How to Get HTML Form Value in JavaScript
-
Use the
document.forms
to Get HTML Form Value in JavaScript -
Use the
id
to Access HTML Form Value in JavaScript
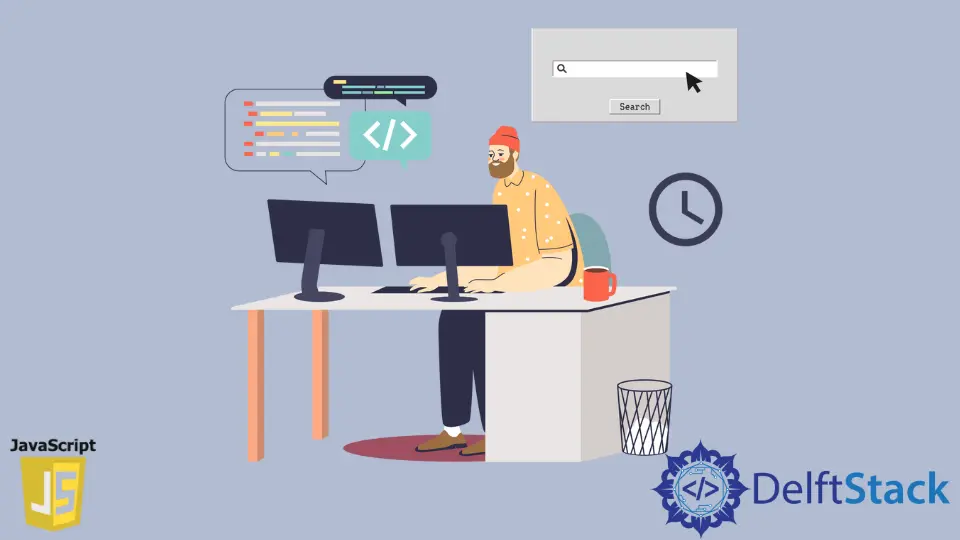
In JavaScript, we can use a specific input element’s id attribute to get the values or trigger the name attribute to access the values.
Both of the conventions will proceed to fetch the raw data from the user. Technically, the target is to grab all the input from a user and ensure the process is working.
Here we will work with the HTML DOM
aka document.forms
to retrieve all the elements from the HTML form
. A brief introduction to the methods available to interact with the HTML elements and attributes is here.
Also, in our example, we will use the casual way of identifying the id
of an element to draw out the information from there.
Use the document.forms
to Get HTML Form Value in JavaScript
The document.forms
method takes the name
attribute to locate the form and its element. Tactically, all values from the form will be passed through this way of initiation.
We will have a select
element followed by a multiple option
element. And we will choose a preference from there, and the value will be separated.
Later, when the document.forms
will be initiated, it will grab the separated value. Let’s check the code block for proper visualization.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<form name="form">
<select name="fruit" id="fruits">
<option value="0">Select Fruit</option>
<option value="mango">Mango</option>
<option value="apple">Apple</option>
<option value="orange">Orange</option>
</select>
<button type = "submit">Hit</button>
</form>
<p id="print"></p>
</body>
</html>
var form = document.forms['form'];
form.onsubmit = function(e) {
e.preventDefault();
var select = document.form.fruit.value;
console.log(select);
document.getElementById('print').innerHTML = select.toUpperCase();
}
Output:
As you can see, the form
object that takes the document.forms
method to interact with the HTML form
values will return all the elements.
The onsubmit
method goes for the further action of working with that selected value. In the instance, the select
object takes the desired value with the corresponding name
attribute of the form
and select
element.
Later we have printed the value in the uppercase format on the page.
Use the id
to Access HTML Form Value in JavaScript
For this example, we will use the documnet.getElementById
the querySelector
to drive on the form inputs.
The facility to use this format is prevalent and works similar to the document.forms
method. So, the following code lines will demonstrate the preview.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<form name="form" id="forms">
Enter name:
<input type="text" id="name">
<input type="button" value=" Click Up">
</form>
<p id="print"></p>
</body>
</html>
var form = document.getElementById('forms');
form.onclick = function(e) {
e.preventDefault();
var name = document.getElementById('name').value;
console.log(name);
document.getElementById('print').innerHTML = name.toUpperCase();
}
Output:
The form accessed by the object form
in JavaScript, and the input’s value was also derived from the .value
method.
In the previous example, we used the button
element to submit the input, and here we used the input type button
to perform the submission.
The difference here is only in the onsubmit
and onclick
, both work similarly.