Form Action Attribute in JavaScript
-
Form
action
Attribute in JavaScript - Another Example Using Form action JavaScript
- Alternative Way to Use Form Action Attribute in JavaScript
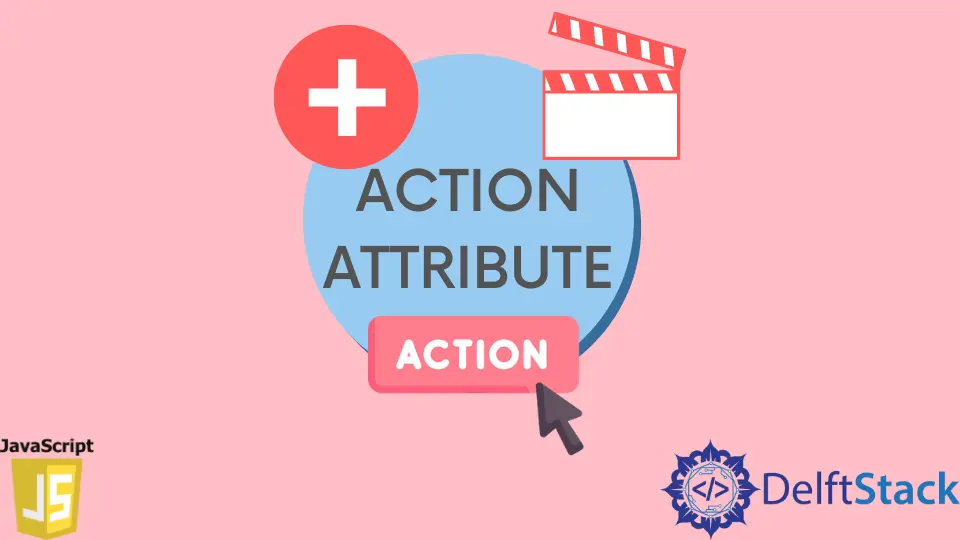
This article explains JavaScript’s form action
attribute. It accesses the form, gets values of all fields, validates form data, and sends it to the right destination. Let’s look at what these action attributes are and how they work.
We can create a form in this way.
<form action="/signup" method="post" id="signup">
</form>
Form action
Attribute in JavaScript
The action
attribute specifies where to send the form data when submitted.
Syntax:
<form action="URL">
action
Attribute Values
- Absolute URL - it points to another website (like
action="https://www.delftstack.com/tutorial/javascript"
) - Relative URL - it points to a file within a website (like
action="example.htm"
)
Form action
Attribute Example Using HTML
Send the form data to a given link to process the input on submitting.
<form action="https://www.delftstack.com" method="get">
<label for="firstname">First name:</label>
<input type="text" id="firstname" name="firstname"><br><br>
<label for="lastname">Last name:</label>
<input type="text" id="lastname" name="lastname"><br><br>
<input type="submit" value="Submit">
</form>
Another Example Using Form action JavaScript
The following example includes an HTML from using a form action
attribute.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Form action javascript</title>
<style>
form label
{
display: inline-block;
width: 100px;
}
form div
{
margin-bottom: 10px;
}
</style>
</head>
<body>
<div class="p-2">
<form id="myForm">
<div>
<label>Name:</label>
<input id="name" name="name" type="text">
</div>
<div>
<label>Email:</label>
<input id="email" name="email" type="email">
</div>
<div>
<input id="submit" type="submit">
</div>
</form>
</div>
</body>
<script>
// setting action on window onload event
window.onload = function () {
setAction('action.php');
};
// this event is used to get form action as an alert which is set by setAction function by using getAction function
document.getElementById('submit').addEventListener('click', function (e) {
e.preventDefault();
getAction();
});
// this function is used to set form action
function setAction(action) {
document.getElementById('myForm').action = action;
return false;
}
// this function is used to get form action assigned by setAction function in an alert
function getAction() {
var action = document.getElementById('myForm').action ;
alert(action);
}
</script>
We have added custom form action
using JavaScript in the code above.
Alternative Way to Use Form Action Attribute in JavaScript
The same results are achievable using the onsubmit
event when a user submits the form.
Syntax:
<element onsubmit="myScript">
Example:
<form onsubmit="myFunction()">
<div>
<label>Name:</label>
<input id="name" name="name" type="text">
</div>
<div>
<label>Email:</label>
<input id="email" name="email" type="email">
</div>
<div>
<input id="submit" type="submit">
</div>
</form>
In the example above, the onsubmit
event is used to submit form data instead of the form action
attribute.