Not in Operator in JavaScript
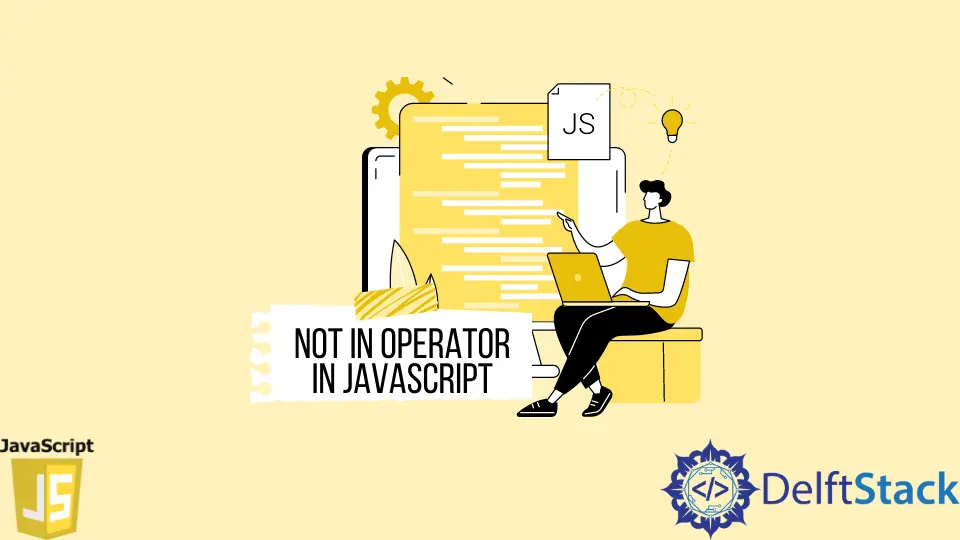
Objects are key-value properties that are most commonly used in JavaScript. To check whether a certain property exists or not, JavaScript offers several possibilities. Today’s post is going to show you how to check whether or not a particular property exists or not on an object in JavaScript.
Check Property Exists or Not in JavaScript
Properties are paired with the key value stored in an object. An object
can contain many such properties. Whether or not a certain property exists in JavaScript is determined by the operator id in object
or Object.prototype.hasOwnProperty()
.
Object.prototype.hasOwnProperty()
in JavaScript
This is a built-in method provided by JavaScript to check whether the specified property belongs to an Object or not. It iterates the object and returns the boolean value according to the result.
Syntax:
Object.prototype.hasOwnProperty(prop);
This function takes prop
as an input in the string
format. It is a mandatory parameter. This method only checks whether the given property exists or not in the object irrespective of the value. It will return true
even if the property value is null
or undefined
. This method can also be called on an Array
since the array is descended from an Object. For more information, read the documentation of the hasOwnProperty()
method.
const inputObject = {
id: 42,
name: 'John Doe'
};
console.log(inputObject.hasOwnProperty('name'));
if (!inputObject.hasOwnProperty('email')) {
inputObject.email = 'Johndoe@gmail.com';
}
console.log(inputObject);
In the above code block, we defined two properties of an object id
and name
. When you will pass the inputObject.hasOwnProperty('name')
, it will check if the name property exist or not in inputObject
. If you want the opposite condition to be checked, add !
before the condition, and it will negate the check. For example, you can add a new property if it does not exist inside an object. The output given by the code above can be seen below.
Output:
true
{
email: "Johndoe@gmail.com",
id: 42,
name: "John Doe"
}
the in
Operator in JavaScript
It is an in-built method provided by JavaScript, which checks if an object or prototype chain owns the specified property. It iterates the object and returns the boolean value according to the result.
Syntax:
prop in object
This function takes prop
as an input in the string
format. It is a mandatory parameter. This method only checks whether the given property or array index exists or not in Object
or its prototype chain
. This method can also be called on an Array
since the array is descended from an Object. For more information, read the documentation of the in
operator method.
The main difference between hasOwnProperty
and in
operator is that the prior one returns false if the property is inherited inside the object or has not been declared. In comparison, the in
operator does not check for the specified property in the object’s prototype chain. For example, the Object
base class has hasOwnProperty
as a function, so hasOwnProperty
will return false
whereas in
operator will return true
.
const inputObject = {
id: 42,
name: 'John Doe'
};
console.log('name' in inputObject);
if (!('phone' in inputObject)) {
inputObject.phone = '7878787878';
}
console.log(inputObject);
In the above code block, we defined two properties of an object id
and name
. When you pass name
in inputObject
, it will check whether the name property exists in inputObject
. If you want the opposite condition to be checked, add !
before the condition, negating the check. For example, you can add a new property, phone
, if it does not exist inside an object. The output given by the code snippet above is seen below.
Output:
true
{
id: 42,
name: "John Doe",
phone: "7878787878"
}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn