The hasOwnProperty in JavaScript
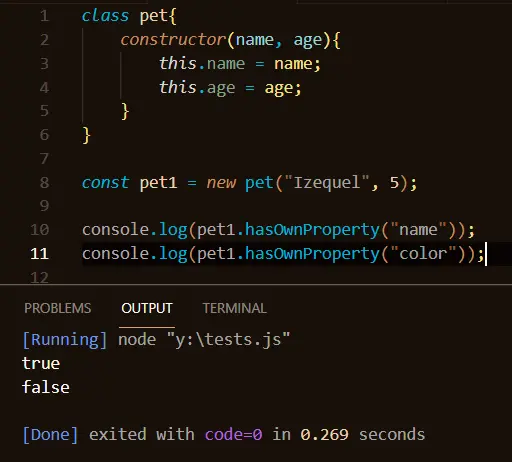
We often see a dilemma regarding the in
convention and the hasOwnProperty
, but both work differently in a specified case. The main concept is in
works for objects that have inherited properties and results in true
, whereas hasOwnProperty
returns true for only properties that are not inherited instead explicitly defined.
Here, we will only discuss the hasOwnProperty
in JavaScript.
Check of hasOwnProperty
on Objects in JavaScript
In JavaScript, the structure to check if a property is defined is like this - objectName.hasOwnProprty(property)
. Here, an object can be an array
or can be a key-value
pair. You can check the directed path.
Use of hasOwnProperty
on Array Object in JavaScript
Arrays are indexed from 0
and proceed till the last available element. So, we prefer the index values for calling the property of an array object. The following instance gives a clear idea.
Code Snippet:
var myObj = ['Liza', 'Kary', 'Blake'];
console.log(myObj.hasOwnProperty(2));
console.log(myObj.hasOwnProperty(3));
Output:
Use of hasOwnProperty
on key-value
Pair Objects in JavaScript
In this regard, we will consider a dictionary that will have key values (string
) and properties (any datatype). Let’s go for the example below:
Code Snippet:
var myObj = {
pet : "penguine",
age : 5,
}
console.log(myObj.hasOwnProperty("age"));
console.log(myObj.hasOwnProperty("color"));
Output:
As you can see, the object myObj
has only two keys - pet
and age
. If we call only these two properties by the hasOwnProperty
we will see the console resulting true
. Otherwise, we called a random property color
, which results in false
.
Check of hasOwnProperty
on Objects of a Class
JavaScript ES6 has an additional feature of class
, and the use of constructor
enables the definition of some dynamic objects. While after declaring the objects, you can easily access them by defining your value for them. The demonstration will clear the explanation of this section. Also, for any custom value, if the property exists within the class constructor
, you will get the answer true
; otherwise, false
.
Code Snippet:
class pet {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const pet1 = new pet('Izequel', 5);
console.log(pet1.hasOwnProperty('name'));
console.log(pet1.hasOwnProperty('color'));