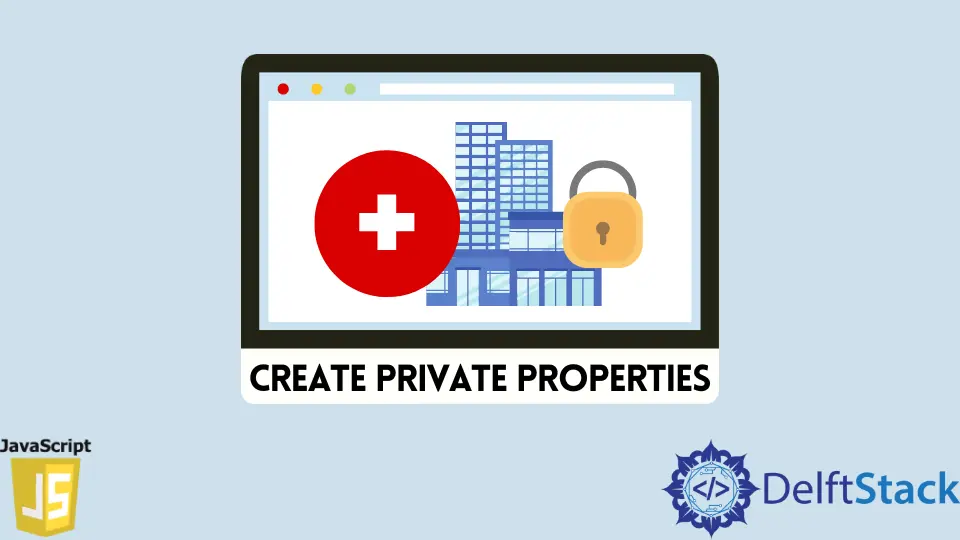
This article will explain ES6 classes, private properties using ES6 classes, and how you can make and declare these private properties in JavaScript. Moreover, different methods to implement the private
keyword in JavaScript are discussed and explained.
The ES6 Classes in JavaScript
Object orientation is a programming paradigm that is based on real-world modeling. It views a program as a collection of objects that communicate through methods.
ES6 also supports these object-oriented components.
In ES6, the class
keyword may be used to build classes. Declaring classes or utilizing class expressions can be used to include them in the code.
Syntax:
class Class_name {}
// declaring a class
var var_name = new Class_name {}
// class expression
Public Properties in JavaScript
The keyword this
declares public properties and methods in JavaScript. They can be accessed both inside and outside the specified function.
Private Properties in JavaScript
In object-oriented languages, the private
keyword is an access modifier that restricts access to properties and methods within the specified class. This makes it simple to conceal underlying logic that should be concealed from prying eyes and not engaged with outside the classroom.
But, with JavaScript, how can you achieve the same effect? private
is not a reserved keyword; instead, it can only be used as a variable.
Syntax:
const private = 'private';
private.js
// private is not a reserved keyword, and you can set it to anything.
Different Methods to Create Private Properties in JavaScript
Fortunately, there are a few solutions for implementing private properties and functions in JavaScript. Methods to implement private properties are given below:
Use closure()
to Create Private Properties in JavaScript
Using closure()
is one of the choices to implement private properties in JavaScript. Inner functions with access to the variables of the surrounding function are known as closures.
Example:
(function one() {
var a = 'Hello World';
// The scope of the outer function is accessible to this inner function.
(function two() {
console.log(a); // This will give output of "Hello World"
})();
})();
Output:
You can access this link to see the working of this code. This means assigning a variable to the topmost self-invoking function call and only exposing part of its inner functions via a function return.
Use ES6 Classes to Create Private Properties in JavaScript
Private properties aren’t supported natively in ES6 classes. However, you can obtain similar effect by putting the new properties within a class function Object() { [native code] }
. We can utilize the getters and setters to access the hidden properties rather than attaching them to the object.
It’s worth noting that each new instance of the class redefines the getters and setters. You may utilize the class
keyword introduced in ES6 to make your code more akin to the OOP approach.
Declare properties and methods outside of your class to make them private.
Example:
class Person {
constructor(name) {
var _name = name this.setName = function(name) {
_name = name;
} this.getName = function() {
return _name;
}
}
}
Use the Latest ECMAScript Proposal to Create Private Properties in JavaScript
You may be able to use the official way of using private fields in the future. Currently, this is at stage 3 proposal as of writing this article.
With the latest version of Babel today, you can already use it. To define private properties or methods, prefix them with a hash.
Example:
class Something {
#property;
constructor() {
this.#property = 'test';
}
#privateMethod() {
return 'hello world';
}
getPrivateMessage() {
return this.#property;
}
}
const instance = new Something();
console.log(instance.property); // It will show "undefined"
console.log(instance.privateMethod); // This will show "undefined"
console.log(instance.getPrivateMessage()); // This will show "test"
You can click this link to watch the execution of the above code segment.
Access Public Property from Private Property in JavaScript
You must understand that this is a MODULE, not a class that needs to be instantiated. To access the public property from private property, use self.
in the public methods/vars and nothing in the private, and it will all work.
Example:
var PersonModule = (function() {
var self = {};
var privateChangeNameToBob = function() {
self.name = 'World';
};
self.sayHello = function() {
console.log('Hello ' + self.name);
};
self.changeName = function() {
privateChangeNameToBob();
};
self.name = '';
return self;
})();
PersonModule.name = 'Test';
PersonModule.changeName();
PersonModule.sayHello();
Output:
Click this link to access the execution of this code.