How to Submit Form Using JavaScript
- Create HTML Form
- Access the Form
- Get Values of Form Fields in JavaScript
- Validate Data From Form Fields in JavaScript
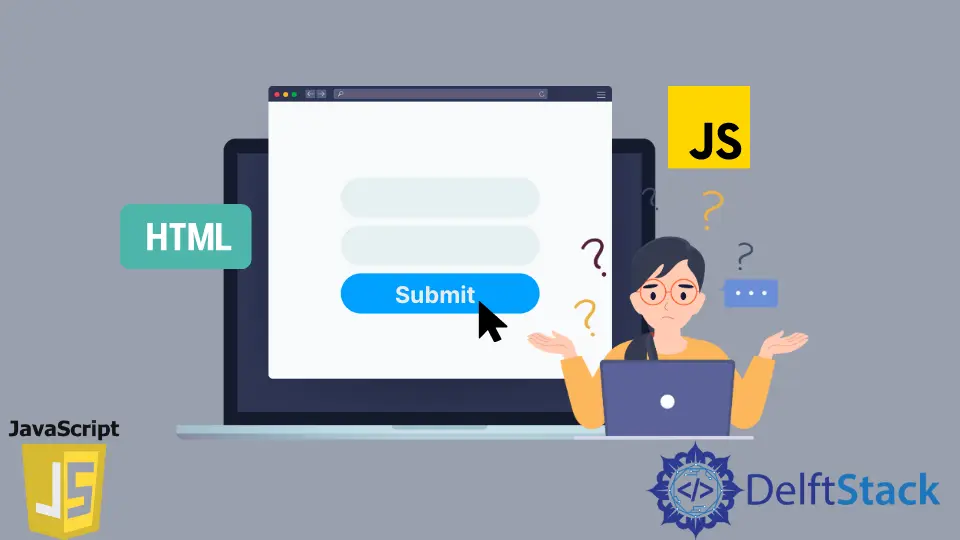
This tutorial will introduce how to submit a form using JavaScript.
Forms help to take data from users and store that in a structured database. Form submission is a key event when we are working with various apps and websites. It is not as simple as calling form.submit()
or clicking on the submit button. When the form is submitted, the submit event is fired right before the request is sent to the server. This is where JavaScript will come into action and validate the data entered. We can even create objects and add various attributes.
So, to make a form submission, we have first to create a form, access the form, get values of various fields, validate all the values and then either click on submit button/call for.submit()
.
Create HTML Form
We can create a HTML form using the <form>
element.
<form action="/" method="post" id="signup-form">
<input type="text" name="Username" placeholder="Full Name">
<input type="email" name="EmailAddress" placeholder="Email Address">
<button type="submit">Submit</button>
</form>
The above form has two attributes: action
and method
.
action
: It specifies the URL that will handle the process of form submission.method
: It specifies the HTTP method used to submit the form. Thepost
method helps store data in a database by sending the data to the server in the body of the request. Theget
method appends the form data to a URL that has?
.
Access the Form
We can access form elements using any one of the JavaScript selectors like getElementByTag, getElementById, etc.
let form = document.getElementById('signup-form');
Get Values of Form Fields in JavaScript
We can access the input fields in the form either by using the form elements array or referencing the field’s id. After we have accessed the field, we can use the .values
attribute to get the values stored inside that field. Once we have acquired the form data, we can validate data before we proceed with the final form submission.
// Syntax1:
let name = form.elements[0];
let username = name.value;
// Syntax2:
let name = form.elements['name'];
let username = name.value;
Validate Data From Form Fields in JavaScript
We add an event listener to the submit button and call our validation procedure before moving to the next page. For Example: for a signup page, the user must provide both the email and password.
// Function to check that the input field is not empty while submitting
function requireValue(input) {
return !(input.value.trim() === '');
}
// Event listener to perform the validation when user clicks on submit button
form.addEventListener('submit', (event) => {
requiredFields.forEach((input) => {
valid = valid | requireValue(input.input);
});
if (!valid) {
event.preventDefault();
}
});
In the above code, we have written a small validation check to see if the input field is empty. Then we have attached an event listener to the submit button to first process the data before passing it on to the action URL. We make sure that the input field is not empty, and in case it is, we prevent the form submission by calling event.preventDefault()
. In this way, JavaScript and HTML handle the complete form submission.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn